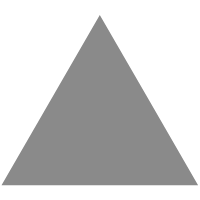
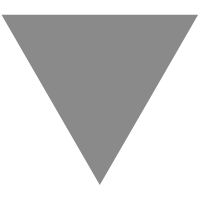
React VS StencilJS Analysis
source link: https://shahruk.com/posts/2022-03-24-React-Stencil-Analysis/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React VS StencilJS Analysis
React VS StencilJS Analysis
24 Mar 2022frontendjavascript
For comparison, I took some performance measurements comparing React vs Stencil. I created a simple webpage with a list where a child element would be appended on every button click. The results of this simple test are here:
View Results in New Window
Source Code
Of all the measurements, the ScriptDuration metric was the most jarring. React's VDOM performance simply could not come close to the performance of StencilJS web components. The JSHeap measurements are also quite stunning, but not surprising for anyone who's ever used a heavy React app. I'm looking forward to taking a deeper look at performance between the two frontend tools in the near future, but for now I'll abstain from any further interpretation of the results.
Most people's introduction to the world of frontend these days is React. React has also overtaken jQuery (the longtime defacto standard library) so it felt a good time to take a look at what React brings to the table.
I've been using Stencil + Ionic framework for a few years now and have fallen in love with the simplicity and concept of how Stencil works. I've also used Angular since Angular 2 and remember the nightmare of Angular modules. Between Angular and React it's clear to see why React won out -- the language is declarative, simple, and expressive. It solves one of the major grievances of modern development: take this element and reference it in this javascript variable. To anyone who's used XCode to build iOS apps before, the simplicity of IBOutlet and click and drop variable references is sorely missed in the world of Javascript, but React brings it close(r).
However beyond the JSX syntax and react starter kit, does React bring much else to the table? React was built internally at Facebook to solve it's own challenges and was released publically in 2013 before web components were drafted in 2014. React uses a virtual DOM instead of the native DOM, most likely due to the challenges it experienced when first writing the library. However ever since then, browsers have only integrated further optimizations into lower level hardware and sticking to Virtual DOM means trusting Facebook's proprietary software diffing algorithms. It's why you see confusing changes from the React team such as the new hooks and inability to edit array values without using a destructured response variable, these are just workarounds to the limitations of working with a VDOM where the actual DOM tree cannot be directly referenced.
These confusing decisions are necessary because the internal React render engine is a closed box. Therefore they have decided to pass custom functions to the developer to make their changes that then get processed internally. Let's compare that to StencilJS which uses native web components. With Stencil generated web components you can style using native attributes like class instead of workarounds like className. There are more benefits, like web component encapsulation and being able to access exposed variables through the DOM element instead of the global scope or object reference.
Compare adding child elements onClick in Stencil:
@State() childElements: string[] = [];
onClick() {
for(var i = 0; i < 1000; i++) {
this.childElements = [
...this.childElements,
'<second-component></second-component>'
];
}
}
To the same exact change in React, where we have to use Suspense and manually assign keys to each child element (and because of this architectural decision, there are modern day challenges with key consistency between server side rendering and client side rendering, which are being addressed in React 18 and beyond).
let [childElements, setElements] = useState([]);
function onClick() {
for(var i = 0; i < 1000; i++) {
childElements = [
...childElements,
<SecondComponent></SecondComponent>
];
setElements(childElements);
}
}
Now compare the actual JSX markup to render the two. Let's take a look at Stencil:
<ul>
{ this.childElements.map((element) => {
return <li innerHTML={ element }></li>
})}
</ul>
And now the same expression in React:
<ul>
<Suspense fallback={<div>Loading...</div>}>
{ childElements.map((element, index) => {
return <li key={index}>{element}</li>
})}
</Suspense>
</ul>
Furthermore, React is a memory hog when compared to Stencil. Stencil uses a compiler to tree shake and implement asynchronous rendering whereas React is a frontend library with a Virtual DOM.
In 2022 I see no reason to use React. After building the following sample To Do app between Stencil and React, I would choose Stencil every time. Unless I'm missing a key benefit of the architectural decisions React has chosen, React's approach seems like a good first approach to the challenges of 2013 that have better solutions in 2022.
- Previous: Using Github Actions for Deployments
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK