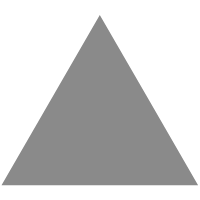
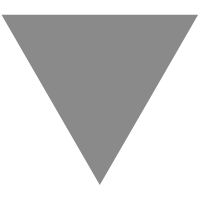
18 个 JavaScript 入门技巧!
source link: https://segmentfault.com/a/1190000040995504
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
作者:Mehul Lakhanpal
译者:前端小智
来源:dev
有梦想,有干货,微信搜索 【大迁世界】 关注这个在凌晨还在刷碗的刷碗智。
本文 GitHub https://github.com/qq449245884/xiaozhi 已收录,有一线大厂面试完整考点、资料以及我的系列文章。
1. 转字符串
const input = 123; console.log(input + ''); // '123' console.log(String(input)); // '123' console.log(input.toString()); // '123'
2. 转数字
const input = '123'; console.log(+input); // 123 console.log(Number(input)); // 123 console.log(parseInt(input)); // 123
3.转布尔值
const input = 1; // 方案1 -使用双感叹号(!!)转换为布尔值 console.log(!!input); // true // 方案2 - 使用 Boolean() 方法 console.log(Boolean(input)); // true
4.字符串'false'
有问题
const value = 'false'; console.log(Boolean(value)); // true console.log(!!value); // true // 最好的检查方法 console.log(value === 'false');
- null vs undefined
null
是一个值,而undefined
不是一个值。null
就像一个空盒子,而undefined
没有盒子。
const fn = (x = '默认值') => console.log(x); fn(undefined); // 默认值 fn(); // 默认值 fn(null); // null
如果传递null,则不采用默认值,而传递undefined
或不传递任何参数时,采用默认值。
6. 真值和虚值
虚值:false
,0
, ""
,null
,undefined
和NaN
。
真值:"Values"
,0"
,{}
,[]
。
7. const 声明变量哪些类型可以被更改
如果值不想被改变时,可以使用 const
:
const name = '前端小智'; name = '王大冶'; // 报错 const list = []; list = [1]; // 报错 const obj = {}; obj = { name: '前端小智' }; // 报错
但用 const 声明的引用类型,它里面值是可以被更改的:
const list = []; list.push(1); // 可以工作 list[0] = 2; // 可以工作 const obj = {}; obj['name'] = '前端小智'; // 可以工作
8. 三等号和双等号的区别
// 双等号 - 将两个操作数转换为相同类型,再比较 console.log(0 == 'o'); // true // 三等号 - 不转换为相同类型 console.log(0 === '0'); // false
9. 接收参数更好的方式
function downloadData(url, resourceId, searchTest, pageNo, limit) {} downloadData(...); // need to remember the order
更简单的方法
function downloadData( { url, resourceId, searchTest, pageNo, limit } = {} ) {} downloadData( { resourceId: 2, url: "/posts", searchText: "WebDev" } );
10.把普通函数改成箭头函数
const func = function() { console.log('a'); return 5; }; func();
可以改写成
const func = () => (console.log('a'), 5); func();
11.从箭头函数返回对象/表达式
const getState = (name) => ({name, message: 'Hi'});
12. 将 set
转换为数组
const set = new Set([1, 2, 1, 4, 5, 6, 7, 1, 2, 4]); console.log(set); // Set(6) {1, 2, 4, 5, 6, 7} set.map((num) => num * num); // TypeError: set.map is not a function
转换为数组
const arr = [...set]
13.检查值是否为数组
const arr = [1, 2, 3]; console.log(typeof arr); // object console.log(Array.isArray(arr)); // true
14. 获取对象的所有键
cosnt obj = { name: "前端小智", age: 16, address: "厦门", profession: "前端开发", }; console.log(Object.keys(obj)); // name, age, address, profession
15. 双问号语法
const height = 0; console.log(height || 100); // 100 console.log(height ?? 100); // 0
这个 ??
的意思是,如果 ??
左边的值是 null
或者 undefined
,那么就返回右边的值。
16. map()
map()
方法创建一个新数组,其结果是该数组中的每个元素是调用一次提供的函数后的返回值。
const numList = [1, 2, 3]; const square = (num) => { return num * num } const squares = numList.map(square); console.log(squares); // [1, 4, 9]
17. try..catch..finally
const getData = async () => { try { setLoading(true); const response = await fetch( "https://jsonplaceholder.typicode.com/posts" ); const data = await response.json(); setData(data); } catch (error) { console.log(error); setToastMessage(error); } finally { setLoading(false); // 不管是否报错,最后都会执行 } }; getData();
18. 解构
const response = { msg: "success", tags: ["programming", "javascript", "computer"], body: { count: 5 }, }; const { body: { count, unknownProperty = 'test' }, } = response; console.log(count, unknownProperty); // 5 'test'
代码部署后可能存在的BUG没法实时知道,事后为了解决这些BUG,花了大量的时间进行log 调试,这边顺便给大家推荐一个好用的BUG监控工具 Fundebug。
原文:https://dev.to/318097/18-tips...
有梦想,有干货,微信搜索 【大迁世界】 关注这个在凌晨还在刷碗的刷碗智。
本文 GitHub https://github.com/qq44924588... 已收录,有一线大厂面试完整考点、资料以及我的系列文章。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK