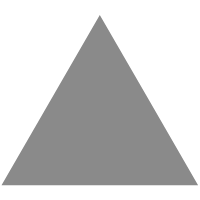
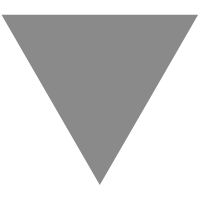
OOP Features of RUST
source link: https://blog.knoldus.com/oop-features-of-rust/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The OOP concept is designed to overcome the drawbacks of procedural programming and structured programming approach. We already know that the four main pillars of OOP (Object Oriented Programming Language) are Encapsulation, Inheritance, Abstraction, and Polymorphism. In this blog, we will discover how rust also provides some of the necessary features of OOP.
1. Encapsulation –
Encapsulation is wrapping of data and information together in a single unit called Class. But since we already know that there is no Class implementation in Rust, so how can we achieve encapsulation in Rust.
In Rust, we achieve this by using structures. Let’s see an example for the same :
pub struct AverageMarks { list: Vec<i32>, average: f64, } impl AverageMarks { pub fn add(&mut self, marks: i32) { self.list.push(marks); self.update_average(); } pub fn remove(&mut self) -> Option<i32> { let result = self.list.pop(); match result { Some(marks) => { self.update_average(); Some(marks) } none => none, } } pub fn average(&self) -> f64 { self.average } fn update_average(&mut self) { let total: i32 = self.list.iter().sum(); self.average = total as f64 / self.list.len() as f64; } } fn main(){ let mut list: Vec<i32> =vec![]; let mut averagemarks =AverageMarks{ list: vec![], average: 0.0 }; averagemarks.add(25); averagemarks.add(16); averagemarks.add(85); println!("average marks of student {}",averagemarks.average()); averagemarks.remove(); println!("average marks of student {}",averagemarks.average()); }
2. Inheritance –
Inheritance is a way of code reusability and type safety. In code reusability, we can implement the behavior of one type and reuse the implementation in different sub-classes, through this, we can share code between two classes. But nowadays most of the programming design do not prefer to use code sharing because more code sharing means more, risk and also sub-classes should not share all the characteristics of the parent class as this makes code less flexible so, Rust does not support Inheritance.
3. Polymorphism
Polymorphism means many forms or we can say that the same function has a different implementation. There are two types of polymorphism: compile time and runtime. Compile time polymorphism is achieved by operator overloading and method overloading in c++ and runtime polymorphism can be achieved by method overriding in c++. Rust takes a different approach to achieve polymorphism by using trait.
pub trait Shape { fn area(&self) -> f32; } pub struct Circle { pub radius: f32, } impl Shape for Circle { fn area(&self) -> f32 { 2 as f32 * 3.14 * self.radius * self.radius } } pub struct Rectangle { pub length: f32, pub width: f32, } impl Shape for Rectangle { fn area(&self) -> f32 { self.length * self.width } } pub struct Square { side: f32, } impl Shape for Square { fn area(&self) -> f32 { self.side * self.side } } fn main() { let circle = Circle { radius: 10.36 }; println!("Area of circle is {}", circle.area()); let rectangle = Rectangle { length: 10.59, width: 20.22 }; println!("Area of Rectangle is {}", rectangle.area()); let square = Square { side: 5.96 }; println!("Area of Square is {}", square.area()); }
4. Abstraction –
Abstraction is the most important feature of OOP, abstraction allows you to show only the necessary information and hide all other details. In rust, we can achieve abstraction by using traits as in traits we only define methods and do not define their implementation.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK