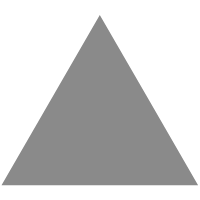
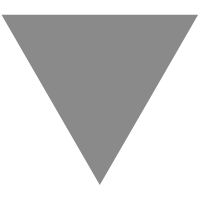
AWS AI Image Recognition Using Serverless on NodeJS
source link: https://dzone.com/articles/aws-ai-image-recognition-using-serverless-on-nodej
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Introduction
In this post, we are going to see how we can identify images using AWS image recognition service called AWS Rekognition on NodeJS, at the end of the guide you will have a system in which you will upload a file to S3, and identification details of that image will be sent to your email address.
Project Setup
We will need to set up a basic serverless project with a serverless.yml file and our lambda function, we also need to install aws-sdk to interact with AWS services,
Serverless.yml File
service: aws-img-rekognition
provider:
name: aws
runtime: nodejs12.x
iamRoleStatements:
- Effect: Allow
Action:
- rekognition:DetectLabels
- s3:Get*
- s3:List*
- sns:Publish*
Resource: "*"
functions:
identifyImg:
handler: src/index.identifyImg
events:
- s3:
bucket: "dev-img-folder"
event: s3:ObjectCreated:*
existing: true
Explanation
Let’s break down what is going on in this serverless.yml file
Permissions – We are defining permissions to allow our lambda function to use AWS rekognition to detect the image, s3 read permissions to read the uploaded image for identification, and lastly permission to publish an SNS event (more on this later).
Lambda functions – We only need a single lambda function for this project, we are defining it in the functions
block.
Events – Final thing is to attach an S3 event to our lambda function so it gets called as soon as a new image is uploaded to the S3 bucket, this bucket
is the name of the bucket where we will upload images, we can create this bucket manually from the AWS console and then put the same name here, the existing
property is set to true
because this bucket is already created, if we don’t pass this flag this template will try to create the bucket.
SNS Topic and Subscription
We are publishing AWS image identification data to an SNS topic, to do this we will need ARN for the SNS topic, an email will be subscribed to this topic, so we will receive all the image related details on the email itself, it is very simple to create an SNS topic and subscription, there is an official AWS documentation for this
Lambda Function
const AWS = require("aws-sdk");
const rekognition = new AWS.Rekognition();
const sns = new AWS.SNS();
exports.identifyImg = async (event) => {
const bucket = event.Records[0].s3.bucket.name;
const key = decodeURIComponent(event.Records[0].s3.object.key.replace(/\+/g, " "));
const params = {
Image: {
S3Object: {
Bucket: bucket,
Name: key,
},
},
MaxLabels: 123,
MinConfidence: 80,
};
try {
const rekognitionData = await rekognition.detectLabels(params).promise();
const snsParams = {
Message: `This image identifies with these - ${rekognitionData.Labels.map((el) => el.Name).toString()}`,
Subject: "Image details",
TopicArn: "arn:aws:sns:us-east-2:175749735948:img-Topic",
};
await sns.publish(snsParams).promise();
} catch (e) {
console.log(e);
}
};
Explanation
Let’s break down this lambda function.
The first step is to get the bucket name and object key which is uploaded to the S3, then we are passing those to the params
object which is the configuration that AWS rekognition accepts.
MaxLabels – This is just to define the maximum number of identifications we want AWS rekognition to return.
MinConfidence – This is the minimum confidence level for the labels to return, confidence here means that how closely should the match be with the label which is returned.
Now we call detectLabels
API of AWS rekognition which will get us the identification data, after we get this data we are going publish this data to our SNS topic.
Message – This is just the configuration key where we pass the message to send to our topic, here we are just mapping the Labels
array by label name and converting that array to string.
TopicArn – This is the ARN of the topic we created above.
Conclusion
If you reach up to this step then congratulations, we now have a system where we can upload an image to the S3 bucket, and then we will receive the image identification data to an email address specified in the subscription created using AWS console and that is how we use AWS image recognition using serverless framework.
You can find the source code on Github
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK