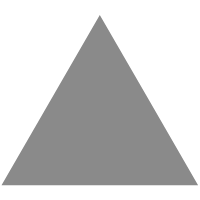
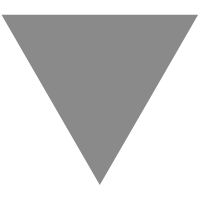
How to use Tailwind CSS in Laravel
source link: https://justlaravel.com/how-to-use-tailwind-css-laravel/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to use Tailwind CSS in Laravel
Hello everybody, welcome to justlaravel.com, I am back with another tutorial. Here am going to tell you how to use Tailwind CSS in Laravel applications. I will set up Tailwind and also create a dummy app in Laravel making use of this Tailwind CSS.
Let’s get started!
You can also watch the video on YouTube here.
To use Tailwind CSS and its utility first components based approach in our Laravel applications, first, we need to create a new Laravel Project.
I will create a new Laravel app using laravel new
command.
laravel new tailwindcss-laravel
After that go to the root folder and run composer install
, so it installs all required packages needed for a Laravel app.
Next, I will navigate to the application’s root and run the project on a local server using php artisan serve
php artisan serve
You should see Laravel’s default page, which means the project is successfully set up.
Let us get into Tailwind CSS now.
Install Tailwind CSS:
Tailwind CSS can be installed in a few ways,
Using npm
This is the best possible option to install Tailwind CSS for any Laravel project. To install a simple npm install command is to run.
npm install tailwindcss
So when the above command is run, the Tailwind CSS will be installed in your project.
Using Yarn
Yarn is also very similar to npm, it can be replaced with npm if the project uses Yarn as a package manager. The below command adds Tailwind CSS to a Laravel app.
yarn add tailwindcss
Using a CDN
This is the least preferred option. To make full use of Tailwind, it must be incorporated into the build process. Anyways if you still prefer to use it as a CDN, you can get if from the link below.
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
Tailwind CSS into Laravel App
So am going to use npm in this Laravel Project, I will navigate to the root of my application and will run the following command in my terminal.
npm install tailwindcss
Import Tailwind CSS to Laravel
Now that Tailwind CSS is added to node_modules we are ready to use and import it into our Laravel, which I just created.
Navigate to app.css
under resources/CSS path and replace the content with the following content.
Am using Laravel 8, it doesn’t have anything inbuilt, but the previous versions have Bootstrap inbuilt which is imported from node_modules and also a preprocessor like SCSS is used. Since Laravel version 8, everything is clean and users are open to add anything they wish to use in their Laravel apps.
/resources/css/app.css
@tailwind base; @tailwind components; @tailwind utilities;
The above directives will inject Tailwind’s base, components, and utilities into our app.
Generate config file for Tailwind CSS
Up to this point, I have installed tailwind and imported it into our applications CSS. Now its time to generate the config file.
The config file is used by Laravel Mix when we build our CSS from the resources folder to the public folder’s app.css
.
There is a command which when run on a terminal or command prompt, will generate us the required config file.
So in your application’s root run the following command.
npx tailwind init
Now if you check your app’s root, you will notice a new file generated named tailwind.config.js
. Open it in your favorite text editor or an IDE and you should see the following code in it.
module.exports = { purge: [], theme: { extend: {}, }, variants: {}, plugins: [], }
This is the default config file and you are free to make any changes required in this file. You can find more settings on this at Tailwind’s main docs — https://tailwindcss.com/docs/
Add Tailwind to Laravel Mix
By now, almost everything is set, I have installed, imported, and also generated the tailwind’s config file. So its time to tell Laravel to use this setup we have done so far.
In Laravel’s mix that is in webpack.mix.js
which is located in the application’s root, I need to tell Laravel to use Tailwind CSS.
Open the file(webpack.mix.js
) and replace it with the following code.
const mix = require('laravel-mix'); const tailwindcss = require('tailwindcss'); mix.js('resources/js/app.js', 'public/js') .postCss('resources/css/app.css', 'public/css', [ require('tailwindcss'), ]);
Note that here am using plain CSS and not any preprocessors, if you are using any preprocessor, then there might be few changes in the above code, please refer to the docs here — https://tailwindcss.com/docs/
Build Laravel assets(Laravel Mix)
Now everything is ready, We just need to compile and run these together. As we have used npm in our project we can simply run npm run dev
or npm run watch
. When I run npm run dev
, every time I make a change I should re-run the command whereas if I run npm run watch
, it will automatically pick up any changes made. So I prefer running it with a watch command.
Before we run any of the two, first I need to run npm install
for all the required node_modules to setup.
npm install
npm run watch
Sample Application
Boom! All done. Everything is set up, installed, and working perfectly. Now let’s do a sample application and use the Tailwind CSS components.
I will call an API, get the data, and display them using Tailwind’s components.
Connect to the API
Here I will connect to my own website’s REST API, as this is in WordPress, by default WordPress offers an API to access and use its data. So I am connecting to my own website.
For any WordPress site, you can add /wp-json/wp/v2/posts
at the end of the URL to get the posts data.
So API endpoint is https://justlaravel.com/wp-json/wp/v2/posts, when that URL is called it will return use some JSON data which looks as in the image below.
Tailwind CSS into Laravel AppGet data from the API
So, I figured out the API endpoint, now I need to get that data into our Laravel app.
Firstly, I will create a controller and create a route to call that controller’s method which will connect to the API and get us the data.
Create a controller,
php artisan make controller:MainController
Define a route, so a major change in Laravel 8 is the normal routing which we have done in all previous versions might not work by default.
Laravel changed the way routing works.
The old way which won’t work,
Route::get('/getData', 'MainController@getData');
The new way, which works.
use App\Http\Controllers\MainController; Route::get('/getData', [MainController::class, 'getData']);
So, make sure you follow the new way while routing in all your future Laravel projects.
Now, in the MainController
, getData()
method, I will call the API using Laravel’s HTTP
method.
// Call the API $response = Http::get('https://justlaravel.com/wp-json/wp/v2/posts');
After calling the API, I need to get the data, and it is available in body()
method of $response
.
// Get the data $data = json_decode($response->body());
and finally, return the data to the view. Here I will use the default welcome.blade.php
.
Complete MainController
will now look like,
<?php namespace App\Http\Controllers; use Illuminate\Support\Facades\Http; class MainController extends Controller { public function getData(){ $response = Http::get('https://justlaravel.com/wp-json/wp/v2/posts'); $data = json_decode($response->body()); return view('welcome')->withData($data); } }
Display the data using Tailwind’s utilities
I have connected to the API, passed the data to the view. So now its time to show the data.
Here am going to use Tailwind CSS features and make a good-looking view without even writing a single line of CSS.
There are many utilities to use, you can find all of them at https://tailwindcss.com/docs.
From the API, I will fetch the title of the post, the featured image, the excerpt, the link of the post.
I will loop the $data
variable sent to the view.
@foreach($data as $post)
We can now find the data required.
Title — {!! $post->title->rendered !!}
Excerpt — {!! $post->excerpt->rendered !!}
Link — {{ $post->link }}
Featured Image — {{ $post->jetpack_featured_media_url }}
After using the utilities and the above variables, the blade file will look like the below snippet.
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Laravel - TailWindCSS</title> <link href="{{ asset('css/app.css') }}" rel="stylesheet"> </head> <body> <h1 class="m-8 text-3xl text-gray-900 leading-tight"> Just Laravel Posts </h1> <div class="px-2"> <div class="flex flex flex-wrap -mx-2"> @foreach($data as $post) <div class="w-1/3 px-2"> <img class="" src="{{ $post->jetpack_featured_media_url }}" alt="Sunset in the mountains"> <div class="px-6 py-4"> <div class="font-bold text-xl mb-2">{!! $post->title->rendered !!}</div> <p class="text-gray-700 text-base"> {!! $post->excerpt->rendered !!} </p> </div> <div class="px-6 pt-4 pb-2"> <span class="inline-block bg-blue-200 rounded-full px-3 py-1 text-sm font-semibold text-gray-700 mr-2 mb-2"><a href="{{ $post->link }}" target="_blank">Click Here</a></span> </div> </div> @endforeach </div> </div> </body> </html>
The output is as below.

That’s guys. You have finally made it to the end of this detailed tutorial on integrating Tailwind CSS into a Laravel Application.
Feel free to look at the other posts on vue js or watch Just Laravel’s videos on YouTube.
- Videos
- The Image
- Builds
- Youtube Videos
- Sources
- Anyways
- Be Incorporated
- Bridal Sets
- Class
- Connected
- Videos
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK