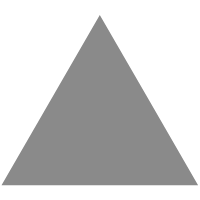
9
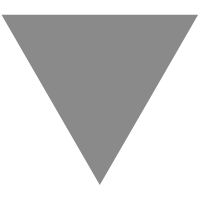
3 simple ways to create an error
source link: https://yourbasic.org/golang/create-error/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
3 simple ways to create an error
yourbasic.org/golang
String-based errors
The standard library offers two out-of-the-box options.
// simple string-based error
err1 := errors.New("math: square root of negative number")
// with formatting
err2 := fmt.Errorf("math: square root of negative number %g", x)
Custom errors with data
To define a custom error type, you must satisfy the predeclared error
interface.
type error interface {
Error() string
}
Here are two examples.
type SyntaxError struct {
Line int
Col int
}
func (e *SyntaxError) Error() string {
return fmt.Sprintf("%d:%d: syntax error", e.Line, e.Col)
}
type InternalError struct {
Path string
}
func (e *InternalError) Error() string {
return fmt.Sprintf("parse %v: internal error", e.Path)
}
If Foo
is a function that can return a SyntaxError
or an InternalError
, you may handle the two cases like this.
if err := Foo(); err != nil {
switch e := err.(type) {
case *SyntaxError:
// Do something interesting with e.Line and e.Col.
case *InternalError:
// Abort and file an issue.
default:
log.Println(e)
}
}
More code examples
Go blueprints: code for common tasks is a collection of handy code examples.
Share:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK