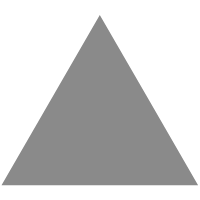
5
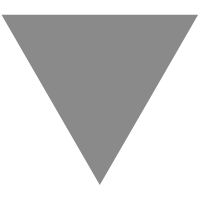
Vectorised Logical Operations in Java 9
source link: https://richardstartin.github.io/posts/vectorised-logical-operations-in-java-9
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Vectorised Logical Operations in Java 9
Oct 1, 2017 | Richard Startin | javavector
This is a short post for my own reference, since I feel I have already done the topic of does Java 9 use AVX for this? to death. Cutting to the chase, Java 9 autovectorises loops to compute logical ANDs, XORs, ORs and ANDNOTs between arrays, making use of the instructions VPXOR
, VPOR
and VPAND
. You can replicate this by running the code at github.
@Benchmark
public long[] xor(LongData state) {
long[] result = new long[state.data1.length];
long[] data1 = state.data1;
long[] data2 = state.data2;
for (int i = 0; i < data1.length && i < data2.length; ++i) {
result[i] = data1[i] ^ data2[i];
}
return result;
}
vmovdqu ymm0,ymmword ptr [r10+r13*8+10h] vpxor ymm0,ymm0,ymmword ptr [rbx+r13*8+10h] vmovdqu ymmword ptr [rax+r13*8+10h],ymm0
@Benchmark
public long[] or(LongData state) {
long[] result = new long[state.data1.length];
long[] data1 = state.data1;
long[] data2 = state.data2;
for (int i = 0; i < data1.length && i < data2.length; ++i) {
result[i] = data1[i] | data2[i];
}
return result;
}
vmovdqu ymm0,ymmword ptr [r10+rsi*8+30h] vpor ymm0,ymm0,ymmword ptr [rbx+rsi*8+30h] vmovdqu ymmword ptr [rax+rsi*8+30h],ymm0
@Benchmark
public long[] and(LongData state) {
long[] result = new long[state.data1.length];
long[] data1 = state.data1;
long[] data2 = state.data2;
for (int i = 0; i < data1.length && i < data2.length; ++i) {
result[i] = data1[i] & data2[i];
}
return result;
}
vmovdqu ymm0,ymmword ptr [r10+r13*8+10h] vpand ymm0,ymm0,ymmword ptr [rbx+r13*8+10h] vmovdqu ymmword ptr [rax+r13*8+10h],ymm0
ANDNOT
@Benchmark
public long[] andNot(LongData state) {
long[] result = new long[state.data1.length];
long[] data1 = state.data1;
long[] data2 = state.data2;
for (int i = 0; i < data1.length && i < data2.length; ++i) {
result[i] = data1[i] & ~data2[i];
}
return result;
}
vpunpcklqdq xmm0,xmm0,xmm0 vinserti128 ymm0,ymm0,xmm0,1h vmovdqu ymm1,ymmword ptr [rbx+r13*8+10h] vpxor ymm1,ymm1,ymm0 vpand ymm1,ymm1,ymmword ptr [r10+r13*8+10h] vmovdqu ymmword ptr [rax+r13*8+10h],ymm1
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK