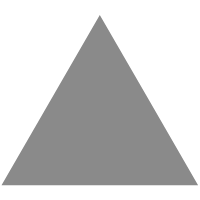
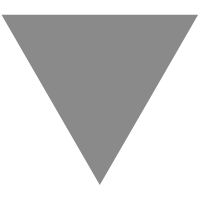
Is there a way to have raw-types in (modern) C++?
source link: https://dev.to/baenencalin/is-there-a-way-to-have-raw-types-in-modern-c-2knd
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Posted on Oct 24
Is there a way to have raw-types in (modern) C++?
What are "raw" types?
Raw types(in Java) is a type who has type-arguments (generics/templates*), but whose type-arguments are not specified in a declaration.
Consider the following (Java) code:
class NeverRaw1 {
NeverRaw1(int i) { this.value = i; }
int value;
}
class PossiblyRaw<T> {
PossiblyRaw(T v) { this.value = v; }
T value;
}
class NeverRaw2 extends PossiblyRaw<int> {
NeverRaw2(int i) { this.value = i; }
}
// ... Some code later...
// This isn't raw because a type argument is specified.
PossiblyRaw<int> notRaw = new PossiblyRaw<>(10);
// This IS raw because none are specified
PossiblyRaw uncooked = new PossiblyRaw<int>(10);
// Invalid. Mismatched types.
notRaw = new PossiblyRaw<float>(10.56);
// Valid. No type mismatch because none is specified.
uncooked = new PossiblyRaw<float>(10.56);
Why would you want types?
So, the first thought that probably popped into your head is "Hey. Isn't that not type-safe?", and to that, I say "Yes. It can be unsafe at times. But then it can also be utilized.".
So? Where can I use this?
Well, here's where I'm stuck. - I'm implementing a Token
type for Janky, and I want to have a parse(std::string|char*)
function that returns an array of Token
.
How is this a problem?
It's a problem because even if you want to return an array of something, you must define the template arguments.
My Token
type is written as such:
template<typename T, T v> struct Token {
T value = v;
TokenType type = TokenType::_UNASSIGNED;
unsigned short col = 0;
unsigned short ln = 0;
};
And I can't create any abstraction, since all pieces of this structure are important to have. -- And because members aren't preserved when you say a piece of data is of its parent type.
So...
What's the best solution here?
Thanks!
Cheers!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK