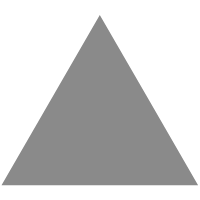
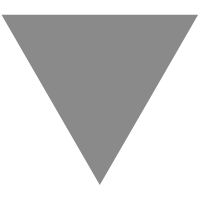
TypeDraft: Language is the New Framework
source link: https://medium.com/@mistlog/typedraft-language-is-the-new-framework-84dfc433971f
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
TypeDraft is a superset of TypeScript and adds DSL and Macro mechanism to the language. If TypeScript is JavaScript that scales, then TypeDraft intends to be TypeScript that scales.
DSL
In this part, we will go through 3 DSLs: debug, match, and watch.
The syntax of DSL is:
{ "use <dsl name>"; ... }
Debug: Conditional Compilation
We can build different versions of a library by specifying different environment variables. For example, in Implement a DSL , we implemented a DSL named as “debug”:
let value = 1;{ "use debug"; console.log(`[Debug]: value is ${value}`); }
The result of compilation depends on the NODE_ENV
you provided in npm script:
"scripts": { "build": "cross-env NODE_ENV=production td ./src", "dev": "cross-env NODE_ENV=dev td ./src" },
In dev mode, the output will be exactly:
let value = 1; console.log(`[Debug]: value is ${value}`);
In production mode, everything in the context of “debug” will be removed:
let value = 1;
In this way, we can achieve the goal of conditional compilation. For example, if you have code for both dev and production:
let url: string = ""; { "use dev"; url = ... // address in test environment }{ "use production"; url = ... // address in production environment }
then you can generate two different versions of library without any runtime code.
Match: Simplify If-Else
We can simplify logic of if-else with “match”, if we have code such as:
let filtered; let currentFilter = 'all';filtered = currentFilter === 'all' ? items : currentFilter === 'completed' ? items.filter(item => item.completed) : items.filter(item => !item.completed);
In DSL match, we will write it in this way:
let filtered; let currentFilter = 'all';{ "use match"; (currentFilter: "all") => filtered = items; (currentFilter: "completed") => filtered = items.filter(item => item.completed); () => filtered = items.filter(item => !item.completed); }
this example can be found in svelte-draft-todo-mvc , for svelte-draft, see Svelte with TypeScript .
Watch
We can write intutive code with “watch” to implement the traditional counter demo:
export default function App() { let count = 0; function handleClick() { count += 1; } let doubled: number; { "use watch"; doubled = count * 2; } <button onClick={handleClick}> Clicked {count} {count === 1 ? 'time' : 'times'} </button>; <p>{count} doubled is {doubled}</p>}
you can find this example in svelte-draft-tutorial/2-reactivity/reactive-declarations/App.tsx .
Macro
The macro support in typedraft is primitive, but still useful. JSX is used to define and use a macro, for this part, see JSX as Macro .
Together with macro, typedraft supports literate programming paradigm.
Ray Tracing in Literate Programming is a good example because the site itself is generated from code, you can find the usage of both macro and svelte-draft.
For example, the skeleton of a section is sketched by:
export default function Section() { let canvas: HTMLCanvasElement; onMount(() => { function generateColor(ray: Ray) { <GenerateColor />; }; <RenderImage />; }); let width = 600; let height = 300; <div class="container"> <canvas bindRef={canvas} width={width} height={height}></canvas> </div> }
Then, implementation of macro RenderImage
and GenerateColor
is placed in other places to keep the structure of code clear and easy to understand.
For more info about typedraft, see https://mistlog.github.io/typedraft-docs/docs/ . I’m sorry that this article is mainly example and link driven, natural language is so hard to deal with!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK