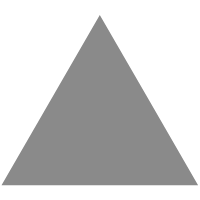
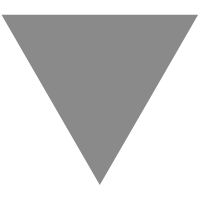
Automate your AWS EC2 Management with Python and Boto 3
source link: https://blog.usejournal.com/aws-ec2-management-with-python-and-boto-3-59d849f1f58f
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Automate your AWS EC2 Management with Python and Boto 3

About this blog
Launching your AWS EC2 instance from AWS Console requires a lot of manual step and configurations. One must follow best practices to keep their instance safe and secure. Often time doing things manually involves repetitive tasks with the scope of having some mistakes. Physical allocation of resources can sometimes put your infrastructure at a higher risk.
Want to read this story later? Save it in Journal.
In this post, we’ll look at how to automate and launch EC2 instance in a particular region with custom VPC, subnet and security group using Python and Boto 3 API.
Requirements
- An Amazon Web Services account for a non-root user.
- Configure Boto 3 library. Check out their official guidelines on how to set up your credentials.
- AWS CLI installation
Steps
1. Initialisation of libraries and code
import boto3
from requests import getclient = boto3.client('ec2', 'us-east-1')
resource = boto3.resource('ec2', 'us-east-1')
2. Setup a VPC
- VPC stands for virtual private cloud. It is a logically isolated dedicated network to create your AWS resources.
- Launch a VPC with 192.168.1.0/24 Classless Inter-Domain Routing (CIDR) in the us-east-1 region.
#Create VPC
vpcInit = client.create_vpc(CidrBlock='192.168.1.0/24')
vpc = resource.Vpc(vpcInit["Vpc"]["VpcId"])
vpc.create_tags(Tags=[{"Key": "Name", "Value": "ec2-vpc"}])
3. Create an internet gateway
- An internet gateway is a VPC component that enables connectivity between your VPC and the internet.
#Create Internet Gateway
igInit = client.create_internet_gateway(TagSpecifications=[
{'ResourceType': 'internet-gateway',
'Tags': [{"Key": "Name", "Value": "ec2-ig"}]},])igId = igInit["InternetGateway"]["InternetGatewayId"]#Attach Internet Gateway to VPC
vpc.attach_internet_gateway(InternetGatewayId=igId)
4. Build a routing table and a public route to an internet gateway
- A route table is a set of rules called routes that decide where network traffic from your subnet or gateway is routed.
#Create Routing Table
routeTable = vpc.create_route_table()#Create public route
route = routeTable.create_route(DestinationCidrBlock='0.0.0.0/0',
GatewayId=igId)
routeTable.create_tags(Tags=[{"Key": "Name", "Value": "ec2-rt"}])
5. Establish a public subnet
- A sub-network, also known as a subnet, is a technical division of an IP network. Subnetting is the method of splitting a network into two or more networks.
- A public subnet is connected to a routing table and has a route to an internet gateway.
#Create Subnet
subnet = vpc.create_subnet(CidrBlock='192.168.1.0/24', AvailabilityZone="{}{}".format('us-east-1', 'a'))
subnet.create_tags(Tags=[{"Key": "Name", "Value": snName}])#Associate subnet with public route table
routeTable.associate_with_subnet(SubnetId=subnet.id)
6. Configure the security group
- To monitor incoming and outgoing traffic, a security group serves as a virtual firewall for your EC2 instances. Inbound rules govern incoming traffic to your instance, while outbound rules govern outgoing traffic from your instance.
- We will ping api.ipify.org to get the public IP of the device and whitelist that IP in the security group.
- For an SSH connection, the ingress rule was defined with a given IP.
ip = get('https://api.ipify.org').text#Create Security Group
secGroup = resource.create_security_group(GroupName='ec2-sg', Description='EC2 Security Group', VpcId=vpc.id)#Ingress Rule
secGroup.authorize_ingress(IpPermissions=[
{'FromPort': 22,
'IpProtocol': 'tcp',
'IpRanges': [{'CidrIp': '{}/0'.format(ip),
'Description': 'SSH Access'
},],
'ToPort': 22,
},
])
7. Generate a PEM file
- PEM is a file format that is commonly used to store cryptographic keys.
- It will be used to authorise and connect an EC2 instance.
keyFileName = "infraSecrets"
#Create key pair
client.create_key_pair(KeyName=keyFileName)#Save generated keys in the pem file
privateKeyFile = open('{}.pem'.format(keyFileName), "w")
privateKeyFile.write(dict(keyPair)['KeyMaterial'])
privateKeyFile.close
8. Launch an EC2 instance
- We will be launching an Amazon Linux 2 AMI machine as a t2.micro instance in the created subnet with the defined security group.
#Launch EC2 Instance
ec2Instances = resource.create_instances(
ImageId = 'ami-048f6ed62451373d9',
InstanceType ='t2.micro', MaxCount = 1, MinCount = 1,
NetworkInterfaces = [{'SubnetId': subnet.id,
'DeviceIndex': 0, 'AssociatePublicIpAddress':
True, 'Groups': [secGroup.group_id]}],
KeyName = keyFileName)
instance = ec2Instances[0]
instance.create_tags(Tags=[{"Key": "Name", "Value": "ec2-infra"}])
instance.wait_until_running()
Final Code
AWS Automation for EC2 InstanceOutput


Summary and What’s Next
In this article, we have automated the process of running an EC2 setup. The code is easy to customise based on the different requirements. In future, We can explore further automation in AWS infrastructures like creating a server-less compute using AWS lambda, dynamo DB and S3. Till then I am open to any of your questions.
I hope that you have enjoyed this post and will help you with the automation of EC2 management. I would love to hear your valuable feedback.
Thank you!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK