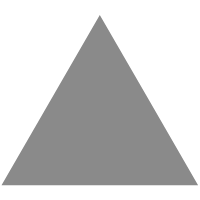
5
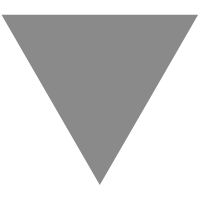
Compute max of two ints/floats
source link: https://yourbasic.org/golang/max-min-function/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Compute max of two ints/floats
yourbasic.org/golang
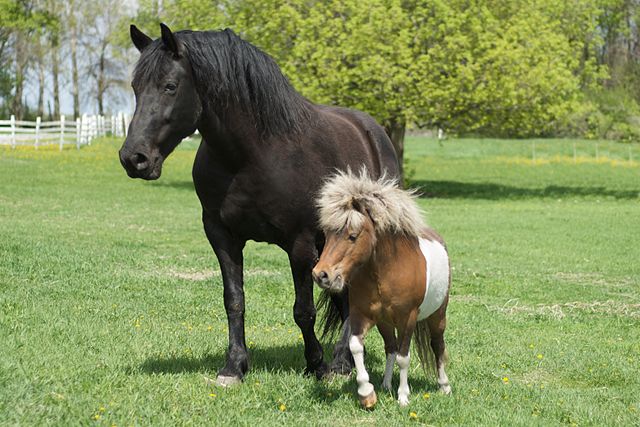
Integers
There is no built-in max or min function for integers, but it’s simple to write your own.
// Max returns the larger of x or y.
func Max(x, y int64) int64 {
if x < y {
return y
}
return x
}
// Min returns the smaller of x or y.
func Min(x, y int64) int64 {
if x > y {
return y
}
return x
}
Floats
For floats, use math.Max
and math.Min
.
// Max returns the larger of x or y.
func Max(x, y float64) float64
// Min returns the smaller of x or y.
func Min(x, y float64) float64
Special cases:
Max(x, +Inf) = Max(+Inf, x) = +Inf
Max(x, NaN) = Max(NaN, x) = NaN
Max(+0, ±0) = Max(±0, +0) = +0
Max(-0, -0) = -0
Min(x, -Inf) = Min(-Inf, x) = -Inf
Min(x, NaN) = Min(NaN, x) = NaN
Min(-0, ±0) = Min(±0, -0) = -0
More code examples
Go blueprints: code for common tasks is a collection of handy code examples.
Share:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK