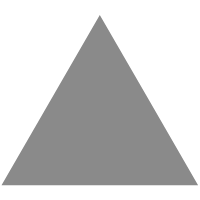
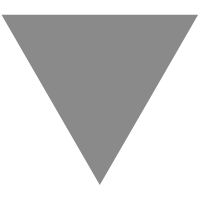
SpringBoot + Spring Cloud Eureka 服务注册与发现
source link: http://www.cnblogs.com/sword-successful/p/13376114.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
什么是Spring Cloud Eureka
Eureka是Netflix公司开发的开源服务注册发现组件,服务发现可以说是微服务开发的核心功能了,微服务部署后一定要有服务注册和发现的能力,Eureka就是担任这个角色。如果你用过Dubbo的话,Dubbo里服务注册和发现就是通过Zookeeper框架完成的。
Eureka 目前是2.2.x版本,目前官方已经宣布不再维护和更新了,不过Eureka 做注册中心已经在生产环境中大规模使用了,可以说很稳定了。从我个人的角度看,目前大家使用的更多的是阿里的 Nacos 和 Consul 这两个组件实现了不止服务发现和注册,微服务开发不用再去依赖更多的组件和框架。这篇文章模拟一下Eureka Server集群和服务提供者集群和服务消费。
版本说明
SpringCloud + SpringBoot开发微服务并不是版本越新越好,Spring Cloud官方提供了一个版本对应关系。目前最新的就是Hoxton, 对应SpringBoot 2.2.x版本。

准备工作
- 新建父工程, 主要约定SpringCloud, SpringBoot版本号,我使用的是Hoxton.SR1, SpringBoot2.2。
- 新建5个子Module,这里两个Eureka Server,两个Service,一个consumer, 两个Eureka Servr我用7001和7002端口来模拟和区分Eureka集群,两个Service 用8001和8002来模拟,主要是想在服务消费时反应客户端负载均衡。
项目结构如下图
父工程pom.xml
<dependencyManagement> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>2.2.2.RELEASE</version> <type>pom</type> <scope>import</scope> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>Hoxton.SR1</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement>
服务注册中心
服务提供者我用了7001和7002模拟集群,两个程序代码都是相同。
新建Module,添加spring-cloud-starter-netflix-eureka-server。
pom.xml
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-server</artifactId> </dependency> </dependencies>
application.yml
server: port: 7001 eureka: client: service-url: defaultZone: http://eureka7002.com:7002/eureka # 集群就是指向其他配置中心 register-with-eureka: false fetch-registry: false instance: hostname: eureka7001.com #eureka服务端实例名称 server: enable-self-preservation: false
修改启动类
@SpringBootApplication @EnableEurekaServer public class MyEurekaServer7001 { public static void main(String[] args) { SpringApplication.run(MyEurekaServer7001.class,args); } }
我这里贴出了7001的代码,7002唯一不同的地方在application.yml配置里,如果是集群的话eureka.client.service-url.defaultZone需要配置集群里其他server节点。这里instance.hostname配置为eureka7001.com, 对应配置需要修改下本机hosts
127.0.0.1 eureka7001.com 127.0.0.1 eureka7002.com
启动两个注册中心服务
eureka7001

eureka7002

服务启动后,注意到DS Replicas中包含集群中另一台机器,说明集群已生效。
服务提供者
服务提供者我这里用了8001和8002两个来模拟集群。

pom.xml
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> </dependencies>
application.yml
server: port: 8001 spring: application: name: CLOUD-STUDENT-SERVICE eureka: client: register-with-eureka: true #集群节点下需要设置为true,才能配合客户端使用Ribbon 负载均衡 fetch-registry: true service-url: defaultZone: http://eureka7001.com:7001/eureka, http://eureka7002.com:7002/eureka #集群需要配置所有注册中心 instance: instance-id: student-service8001 prefer-ip-address: true # 访问路径可以显示ip地址
添加一个服务接口
@RestController @RequestMapping("/student") public class StudentController { @GetMapping("/list") public List<String> list(){ return Arrays.asList("Kobe","Lin","Tim","James"); } @GetMapping("/version") public String version(){ return "8001,202007162310"; } }
修改启动类
@SpringBootApplication @EnableEurekaClient public class MyStudentService8001 { public static void main(String[] args) { SpringApplication.run(MyStudentService8001.class,args); } }
依次启动两个服务提供者8001和8002。服务启动后无错误的情况下刷新Eureka Server界面。
eureka7001

eureka7002

在Application里已经看到CLOUD-STUDENT-SERVICE的状态中已经有两个服务注册进来了。
服务消费者
服务消费者我就写了一个Module,端口8087
pom.xml
<dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> </dependencies>
application.yml
这里客户端消费服务时需要配置集群中的两个注册中心地址。
server: port: 8087 spring: application: name: student-consumer eureka: client: fetch-registry: true register-with-eureka: false service-url: defaultZone: http://eureka7001.com:7001/eureka, http://eureka7002.com:7002/eureka
服务消费接口
服务消费我们仍然用的RestTemplate,客户端负载均衡用的实际上是Ribbon组件,默认使用轮训算法。
@RestController @RequestMapping("/student") public class StudentController { @Autowired RestTemplate restTemplate; @GetMapping("/version") public String index(){ return restTemplate.getForObject("http://CLOUD-STUDENT-SERVICE/student/version",String.class); } }
修改启动类
我在启动类还配置了RestTemplate,启动类两个关键注解SpringBootApplication和EnableEurekaClient。
@SpringBootApplication @EnableEurekaClient public class StudentConsumer8087 { @Bean @LoadBalanced public RestTemplate restTemplate(){ return new RestTemplate(); } public static void main(String[] args) { SpringApplication.run(StudentConsumer8087.class,args); } }
启动并访问服务消费者接口 http://localhost:8087/student/version , 从输出结果能看到会轮训调用8001和8002的接口8002,202007162310,8001,202007162310。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK