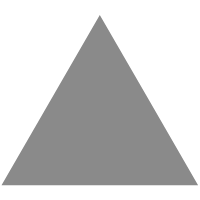
12
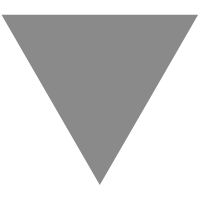
How to Reset a Form Using jQuery or JavaScript
source link: https://www.laravelcode.com/post/how-to-reset-a-form-using-jquery-or-javascript
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Ad byValueimpression
Use the reset()
Method
You can simply use the JavaScript reset()
method to reset a web form.
Let's take a look at the
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Reset Form Using jQuery</title>
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<script>
$(document).ready(function(){
$(".reset-btn").click(function(){
$("#myForm").trigger("reset");
});
});
</script>
</head>
<body>
<form action="/examples/html/action.php" method="post" id="myForm">
<label>First Name:</label>
<input type="text" name="first-name">
<input type="submit" value="Submit">
</form>
<br>
<button type="button" class="reset-btn">Custom Reset Button</button>
</body>
</html>
following example to understand how it basically works:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Reset Form Using JavaScript</title>
<script>
function resetForm() {
document.getElementById("myForm").reset();
}
</script>
</head>
<body>
<form action="/examples/html/action.php" method="post" id="myForm">
<label>First Name:</label>
<input type="text" name="first-name">
<input type="submit" value="Submit">
</form>
<br>
<button type="button" onclick="resetForm();">Custom Reset Button</button>
</body>
</html>
In jQuery there is no method like reset()
, however you can use the jQuery trigger()
method to trigger the JavaScript native reset()
method, like this:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK