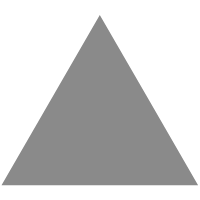
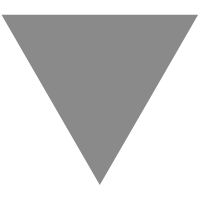
Mocha- A Rich JavaScript Framework.
source link: https://blog.knoldus.com/mocha-a-rich-javascript-framework/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Mocha- A Rich JavaScript Framework.
Reading Time: 4 minutes
When I have started using postman and design my first framework, I have got to know that postman uses Mocha as an internal JavaScript framework to execute and manage the test cases. A few days later I was exploring Cypress.io which also follows Mocha standards for executing and managing test cases. That makes me curious to explore more about Mocha and use its powerful features to make the framework more robust and easy to maintain.
Following is the agenda of this blog:
- Introduction to Mocha
- Mocha Setup
- Mocha Functions
- Mocha Hooks
- Mocha Test Features
- Mocha Retry
- Mocha Timeout
- Mocha Reporters
Introduction to Mocha:
Mocha is a rich JavaScript testing framework running on NodeJs and browsers, just like TestNG and JUnit. it helps to organize and execute the test cases. It is a tool that makes asynchronous testing simple and easy to execute by providing dozen of useful utilities.
Mocha Setup
Just like other JavaScript-based testing frameworks, Mocha also used NodeJs and npm as their execution environment so first ensure NodeJs and other node modules have installed or not, if not, please visit their official website to install. To check that node and other related node modules have installed or not please hit the following commands:
- $ node –version
[It will return the installed version of node] - $ npm –version
[It will returns the installed version of npm module.]
As Mocha also comes as a node module, so to install Mocha send following npm command:
- $ npm install -g mocha
[This command will install the latest stable version of Mocha.]
Mocha Functions:
Mocha comes pre-bundled with two function calls:
- describe(): Describe is a function in the Mocha framework that helps to group the test cases at the test suite level. It is a function that takes two parameters.
- Test Suite Description,
- Callback Function.
describe('Feature Login-Test Suites', function() {
it('TC-T1 | Verify a user can generate login credentials and see error Validations', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TEXTBOX).type(USERNAME);
cy.get(PASSWORD_TEXTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle)
});
it('TC-T2 | Verify an existing user can login into the application and logout', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TESTBOX).type(USERNAME);
cy.get(PASSWORD_TESTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle);
cy.get(USER_PROFILE).click();
cy.get(SIGN_OUT).click();
});
})
- it(): Same as describe() function, ‘it’ is also a function available in the Mocha framework which contains the test case. It also takes two parameters.
- Test Case Description,
- Test code.
it('TC-T1 | Verify a user can generate login credentials and see error Validations', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TEXTBOX).type(USERNAME);
cy.get(PASSWORD_TEXTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle)
});
Note: ‘cy’ is an object in cypress.io which act as an driver just like selenium.
Mocha Hooks:
Mocha supports BDD like interface and comes with four hooks that should be used to set preconditions and clean up the data before and after the tests. These hooks will run as per the defined order. Any hook can be invoked with an optional description, making it easier to pinpoint errors in your tests. If a hook is given a named function, that name will be used if no description is supplied.
describe('hooks', function() {
before(function() {
// runs before all tests in this block
});
after(function() {
// runs after all tests in this block
});
beforeEach(function() {
// runs before each test in this block
});
afterEach(function() {
// runs after each test in this block
});
});
Note: All these hooks should be present in the describe() block if these are not in the describe block it will not execute any of the hooks. It accepts the parameter the same as describe and it functions.
Mocha Test Features:
Mocha provides different test features to ensure which test should be executed and which not to be executed. Mocha provides the following three features:
- Exclusive Tests: The exclusivity feature allows you to run only the specified suite or test-case by appending ‘.only()’ to the function. Previous to v3.0.0, .only() used string matching to decide which tests to execute; this is no longer the case. In v3.0.0 or newer, we can mark multiple tests as exclusive in the suites and tests will be executed as per their precedence.
describe.only('Feature Login-Test Suites', function() {
it.only('TC-T1 | Verify a user can generate login credentials and see error Validations', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TEXTBOX).type(USERNAME);
cy.get(PASSWORD_TEXTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle)
});
it.only('TC-T2 | Verify an existing user can login into the application and logout', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TESTBOX).type(USERNAME);
cy.get(PASSWORD_TESTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle);
cy.get(USER_PROFILE).click();
cy.get(SIGN_OUT).click();
});
})
- Inclusive Tests: This feature is the inverse of .only(). By appending .skip(), we can mark the test as inclusive and tell Mocha to simply ignore the test case(s).
describe.skip('Feature Login-Test Suites', function() {
it.only('TC-T1 | Verify a user can generate login credentials and see error Validations', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TEXTBOX).type(USERNAME);
cy.get(PASSWORD_TEXTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle)
});
it.skip('TC-T2 | Verify an existing user can login into the application and logout', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TESTBOX).type(USERNAME);
cy.get(PASSWORD_TESTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle);
cy.get(USER_PROFILE).click();
cy.get(SIGN_OUT).click();
});
})
- Pending Tests: “Pending”–as in “someone should write these test cases eventually”–test-cases are simply those without a callback, Pending tests will be included in the test results, and marked as pending. A pending test is not considered a failed test. Anything skipped will be marked as pending, and reported as such.
describe('Feature Login-Test Suites', function() {
it('TC-T1 | Verify a user can generate login credentials and see error Validations');
// This will mark as pending
it.skip('TC-T2 | Verify an existing user can login into the application and logout', function() {
cy.visit(BASEURL);
cy.get(USERNAME_TESTBOX).type(USERNAME);
cy.get(PASSWORD_TESTBOX).type(PASSWORD);
cy.get(SIGNIN).click();
cy.title().should('include', pageTitle);
cy.get(USER_PROFILE).click();
cy.get(SIGN_OUT).click();
});
})
Retry In Mocha
- Retry is a function available in mocha to execute the failed test cases several times as per the need.
- Retry function primarily used for end to end testing like selenium web-driver.
- It cannot be used with before() and after() hooks but can be run with the other two hooks.
- It is not recommended to user retry() for unit test cases.
Timeout in Mocha
There are three levels of timeout available in Mocha
- Suite Level Timeout: Suite-level timeouts may be applied to entire test “suites”, or disabled via this.timeout(0). This will be inherited by all nested suites and test-cases that do not override the value.
describe('Feature Login-Test Suites', function() {
this.timeout(500);
it.only('LLCARES-T1 | Verify a user can generate login credentials and see error Validations', function(done) {
// ----------- Tests
});
})
- Test Level Timeout: Test-specific timeouts may also be applied, or the use of this.timeout(0) to disable timeouts all together
describe('Feature Login-Test Suites', function() {
it.only('LLCARES-T1 | Verify a user can generate login credentials and see error Validations', function(done) {
this.timeout(500);
setTimeout(done, 3000);
});
})
- Hook Level Timeout:
describe('Feature Login-Test Suites', function() {
beforeEach(function(done) {
this.timeout(500);
setTimeout(done, 3000);
})
it.only('LLCARES-T1 | Verify a user can generate login credentials and see error Validations', function(done) {
// ---------- Tests
});
})
Mocha Reporters
- Mocha reporters are mostly terminal based, there are different type of reporters available in mocha.
- spec and dot matrix are the commonly used reporters in Mocha.
- $ mocha test/ –reporter [:spec]/[:dot]/[:nyan]/[:json]
I hope you have enjoyed this post, follow up here to see what’s next..
References:
https://mochajs.org/
https://testautomationu.applitools.com/mocha-javascript-tests
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK