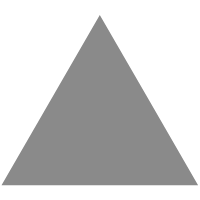
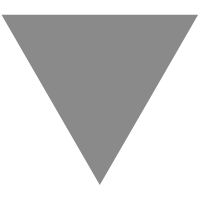
Sum of subsets of all the subsets of an array | O(2^N)
source link: https://www.geeksforgeeks.org/sum-of-subsets-of-all-the-subsets-of-an-array-o2n/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
- Difficulty Level : Hard
- Last Updated : 18 Nov, 2019
Given an array arr[] of length N, the task is to find the overall sum of subsets of all the subsets of the array.
Examples:
Input: arr[] = {1, 1}
Output: 6
All possible subsets:
a) {} : 0
All the possible subsets of this subset
will be {}, Sum = 0
b) {1} : 1
All the possible subsets of this subset
will be {} and {1}, Sum = 0 + 1 = 1
c) {1} : 1
All the possible subsets of this subset
will be {} and {1}, Sum = 0 + 1 = 1
d) {1, 1} : 4
All the possible subsets of this subset
will be {}, {1}, {1} and {1, 1}, Sum = 0 + 1 + 1 + 2 = 4
Thus, ans = 0 + 1 + 1 + 4 = 6Input: arr[] = {1, 4, 2, 12}
Output: 513
Approach: In this article, an approach with O(N * 2N) time complexity to solve the given problem will be discussed.
First, generate all the possible subsets of the array. There will be 2N subsets in total. Then for each subset, find the sum of all of its subset.
For, that it can be observed that in an array of length L, every element will come exactly 2(L – 1) times in the sum of subsets. So, the contribution of each element will be 2(L – 1) times its values.
Below is the implementation of the above approach:
- Python3
filter_none
edit
close
play_arrow
link
brightness_4
code
// C++ implementation of the approach
#include <bits/stdc++.h>
using
namespace
std;
// Function to sum of all subsets of a
// given array
void
subsetSum(vector<
int
>& c,
int
& ans)
{
int
L = c.size();
int
mul = (
int
)
pow
(2, L - 1);
for
(
int
i = 0; i < c.size(); i++)
ans += c[i] * mul;
}
// Function to generate the subsets
void
subsetGen(
int
* arr,
int
i,
int
n,
int
& ans, vector<
int
>& c)
{
// Base-case
if
(i == n) {
// Finding the sum of all the subsets
// of the generated subset
subsetSum(c, ans);
return
;
}
// Recursively accepting and rejecting
// the current number
subsetGen(arr, i + 1, n, ans, c);
c.push_back(arr[i]);
subsetGen(arr, i + 1, n, ans, c);
c.pop_back();
}
// Driver code
int
main()
{
int
arr[] = { 1, 1 };
int
n =
sizeof
(arr) /
sizeof
(
int
);
// To store the final ans
int
ans = 0;
vector<
int
> c;
subsetGen(arr, 0, n, ans, c);
cout << ans;
return
0;
}
6
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK