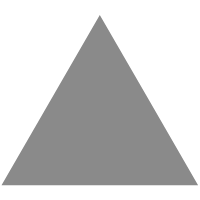
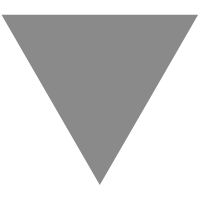
这些Stream流的常用方法你得记住,步骤简单不麻烦!
source link: http://www.cnblogs.com/lwh1019/p/14044639.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
forEach遍历
/*
forEach:该方法接收一个Consumer接口函数,将每一个流元素交给该函数处理
简单记:
forEach方法:用来遍历流中的数据
是一个终结方法,遍历之后就不能继续调用Stream流中的其他方法
*/
public class demo01Stream_ForEach { public static void main(String[] args) { //获取一个Stream流 Stream<String>stream= Stream.of("张三","李四","王五","赵六"); //使用Stream流的方法forEach对stream流中的数据遍历 stream.forEach((String name)->{ System.out.println(name); }); } }
filter过滤
/*
filter:用于对Stream流中的数据进行过滤
filter(Predicate<? super T>predicate)
filter方法的参数Predicate是一个函数式接口,可以使用lambda表达式
Predicate中的抽象方法
boolean test(T t)
*/
public class demo01Stream_filter { public static void main(String[] args) { //创建一个Stream流 Stream<String> stream = Stream.of("张三", "李四", "王五", "赵六", "冯老七"); Stream<String> stream1 = stream.filter((String name) -> { return name.startsWith("冯"); }); //遍历stream1 stream1.forEach((name)-> System.out.println(name)); } }
Stream流属于管道流,只能被消费一次
第一个Stream流调用完毕方法,数据会流转到下一个流中
第一个流会关闭,不再被调用
map方法转换流
/*
将流中的元素映射到另一个流中,使用map方法
该接口需要一个Function函数式接口参数,可以将当前流中的T类型数据转换为另一种R类型的流
Function中的抽象方法:
R apply(T t)
*/
public class demoStream_map { public static void main(String[] args) { //获取一个String类型的stream流 Stream<String>stream = Stream.of("1","2","3","4"); //使用map方法,把字符串类型的整数,转换(映射)为Integer类型的整数 Stream<Integer> stream1 = stream.map((String s) -> { return Integer.parseInt(s); }); stream1.forEach((i)-> System.out.println(i)); } }
count方法 统计个数
/*
count方法 用于统计Stream流中的元素个数
long count();
count方法是一个终结方法,返回值是一个long类型的整数
不能再调用Stream流其他方法
*/
public class demoStream_count { public static void main(String[] args) { ArrayList<Integer> list= new ArrayList<>(); list.add(1); list.add(2); list.add(3); list.add(4); list.add(5); Stream<Integer> stream = list.stream(); long count = stream.count(); System.out.println(count); } }
limit截取元素
/*
limit:用于截取流中的元素
limit可以对流进行截取,只取用前n个
limit(long maxSize);
参数是一个long型,如果集合当前长度大于参数则进行截取,否则不进行操作
limit是一个延迟方法,可以继续使用Stream流方法
*/
public class demoStream_limit { public static void main(String[] args) { String[] arr= {"xxx","wwq","wqew","wewqewqe"}; Stream<String> stream = Stream.of(arr); Stream<String> stream1 = stream.limit(2); stream1.forEach((name)-> System.out.println(name)); } }
skip方法跳过元素
/*
skip方法:用于跳过元素
skip(long n)
如果流的当前长度大于n,则跳过前n个,否则将会得到一个长度为0的空流
*/
public class demoStream_skip { public static void main(String[] args) { String[] arr= {"xxx","wwq","wqew","wewqewqe"}; Stream<String> stream = Stream.of(arr); Stream<String> stream1 = stream.skip(2); stream1.forEach((name)-> System.out.println(name)); } }
concat方法合并流
/*
concat:用于把流组合到一起
如果有两个流,希望合并成为一个流,可以使用Stream接口的静态方法concat
*/
public class demoStream_concat { public static void main(String[] args) { Stream<String> stream = Stream.of("张三", "李四", "王五", "赵六", "冯老七"); String[] arr= {"QWQ", "ERE", "TYT", "UIU", "OIO"}; Stream<String> stream1 = Stream.of(arr); //合并 Stream<String> stream2 = Stream.concat(stream, stream1); stream2.forEach((name)-> System.out.print(name+" ")); } }
最后
欢迎关注公众号:前程有光,领取一线大厂Java面试题总结+各知识点学习思维导+一份300页pdf文档的Java核心知识点总结!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK