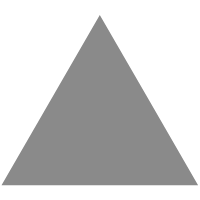
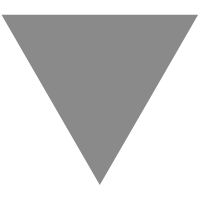
Pattern matching in RUST
source link: https://blog.knoldus.com/pattern-matching-in-rust/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Pattern matching in RUST
Reading Time: 2 minutes
Pattern matching is a mechanism for checking a value against a pattern. A successful match can also deconstruct a value into its constituent parts. It is a more powerful version of the statementswitch
in languages like C++, and Java, it can likewise be used in place of a series of if/else statements.
In Rust, Patterns are a special type of syntax for matching against the structure of types, both complex and simple. Using patterns in conjunction with expressions and match construct gives you more control over a program’s control flow. A pattern consists of a combination of the following:
- Literals
- Destructured arrays, enums, structs, or tuples
- Variables
- Wildcards
- Placeholders
These components describe the shape of the data we’re working with, which we then match against values to determine whether our program has the correct data to continue running a particular piece of code.
- Pattern matching on Literals, variable and wildcards
fn main() {
let x = 1;
match x {
1 => println!("one"),
2 => println!("two"),
3 => println!("three"),
_ => println!("anything"),}
}
2. Pattern matching on Enums and Struct
struct Point {
x: i32,
y: i32,
}
fn main() {
let p = Point { x: 0, y: 7 };
match p {
Point { x, y: 0 } => println!("On the x axis at {}", x),
Point { x: 0, y } => println!("On the y axis at {}", y),
Point { x, y } => println!("On neither axis: ({}, {})", x, y),
}
}
enum Message {
Quit,
Move { x: i32, y: i32 },
Write(String),
ChangeColor(i32, i32, i32),
}
fn main() {
let msg = Message::ChangeColor(0, 160, 255);match msg {
Message::Quit => {
println!("The Quit variant has no data to destructure.")
},
Message::Move { x, y } => {
println!("Move in the x direction {} and in the y direction {}", x,y); }
Message::Write(text) => println!("Text message: {}", text),
Message::ChangeColor(r, g, b) => {
println!("Change the color to red {}, green {}, and blue }", r, g, b )
}
}
}
3.Pattern matching on Multiple Possibilities and Pattern Guard
let num = Some(4);
match num {
Some(x) if x < 5 => println!("less than five: {}",x),
Some(x) => println("{}",x),
None => (),
}
fn main() {
let x = 4;
let y = false;
match x {
4 | 5 | 6 if y => println!("yes"),
_ => println!("no"),
}
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK