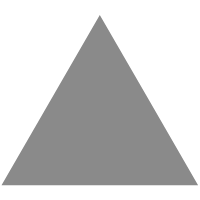
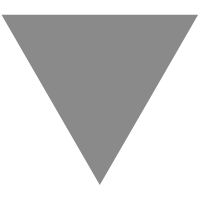
spring-boot-route(九)整合JPA操作数据库
source link: https://segmentfault.com/a/1190000030686550
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
单调的增删改查让越来越多的程序员感到乏味,这时候就出现了很多优秀的框架,完成了对增删改查操作的封装,只需要简单配置,无需书写任何sql,就可以完成增删改查。这里比较推荐的是Spring Data Jpa。
Spring Data JPA是Spring Data家族的一部分,可以轻松实现基于JPA的存储库。 此模块处理对基于JPA的数据访问层的增强支持。 它使构建使用数据访问技术的Spring驱动应用程序变得更加容易。
我们继续使用前两章用的数据库结构来进行演示。
一 引入mysql和spring-data-jpa依赖
<dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
二 创建实体类
@Data @NoArgsConstructor @AllArgsConstructor @Entity public class Student implements Serializable { private static final long serialVersionUID = 6712540741269055064L; @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Integer studentId; private Integer age; private String name; private Integer sex; private Date createTime; private Integer status; }
@GeneratedValue是主键生成策略,Jpa自带的几种主键生成策略如下:
- TABLE: 使用一个特定的数据库表格来保存主键
- SEQUENCE: 根据底层数据库的序列来生成主键,条件是数据库支持序列。这个值要与generator一起使用,generator 指定生成主键使用的生成器(可能是orcale中自己编写的序列)
- IDENTITY: 主键由数据库自动生成(主要是支持自动增长的数据库,如mysql)
- AUTO: 主键由程序控制,也是GenerationType的默认值
主键生成策略扩展
自定义主键生成器:
public class MyGenerator implements IdentifierGenerator { @Override public Serializable generate(SharedSessionContractImplementor sharedSessionContractImplementor, Object o) throws HibernateException { return getId(); } public static long getId(){ return System.currentTimeMillis(); } }
然后在实体类做一下配置:
@Data @NoArgsConstructor @AllArgsConstructor @Entity public class Student implements Serializable { private static final long serialVersionUID = 6712540741269055064L; @Id @GenericGenerator(name="idGenerator",strategy = "com.javatrip.springdatajpa.entity.MyGenerator") @GeneratedValue(generator = "idGenerator") private Integer studentId; private Integer age; private String name; private Integer sex; private Date createTime; private Integer status; }
三 创建dao接口
dao层接口实现JpaRepository,泛型选择pojo和其主键类型,就会自动实现简单的CRUD等接口,无需手动开发,就能快速进行调用。
public interface StudentRepository extends JpaRepository<Student,Integer> { /** * 根据年龄,名字模糊查询 * @return */ Student findByNameLikeAndAge(String name,int age); }
Jpa除了实现CRUD方法,还支持字段名模糊查询等各种不用手写sql的操作。
四 测试类测试CRUD
@SpringBootTest class SpringDataJpaApplicationTests { @Autowired StudentRepository repository; @Test void contextLoads() { // 查询所有实体 List<Student> all = repository.findAll(); // 根据id查询实体类 Optional<Student> byId = repository.findById(100); // 根据id删除数据 repository.deleteById(100); // 插入一条数据 // 如果数据库存在该实体的主键,则更新,否则插入 Student student = new Student(); student.setAge(18); student.setName("Java旅途"); repository.save(student); repository.findByNameLikeAndAge("Java",18); } }
spring-data-jpa在外国程序员界非常普遍。相比其他两种方式,它不需要写sql就可以完成非常完善的数据操作,我也是比较推荐使用它作为orm框架。
此是spring-boot-route系列的第九篇文章,这个系列的文章都比较简单,主要目的就是为了帮助初次接触Spring Boot 的同学有一个系统的认识。本文已收录至我的 github
,欢迎各位小伙伴 star
!
github: https://github.com/binzh303/s...
点关注、不迷路
如果觉得文章不错,欢迎 关注 、 点赞 、 收藏 ,你们的支持是我创作的动力,感谢大家。
如果文章写的有问题,请不要吝啬,欢迎留言指出,我会及时核查修改。
如果你还想更加深入的了解我,可以微信搜索「 Java旅途 」进行关注。回复「 1024 」即可获得学习视频及精美电子书。每天7:30准时推送技术文章,让你的上班路不在孤独,而且每月还有送书活动,助你提升硬实力!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK