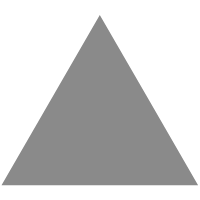
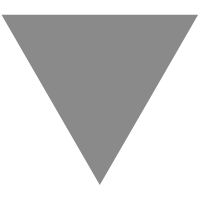
Closures in JavaScript: Video
source link: https://debugmode.net/2020/12/13/closures-in-javascript-video/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Closures in JavaScript: Video
A JavaScript closure is a function which remembers the environment in which it was created. We can think of it as an object with one method and private variables. JavaScript closures are a special kind of object which contains the function and the local scope of the function with all the variables (environment) when the closure was created.
// normal closure function Add(num1) { function Addfoo(num2) { let result = num1 + num2; return result; } return Addfoo; } var a1 = Add(9)(2); console.log(a1); var b1 = Add(15)(12); console.log(b1); // counter function counter(num1) { let c = num1; function count() { c = c + 1; return c; } return count; } var a = counter(2); console.log(a()); console.log(a()); console.log(a()); console.log(a()); var b = counter(100); console.log(b()); console.log(b()); console.log(b()); console.log(b());
Rate this:
Related
Variable Scoping and Hoisting in JavaScript
Variable Scoping in JavaScript is quite different than other programming languages like C. In this post we will learn variable scoping in JavaScript. Simply putting, Scope of a JavaScript variable is within region of the program it is defined. So a variable defined inside function will have scope inside that…
In "JavaScript"
Working with objects in a JavaScript Arrow Function
The JavaScript Arrow function is a shorter way of writing a function expression. Let us say you have a function expression as below, var add = function (num1, num2) { return num1 + num2; } The above function can refactor for a shorter syntax using the arrow function as below, var add = (num1, num2) => num1 + num2; So, as you see, the arrow function provides…
In "JavaScript"
Invocation patterns in JavaScript
In JavaScript you can invoke a function in four different ways. In this post we will take a look on various invocation patterns. There are four ways a function can be invoked in JavaScript. Four invocation patterns in JavaScript are as follows, Function Invocation Pattern Method Invocation Pattern Constructor Invocation…
In "JavaScript"
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK