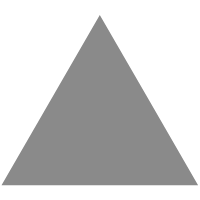
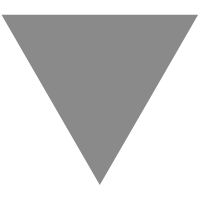
Overload +/- operators to add / subtract vectors
source link: https://www.codesd.com/item/overload-operators-to-add-subtract-vectors.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Overload +/- operators to add / subtract vectors
I am working on a class for a c++ project, and I have to overload all of my operators to work on vectors. Specifically, I define my vector as follows:
template<class Type>
ThreeVector<Type>::ThreeVector(Type x, Type y, Type z) {
mx=x;
my=y;
mz=z;
}
And my +operator as:
template<class Type>
ThreeVector<Type> operator+(const ThreeVector<Type>& v, const//
ThreeVector<Type>& w) {
return ThreeVector<Type>(v)+=w;
}
Where I have already overloaded the += and -= operators. However, I keep getting this error:
ThreeVT.cxx:12:26: error: no matching function for call to//
‘ThreeVector<double>::ThreeVector(ThreeVector<double>)’
ThreeVector<double> d=c+a;
ThreeVector.h:141:29: error: no matching function for call to
‘ThreeVector<double>::ThreeVector(const ThreeVector<double>&)’
return ThreeVector<Type>(v)+=w;
Any help would be much appreciated! This error seems to come up no matter what I do, and I don't know what it really means in this context.
You have several problems:
Functions that take a reference:
ThreeVector( ThreeVector&);
ThreeVector<Type> operator=( ThreeVector&);
ThreeVector<Type> operator+=( ThreeVector&);
ThreeVector<Type> operator-=( ThreeVector&);
ThreeVector<Type> operator-( ThreeVector&);
Should take a const reference, unless they actually change the parameter:
ThreeVector(const ThreeVector&);
ThreeVector<Type> operator=(const ThreeVector&);
ThreeVector<Type> operator+=(const ThreeVector&);
ThreeVector<Type> operator-=(const ThreeVector&);
ThreeVector<Type> operator-(const ThreeVector&);
Your accessor functions are non-const:
Type x();
Type y();
Type z();
But should be const:
Type x() const;
Type y() const;
Type z() const;
All of these changes should be made both in the class body and the function definitions.
Now, on to your operator+
: operator+
can either be a free function or a member function. Either way, you need a left-hand side and a right-hand side. If operator+
is a member function than the lhs is always this
. Thus, for a binary + function your declaration looks like this:
ThreeVector<Type> operator+(const ThreeVector& rhs) const;
Note that the parameter is passed by const
ref, and the function itself is const
, so it can be called on a const ThreeVector
.
The implementation in your code was missing the class name. It should look like:
template<class Type>
ThreeVector<Type> ThreeVector<Type>::operator+(const ThreeVector<Type>& rhs) const
Then, the body of your function can make use of the this
keyword:
return ThreeVector<Type>(*this) += rhs;
A note about operator+=: The standard convention is that operator+=, -=, etc. return a reference to the object that just changed. return *this
. Your function should look like:
ThreeVector<Type>& operator+=(const ThreeVector&);
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK