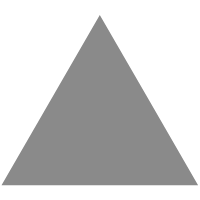
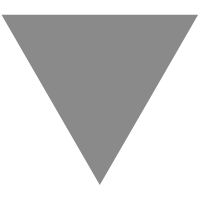
golang中struct、json、map互相转化
source link: https://studygolang.com/articles/35184
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
golang中struct、json、map互相转化
xiaojun1195 · 大约2小时之前 · 61 次点击 · 预计阅读时间 4 分钟 · 大约8小时之前 开始浏览golang中struct、json、map互相转化
小拳头 2018-05-07 23:14:14 85099 收藏 38
订阅专栏
一、Json和struct互换
(1)Json转struct例子:
package main
import (
"fmt"
"encoding/json"
)
type People struct {
Name string `json:"name_title"`
Age int `json:"age_size"`
}
func JsonToStructDemo(){
jsonStr := `
{
"name_title": "jqw"
"age_size":12
}
`
var people People
json.Unmarshal([]byte(jsonStr), &people)
fmt.Println(people)
}
func main(){
JsonToStructDemo()
}
输出:
注意json里面的key和struct里面的key要一致,struct中的key的首字母必须大写,而json中大小写都可以。
(2)struct转json
在结构体中引入tag标签,这样匹配的时候json串对应的字段名需要与tag标签中定义的字段名匹配,当然tag中定义的名称不需要首字母大写,且对应的json串中字段名仍然大小写不敏感。此时,结构体中对应的字段名可以不用和匹配的一致,但是首字母必须大写,只有大写才是可对外提供访问的。
package main
import (
"fmt"
"encoding/json"
)
type People struct {
Name string `json:"name_title"`
Age int `json:"age_size"`
}
func StructToJsonDemo(){
p := People{
Name: "jqw",
Age: 18,
}
jsonBytes, err := json.Marshal(p)
if err != nil {
fmt.Println(err)
}
fmt.Println(string(jsonBytes))
}
func main(){
StructToJsonDemo()
}
输出:
二、json和map互转
(1)json转map例子:
func JsonToMapDemo(){
jsonStr := `
{
"name": "jqw",
"age": 18
}
`
var mapResult map[string]interface{}
err := json.Unmarshal([]byte(jsonStr), &mapResult)
if err != nil {
fmt.Println("JsonToMapDemo err: ", err)
}
fmt.Println(mapResult)
}
输出:
(2)map转Json例子
func MapToJsonDemo1(){
mapInstances := []map[string]interface{}{}
instance_1 := map[string]interface{}{"name": "John", "age": 10}
instance_2 := map[string]interface{}{"name": "Alex", "age": 12}
mapInstances = append(mapInstances, instance_1, instance_2)
jsonStr, err := json.Marshal(mapInstances)
if err != nil {
fmt.Println("MapToJsonDemo err: ", err)
}
fmt.Println(string(jsonStr))
}
输出:
func MapToJsonDemo2(){
b, _ := json.Marshal(map[string]int{"test":1, "try":2})
fmt.Println(string(b))
}
输出:
三、map和struct互转
(1)map转struct
需要安装一个第三方库
在命令行中运行: go get github.com/goinggo/mapstructure
例子:
func MapToStructDemo(){
mapInstance := make(map[string]interface{})
mapInstance["Name"] = "jqw"
mapInstance["Age"] = 18
var people People
err := mapstructure.Decode(mapInstance, &people)
if err != nil {
fmt.Println(err)
}
fmt.Println(people)
}
输出
(2)struct转map例子
func StructToMapDemo(obj interface{}) map[string]interface{}{
obj1 := reflect.TypeOf(obj)
obj2 := reflect.ValueOf(obj)
var data = make(map[string]interface{})
for i := 0; i < obj1.NumField(); i++ {
data[obj1.Field(i).Name] = obj2.Field(i).Interface()
}
return data
}
func TestStructToMap(){
student := Student{10, "jqw", 18}
data := StructToMapDemo(student)
fmt.Println(data)
}
输出:
————————————————
版权声明:本文为CSDN博主「小拳头」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。
原文链接:https://blog.csdn.net/xiaoquantouer/article/details/80233177
有疑问加站长微信联系(非本文作者)

入群交流(和以上内容无关):加入Go大咖交流群,或添加微信:liuxiaoyan-s 备注:入群;或加QQ群:701969077
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK