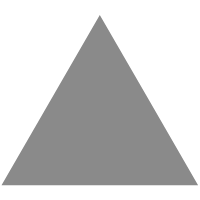
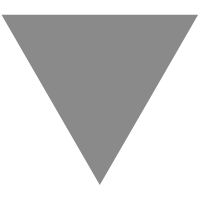
Sum of subsets of all the subsets of an array | O(N)
source link: https://www.geeksforgeeks.org/sum-of-subsets-of-all-the-subsets-of-an-array-on/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
- Difficulty Level : Hard
- Last Updated : 06 Nov, 2019
Given an array arr[] of length N, the task is to find the overall sum of subsets of all the subsets of the array.
Examples:
Input: arr[] = {1, 1}
Output: 6
All possible subsets:
a) {} : 0
All the possible subsets of this subset
will be {}, Sum = 0
b) {1} : 1
All the possible subsets of this subset
will be {} and {1}, Sum = 0 + 1 = 1
c) {1} : 1
All the possible subsets of this subset
will be {} and {1}, Sum = 0 + 1 = 1
d) {1, 1} : 4
All the possible subsets of this subset
will be {}, {1}, {1} and {1, 1}, Sum = 0 + 1 + 1 + 2 = 4
Thus, ans = 0 + 1 + 1 + 4 = 6Input: arr[] = {1, 4, 2, 12}
Output: 513
Approach: In this article, an approach with O(N) time complexity to solve the given problem will be discussed.
The key is observing the number of times an element will repeat in all the subsets.
Let’s magnify the view. It is known that every element will appear 2(N – 1) times in the sum of subsets. Now, let’s magnify the view even further and see how the count varies with the subset size.
There are N – 1CK – 1 subsets of size K for every index that include it.
Contribution of an element for a subset of size K will be equal to 2(K – 1) times its value. Thus, total contribution of an element for all the subsets of length K will be equal to N – 1CK – 1 * 2(K – 1)
Total contribution among all the subsets will be equal to:
N – 1CN – 1 * 2(N – 1) + N – 1CN – 2 * 2(N – 2 + N – 1CN – 3 * 2(N – 3) + … + N – 1C0 * 20.
Now, the contribution of each element in the final answer is known. So, multiply it to the sum of all the elements of the array which will give the required answer.
Below is the implementation of the above approach:
- Python3
filter_none
edit
close
play_arrow
link
brightness_4
code
// C++ implementation of the approach
#include <bits/stdc++.h>
using
namespace
std;
#define maxN 10
// To store factorial values
int
fact[maxN];
// Function to return ncr
int
ncr(
int
n,
int
r)
{
return
(fact[n] / fact[r]) / fact[n - r];
}
// Function to return the required sum
int
findSum(
int
* arr,
int
n)
{
// Intialising factorial
fact[0] = 1;
for
(
int
i = 1; i < n; i++)
fact[i] = i * fact[i - 1];
// Multiplier
int
mul = 0;
// Finding the value of multipler
// according to the formula
for
(
int
i = 0; i <= n - 1; i++)
mul += (
int
)
pow
(2, i) * ncr(n - 1, i);
// To store the final answer
int
ans = 0;
// Calculate the final answer
for
(
int
i = 0; i < n; i++)
ans += mul * arr[i];
return
ans;
}
// Driver code
int
main()
{
int
arr[] = { 1, 1 };
int
n =
sizeof
(arr) /
sizeof
(
int
);
cout << findSum(arr, n);
return
0;
}
6
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK