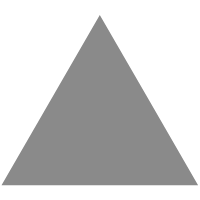
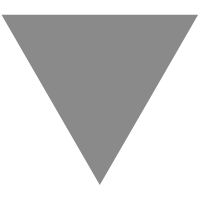
从问题入手,深入了解JavaScript中原型与原型链
source link: http://www.cnblogs.com/liujingjiu/p/14380700.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
从问题入手,深入了解JavaScript中原型与原型链
前言
开篇之前,我想提出3个问题:
- 新建一个不添加任何属性的对象为何能调用toString方法?
- 如何让拥有相同构造函数的不同对象都具备相同的行为?
- instanceof关键字判断对象类型的依据是什么?
要是这3个问题都能回答上来,那么接下来的内容不看也罢。但若是对这些问题还存在疑虑和不解,相信我,下面的内容将正是你所需要的。
正文
新建一个不添加任何属性的对象为何能调用toString方法?
我在 深入了解JavaScript中基于原型(prototype)的继承机制 一文中提到过,JavaScript使用的是 基于原型的继承机制 ,它的 引用类型与其对应的值 将都存在着__proto__属性, 指向继承的原型对象 。当访问对象属性无果时,便会在其原型对象中继续查找,倘若其原型对象中还是查询无果,那便接着去其原型对象的原型中去查找,直到查找成功或原型为null时才会停止查找。
let obj = { } obj.toString();//"[object Object]"
这段代码就是在obj对象中查找toString方法,查询无果,继而在其原型中查找toString方法,正好其原型中含有toString方法,故而得以输出"[object Object]"。
如何让拥有相同构造函数的不同对象都具备相同的行为?
下面是一段实现了 发布订阅模式 的代码:
let _indexOf = Array.prototype.indexOf; let _push = Array.prototype.push; let _slice = Array.prototype.slice; let _concat = Array.prototype.concat; let _forEach = Array.prototype.forEach; function Publish(){ this.subList; this.indexOf = function(sub){ let index = -1; if(typeof this.subList === 'undefined' || this.subList === null){ this.subList = []; } if(typeof sub !== 'undefined' && sub !== null){ index = _indexOf.call(this.subList,sub); } return index; } this.addSub = function(sub){ let index = this.indexOf(sub); index > -1 ? '' : _push.call(this.subList,sub); }; this.removeSub = function(sub){ let index = this.indexOf(sub); index > -1 ? index === 0 ? this.subList = _slice.call(this.subList,1) : this.subList = _concat.call(_slice.call(this.subList,0,index),_slice.call(this.subList,index + 1)) : ''; }; this.notifySingle = function(sub,msg){ let index = this.indexOf(sub); index > -1 ? (typeof sub.onReceive === 'function' ? sub.onReceive(msg) : '') : ''; }; this.notifyAll = function(msg){ if(typeof this.subList !== 'undefined' && this.subList !== null){ _forEach.call(this.subList,(sub)=>{ if(typeof sub !== 'undefined' && sub !== null){ typeof sub.onReceive === 'function' ? sub.onReceive(msg) : ''; } }) } }; } function Subscription(name){ this.name = name; this.onReceive = function(msg){ console.log(this.name + ' 收到消息 : ' + msg); }; } let pub = new Publish(); let sub1 = new Subscription('sub1'); let sub2 = new Subscription('sub2'); let sub3 = new Subscription('sub3'); let sub4 = new Subscription('sub4'); pub.addSub(sub1); pub.addSub(sub1); pub.addSub(sub2); pub.addSub(sub3); pub.addSub(sub4); pub.notifyAll('这是一条全部推送的消息'); // sub1 收到消息 : 这是一条全部推送的消息 // sub2 收到消息 : 这是一条全部推送的消息 // sub3 收到消息 : 这是一条全部推送的消息 // sub4 收到消息 : 这是一条全部推送的消息 pub.notifySingle(sub2,"这是一条单独推送的消息"); // sub2 收到消息 : 这是一条单独推送的消息 pub.removeSub(sub3); pub.notifyAll('这是一条全部推送的消息'); // sub1 收到消息 : 这是一条全部推送的消息 // sub2 收到消息 : 这是一条全部推送的消息 // sub4 收到消息 : 这是一条全部推送的消息
此代码中拥有同一构造函数的所有对象都含有不同的方法。
sub1.onReceive === sub2.onReceive;//false sub1.onReceive === sub3.onReceive;//false sub1.onReceive === sub4.onReceive;//false sub2.onReceive === sub3.onReceive;//false sub2.onReceive === sub4.onReceive;//false sub3.onReceive === sub4.onReceive;//false
这样会导致:
1. 浪费内存;
2. 不易于对方法进行批量操作。
接下来是改进版本, 使用原型达到代码复用的效果 :
let _indexOf = Array.prototype.indexOf; let _push = Array.prototype.push; let _slice = Array.prototype.slice; let _concat = Array.prototype.concat; let _forEach = Array.prototype.forEach; function Publish(){ this.subList; } Publish.prototype.indexOf = function(sub){ let index = -1; if(typeof this.subList === 'undefined' || this.subList === null){ this.subList = []; } if(typeof sub !== 'undefined' && sub !== null){ index = _indexOf.call(this.subList,sub); } return index; } Publish.prototype.addSub = function(sub){ let index = this.indexOf(sub); index > -1 ? '' : _push.call(this.subList,sub); }; Publish.prototype.removeSub = function(sub){ let index = this.indexOf(sub); index > -1 ? index === 0 ? this.subList = _slice.call(this.subList,1) : this.subList = _concat.call(_slice.call(this.subList,0,index),_slice.call(this.subList,index + 1)) : ''; }; Publish.prototype.notifySingle = function(sub,msg){ let index = this.indexOf(sub); index > -1 ? (typeof sub.onReceive === 'function' ? sub.onReceive(msg) : '') : ''; }; Publish.prototype.notifyAll = function(msg){ if(typeof this.subList !== 'undefined' && this.subList !== null){ _forEach.call(this.subList,(sub)=>{ if(typeof sub !== 'undefined' && sub !== null){ typeof sub.onReceive === 'function' ? sub.onReceive(msg) : ''; } }) } }; function Subscription(name){ this.name = name; } Subscription.prototype.onReceive = function(msg){ console.log(this.name + ' 收到消息 : ' + msg); }; let pub = new Publish(); let sub1 = new Subscription('sub1'); let sub2 = new Subscription('sub2'); let sub3 = new Subscription('sub3'); let sub4 = new Subscription('sub4'); pub.addSub(sub1); pub.addSub(sub1); pub.addSub(sub2); pub.addSub(sub3); pub.addSub(sub4); pub.notifyAll('这是一条全部推送的消息'); // sub1 收到消息 : 这是一条全部推送的消息 // sub2 收到消息 : 这是一条全部推送的消息 // sub3 收到消息 : 这是一条全部推送的消息 // sub4 收到消息 : 这是一条全部推送的消息 pub.notifySingle(sub2,"这是一条单独推送的消息"); // sub2 收到消息 : 这是一条单独推送的消息 pub.removeSub(sub3); pub.notifyAll('这是一条全部推送的消息'); // sub1 收到消息 : 这是一条全部推送的消息 // sub2 收到消息 : 这是一条全部推送的消息 // sub4 收到消息 : 这是一条全部推送的消息
sub1.onReceive === sub2.onReceive;//true sub1.onReceive === sub3.onReceive;//true sub1.onReceive === sub4.onReceive;//true sub2.onReceive === sub3.onReceive;//true sub2.onReceive === sub4.onReceive;//true sub3.onReceive === sub4.onReceive;//true
改进版本与之前的版本相比有一个特点:拥有同一构造函数的对象,属性是唯一的,行为是一致的。所有对象都拥有独立于其它对象的属性,却存在相同的行为。 这正是因为在改进版本中, 方法存在于构造函数的prototype属性值上,其将被其创建的对象所继承 。也正是因为如此, 尽管此时的sub1、sub2、sub3、sub4中都不包含onReceive方法,但也可以通过继承的原型对象Subscription.prototype去达到调用onReceive的目的 。而且 修改Subscription.prototype上的onReceive方法是可以马上作用到sub1、sub2、sub3、sub4上的 。将方法定义到构造函数的prototype属性值上,就可以让拥有相同构造函数的不同对象都具备相同的行为以达到代码复用目的。
instanceof关键字判断对象类型的依据是什么?
我在 深入了解JavaScript中基于原型(prototype)的继承机制 中声明了 函数Person ,并以它为构造函数创建了 person对象 。
function Person(){ } let person = new Person();
person对象的继承Person函数的prototype属性值,而Person函数的prototype属性值又继承Object函数的prototype属性值,这种一层一层继承的关系构成了原型链。
instanceof关键字判断对象类型的依据便是判断函数的prototype属性值是否存在于对象的原型链上。
正如Person函数的prototype属性值和Object函数的prototype属性值都存在于person对象的原型链上,所以使用instanceof判断两者都为true。
person instanceof Person;//true person instanceof Object;//true
而Function函数的prototype属性值不存在于person对象的原型链上,所以使用instanceof判断Function函数为false。
person instanceof Function;//false
最后,完成一个instanceof。
/** * obj 变量 * fn 构造函数 */ function myInstanceof(obj,fn){ let _prototype = Object.getPrototypeOf(obj); if(null === _prototype){ return false; } let _constructor = _prototype.constructor; if(_constructor === fn){ return true; } return myInstanceof(_prototype,fn); } //测试代码 myInstanceof({},Object);//true myInstanceof([],Array);//true myInstanceof(window,Window);//true myInstanceof(new Map(),Map);//true myInstanceof({},Array);//false myInstanceof({},Function);//false
大功告成。
结尾
这3个问题的解答分别对原型和原型链的含义以及它们在JavaScript中起到了什么作用进行了阐述。不过由于本人才疏学浅,难免会遇到一些我个人理解亦或是表达存在错误的地方,还望各位遇到之时,能不吝指出。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK