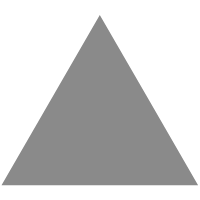
16
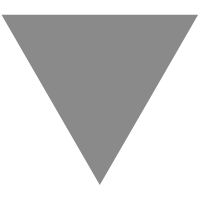
golang 策略模式之排序算法策略
source link: https://studygolang.com/articles/31465
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
起源
最近在学设计模式,这个东西学起来,总是模模糊糊,看起来懂,又不知该应用到何处,咬着牙学完了之后,准备学习算法,写了两个简单的排序算法,突然灵光一闪,如果我想用不用的算法去排序的时候,完全可以用策略模式,正好学以致用
示例
我先设计了一个排序接口
type sortAlgo interface { sort([]int) }
然后,我写了两个算法,一个是冒泡排序,一个是简单选择排序,都实现了这个接口
type bubbleSort struct { } func (b *bubbleSort) sort(data []int) { n := len(data) for i:=0; i<n-1; i++ { for j:=n-1; j>i; j-- { if data[j-1] > data[j] { tmp := data[j-1] data[j-1] = data[j] data[j] = tmp } } }} type simpleSelectSort struct { } func (s *simpleSelectSort) sort(data []int) { n := len(data) for i:=0; i<n; i++ { minIndex := i for j:=i+1; j<n; j++ { if data[j] < data[minIndex] { minIndex = j } } if minIndex != i { tmp := data[minIndex] data[minIndex] = data[i] data[i] = tmp } }}
最后,我构建一个策略模式,可以通过设计,决定使用哪一个算法
type sortStrategy struct { data []int sortAlgo } func (s *sortStrategy) setAlgo(sa sortAlgo) { s.sortAlgo = sa } func (s *sortStrategy) setData(data []int) { s.data = data } func (s *sortStrategy) run() { s.sortAlgo.sort(s.data) }
总结
说实话,这么写有点Java了,但是我看的设计模式的书,就是基于Java的,先学习思想嘛
我脑海中,现在还蹦出来另一个思路,排序时,接收一个排序方法,再调用
type sortFunc func([]int)
这样是不是更gopher了呢
有疑问加站长微信联系(非本文作者)

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK