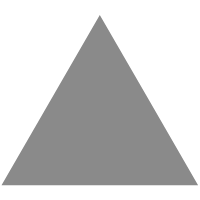
5
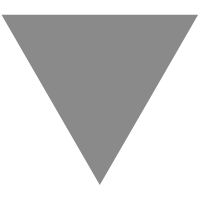
Create a list of objects from a file using lambda expressions
source link: https://marco.dev/java-read-file-list-lambda/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The lambda expression
public List<Conference> readInputStream(InputStream inputStream) throws IOException {
// target list
List conferenceModelCollection;
try (
// open the url stream, wrap it an a few "readers"
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
// read the file, line by line
conferenceModelCollection = reader.lines()
// skip the first 4 lines - contain metadata
.skip(4)
// take the line and convert into an object
.map(this::convertToConference)
// sort the lines by date
.sorted((f1, f2) -> f1.getBegin().compareTo(f2.getEnd()))
// add to the existing collection
.collect(Collectors.toList());
}
return conferenceModelCollection;
}
Example of the converter
private Conference convertToConference(String dataLine) {
String[] data = dataLine.split("\\|");
int dataPosition = 1;
Conference conference = new Conference();
conference.setName(data[dataPosition++]);
conference.setWebsite(data[dataPosition++]);
conference.setBegin(LocalDate.parse(data[dataPosition++], DATE_FORMATTER));
...
return conference;
}
Read the file
private List<Conference> readCalendarDataFromUrl() throws IOException {
URL url = new URL(urlString);
return readInputStream(url.openStream());
}
Author
Marco Molteni
Marco Molteni Blog
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK