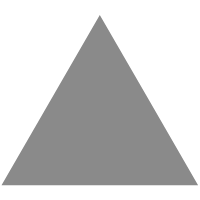
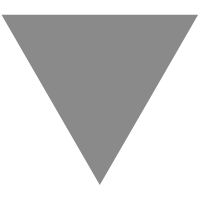
ImageField的使用笔记
source link: https://blog.csdn.net/weixin_44836038/article/details/111410589
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
ImageField的使用笔记
今天完善作业写的订单系统,主要是给每一个菜品增加图片,看起来美观一些,但是没想到这个小小的需求花了我一天时间,记录下来,算增长知识了。
1.配置setting文件
MEDIA_ROOT代表的是上传图片的根目录,MEDIA_URL代表的是访问文件时url的前缀。
# 图片储存根路径
MEDIA_ROOT = join('media')
# 图片访问url
MEDIA_URL = '/IMG/'
2.model里面增加ImageField属性
up_load一定要配置,代表你最后的图片会存储到MEDIA_ROOT/up_load(实际上是你赋予的名称)这个文件夹中。
class Menu(models.Model):
"""
餐品数据库
"""
ID = models.BigAutoField(primary_key=True,editable=False)
lastEditTime = models.DateTimeField(auto_now_add=True)
merchantID = models.ForeignKey(Usr, verbose_name="商家账号", on_delete=models.CASCADE,to_field='ID')
itemName = models.CharField(max_length=20,verbose_name="餐品名")
itemText = models.TextField(verbose_name="餐品简介")
price = models.FloatField(verbose_name="餐品价格")
################# up_load代表你上传图片所存储的文件夹名字
picture = models.ImageField(verbose_name='餐品图片',null=True,upload_to='img/')
class Meta:
db_table = "Menu"
verbose_name = "餐品数据表"
ordering=['-lastEditTime']
3.Form表单类
本项目使用的是django自带的Form表单类进行数据的传递。
class MerchantDish(forms.Form):
"""
商家菜品提交表单
"""
itemName = forms.CharField(max_length=20,label="餐品名")
itemText = forms.CharField(max_length=300,label="餐品简介")
price = forms.FloatField(label="餐品价格")
picture = forms.ImageField(label='餐品图片')
4.html模板文件(增加菜品)
注意一定要添加:enctype=“multipart/form-data”。
<form action="updateDish_post/" method="post" enctype="multipart/form-data">
{% csrf_token %} {{form.as_p}}
<button type="submit">修改</button>
<button type="button"><a href="/MerchantSystem/DelDish/{{dishID}}/">删除</a></button>
</form>
5.显示菜品的html模板文件
重要的是src中路径的配置,有两种方法,建议法一,自己感觉比较安全,就算没有picture时也不会报错。(注意:可调整图片显示大小)
法一:/IMG(你自己定义的MEDIA_URL)/{{dish.picture}} ----dish代表后端传来的菜品,dish.picture代表你使用的这个类中的那个有ImageField属性的字段;
法二:{{dish.picture.url}} 因为ImageField是文件类,里面有三个属性name、path、url可以直接访问。
{% for dish in menu %}
<!--将目录的数据展示在html中-->
<!-- 提交到一个含参数的url注意后端的接收 -->
<form action="/MerchantSystem/Dish/{{dish.ID}}/" method="post">
{% csrf_token %}
<li class="media">
<div class="media-left media-middle" >
<img class="media-object" width="150" height="150" src="/IMG/{{dish.picture}}" alt="">
</div>
<div class="media-body">
<h4 class="media-heading">
<button type='submit' class=" url" title="更新菜品信息">
菜名:{{dish.itemName|default:"Null"}}
</button>
<span class="label label-default">
价格:{{dish.price|default:"Null"}}
</span>
</h4>
简介:{{dish.itemText|default:"Null"}}
</div>
</li>
</form>
{% empty %}
<!--若中无数据展示如下内容-->
<p>暂无数据..</p>
{% endfor %} {% endblock tableBody %}
6.路径静态化
在所有的url中都要配置如下:urlpatterns + static…
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
path('', views.base_view, name = "base"),# 顾客服务系统
path('order/<int:dishID>/', views.order_view),# 订单详情
path('order/<int:dishID>/submit/',views.order_submit),# 提交订单
path('pay/<int:orderID>/', views.pay_view),# 缴费
path('pay/<int:orderID>/submit/',views.pay_submit),#确认账单
path('order/list/',views.order_list_view),#历史订单列表
path('order/confirm/<int:orderID>/',views.order_confirm),#订单确认收到
path('order/comment/<int:orderID>/',views.comment),#到达相应菜品的评论界面
path('order/comment_post/<int:orderID>/',views.comment_post)#提交评论
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
7.修改上传的图片
首先用form表单上传图片,检查有效之后,把cleaned_data中的picture数据赋值给要更新对象中的picture属性,最后save即可。代码如下:
def updateDish_post(request,dishID):
"""
接受修改菜品的请求
"""
dish_form = MerchantDish(request.POST,request.FILES)
if dish_form.is_valid() :
dish = Menu.objects.get(ID = dishID)
dish.itemName = dish_form.cleaned_data['itemName']
dish.itemText = dish_form.cleaned_data['itemText']
dish.price = dish_form.cleaned_data['price']
dish.picture = dish_form.cleaned_data['picture']
dish.save()
# dishChange = dish_form.clean()
return redirect('/MerchantSystem/')
elif dish_form.errors is not None:
print(dish_form.errors)
return HttpResponse(str(dish_form.errors))
8.设置默认图片
这个步骤我查了好久的资料,但是都不行,好像不可以直接在model.py文件中设置default,我最后都快放弃了,但是自己还是凭运气试出来了,不知道原理,但还是放在这,希望对大家有帮助。
方法是在显示图片的html模板中的src处写一个default,代码如下:
dish是后端传过来的参数,default指向的是默认图片所在的位置。
<div class="media-left media-middle" >
<!-- {{dish.url|default:"Null"}} -->
<img class="media-object" width="150" height="150" src="/IMG/{{dish.picture|default:'img/default.jpg'}}" alt="">
</div>
参考资料:
ImageField的使用:https://www.jianshu.com/p/6107e9843ee1
默认图片的赋值(感觉方法不行):https://www.cnblogs.com/haoshine/p/5447181.html
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK