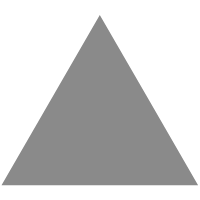
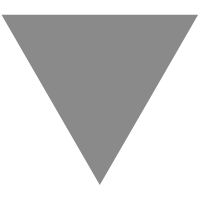
How to replace all occurrences of a string in JavaScript
source link: https://www.laravelcode.com/post/how-to-replace-all-occurrences-of-a-string-in-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to replace all occurrences of a string in JavaScript
Use the JavaScript replace()
method
You can use the JavaScript replace()
method in combination with the regular expression to find and replace all occurrences of a word or substring inside any string.
Let's check out an example to understand how this method basically works:
<script>
var myStr = 'freedom is not worth having if it does not include the freedom to make mistakes.';
var newStr = myStr.replace(/freedom/g, "liberty");
// Printing the modified string
document.write(newStr);
</script>
However, if the string comes from unknown/untrusted sources like user inputs, you must escape it before passing it to the regular expression. The following example will show you the better way to find and replace a string with another string in JavaScript.
<script>
/* Define function for escaping user input to be treated as
a literal string within a regular expression */
function escapeRegExp(string){
return string.replace(/[.*+?^${}()|[\]\\]/g, "\\$&");
}
/* Define functin to find and replace specified term with replacement string */
function replaceAll(str, term, replacement) {
return str.replace(new RegExp(escapeRegExp(term), 'g'), replacement);
}
/* Testing our replaceAll() function */
var myStr = 'if the facts do not fit the theory, change the facts.';
var newStr = replaceAll(myStr, 'facts', 'statistics')
// Printing the modified string
document.write(newStr);
</script>

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK