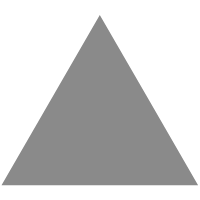
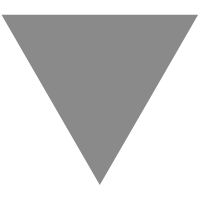
How to Avoid JavaScript Type Conversions | by Viduni Wickramarachchi | Mar, 2021...
source link: https://blog.bitsrc.io/how-to-avoid-javascript-type-conversions-29e1258f37d8
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Avoid JavaScript Type Conversions
JavaScript performs implicit conversions between data types. Let’s find out how to avoid it.

Have you ever experienced a scenario where certain value comparisons in JavaScript do not result in what you expected?
For example, take a look at the condition below.

Even though []==0
results in true
, the if
condition has not executed according to that result. Ever wondered why that is?
This article is all about explaining how these value comparisons work and what affects them. Before diving into that explanation, there is a concept you should be familiar with; Type Conversions.
What are JavaScript Type Conversions?
This is also known as Type Coercion. For those of you who aren’t familiar with this concept, it is simply the automatic conversion of values from one data type to another.
This occurs because JavaScript is a weakly typed language.
Let’s look at an example to make it clearer.

In this example, the two variables defined are of two types; String and Number. However, when we compare those using ==
(Abstract Equality Comparison / Non-strict comparison), it results true
. The reason behind this is when we compare these two using ==
JavaScript will automatically try to convert the String type to Number type to produce the result. It is a forceful type of conversion.
There are many types of coercion in JavaScript.
- Number conversions
- String conversions
- Boolean conversions
- Type conversion for objects, etc.
Here are some examples.

Is type coercion always good?
Well, in a scenario as above, the type conversion does no harm. However, there are many instances where type coercion can cause problems.
Let’s have a look at an example.

Here, JavaScript has converted the Number type to String. This is the opposite of what happened in the equality comparison. The result we expected was 450. However, we got a String output instead.
Therefore, what is our conclusion?
JavaScript type conversions are inconsistent. It should be avoided in Production code!
Now that you have a clear idea about type conversions and why they should be avoided, let’s have a look at how to avoid them. This is the most important part of this article. Therefore, sit back, grab a coffee and concentrate well 😃
Tip: Collaborate on independent components with Bit
Bit is an ultra-extensible tool that lets you author, version, and share independent components.
Use it to build modular design systems, author and deliver micro frontends or simply share components between applications.
Material UI components shared individually on Bit.devHow to avoid JavaScript type conversions
1. Use explicit conversions with mathematical operations
If you need to use a mathematical operation on user input or any other value, it is safer to do an explicit conversion yourself before doing the operation. This way, you can avoid any unexpected behavior. Especially with the +
operator. This is a special kind of operator which is used for additions as well as string concentrations. Therefore, if you need to add, it is safer to convert the values to an integer and float beforehand.

2. Concatenate string using template literals instead of +
If you have two numbers that you need to concatenate, it is safer to use template literals. Especially if you aren’t sure about the type of your values.

You could also use explicit conversions to derive the same result.

3. Use strict comparison (===) when comparing values
Earlier we saw that when ==
is used, JavaScript performs an implicit type conversion which results in inconsistent results. Therefore, this is not safe to use in our production code.
In order to derive the expected results, we should always use ===
for comparisons. This triple equal sign implicitly says,
I care about both the value and type of a variable
Therefore, if a number and a string are compared with the value, it will result in false
as it will be considering the type of the variable as well.

This is the safer approach to derive consistent results as expected.
Up until now, we have explored JS type conversions and how to avoid them in order to eliminate unwanted errors. However, you might still be confused about the first example I provided and why it happened so.
In JavaScript, there are two variants of data types.
- Primitive values (String, Number, etc.)
- Non-primitive values (Arrays, Objects)
We spoke about type conversions of primitive data types so far. The first example I provided involves non-primitive data types such as arrays.
All non-primitive data types have an inbuilt function called .toPrimitive()
. When non-primitive and primitive values are compared, the non-primitive type is automatically converted to a primitive type by this function. In the first example we looked at, the empty array is converted to an empty string when a non-strict comparison is done using this function. To be precise, the exact function used to do this conversion is toString()
. Therefore, the empty array (which is converted to an empty string) is equal to 0.

However, as we saw earlier, when the empty array is checked inside an if
condition, the line inside the condition was executed. But how could that happen if the empty array implicitly converts to 0?
Well, this falls under a separate JavaScript condition; truthy and falsy values. Along with true
, JavaScript considers all values as truthy values, except for few values. For example, 0, -0, "”
are considered as falsy values. You can find all the falsy values here. Since an empty array is not considered as a falsy value, when it is checked for in a condition, it will be executed as true
. (No type-conversion will happen here, the empty array is kept as an array — Another example for type conversion inconsistencies.)

Conclusion
JavaScript being a loosely typed language performs implicit type conversions. This will result in inconsistencies and unexpected results. Therefore, we should avoid such type conversions at all times. If you are unsure about the type of value, it can be checked using typeof
. Checking for the type will give you a better understanding of how you should do a conversion.
Thanks for reading!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK