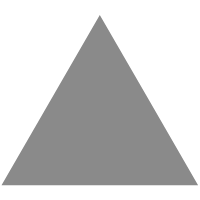
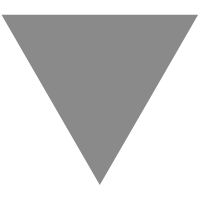
玩转数据处理120题|Pandas版本
source link: http://mp.weixin.qq.com/s?__biz=MzI1MTE2ODg4MA%3D%3D&%3Bmid=2650072885&%3Bidx=1&%3Bsn=81629853a68881627b0b7c9a4c74c605
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Pandas进阶修炼120题系列一共涵盖了数据处理、计算、可视化等常用操作,希望通过120道精心挑选的习题吃透pandas。并且针对部分习题给出了 多种解法与注解 ,动手敲一遍代码一定会让你有所收获!
1
创建DataFrame
题目 :将下面的字典创建为DataFrame
data = {"grammer":["Python","C","Java","GO",np.nan,"SQL","PHP","Python"],
"score":[1,2,np.nan,4,5,6,7,10]}
难度 ::star:
期望结果
Python解法
import numpy as np
import pandas as pd
df = pd.DataFrame(data)
# 假如是直接创建
df = pd.DataFrame({
"grammer": ["Python","C","Java","GO",np.nan,"SQL","PHP","Python"],
"score": [1,2,np.nan,4,5,6,7,10]})
注:1-20题均基于该数据框给出
2
数据提取
题目 :提取含有字符串 "Python" 的行
难度 ::star::star:
期望结果
grammer score
0 Python 1.0
7 Python 10.0
Python解法 :
#> 1
df[df['grammer'] == 'Python']
#> 2
results = df['grammer'].str.contains("Python")
results.fillna(value=False,inplace = True)
df[results]
3
提取列名
题目 :输出df的所有列名
难度 : :star:
期望结果
Index(['grammer', 'score'], dtype='object')
Python解法
df.columns
4
修改列名
题目 :修改第二列列名为 'popularity'
难度 ::star::star:
Python解法
df.rename(columns={'score':'popularity'}, inplace = True)
5
字符统计
题目 :统计 grammer 列中每种编程语言出现的次数
难度 ::star::star:
Python解法
df['grammer'].value_counts()
6
缺失值处理
题目 :将空值用上下值的平均值填充
难度 : :star::star::star:
Python解法
# pandas里有一个插值方法,就是计算缺失值上下两数的均值
df['popularity'] = df['popularity'].fillna(df['popularity'].interpolate())
7
数据提取
题目 :提取 popularity 列中值大于3的行
难度 ::star::star:
Python解法
df[df['popularity'] > 3]
8
数据去重
题目 :按照 grammer 列进行去重
难度 : :star::star:
Python解法
df.drop_duplicates(['grammer'])
9
数据计算
题目 :计算 popularity 列平均值
难度 : :star::star:
Python解法
df['popularity'].mean()
# 4.75
10
格式转换
题目 :将 grammer 列转换为list
难度 : :star::star:
Python解法
df['grammer'].to_list()
# ['Python', 'C', 'Java', 'GO', nan, 'SQL', 'PHP', 'Python']
11
数据保存
题目 :将DataFrame保存为EXCEL
难度 : :star::star:
Python解法
df.to_excel('filename.xlsx')
12
数据查看
题目 :查看数据行列数
难度 : :star:
Python解法
df.shape
# (8, 2)
13
数据提取
题目 :提取 popularity 列值大于3小于7的行
难度 : :star::star:
Python解法
df[(df['popularity'] > 3) & (df['popularity'] < 7)]
14
位置处理
题目 :交换两列位置
难度 : :star::star::star:
Python解法
temp = df['popularity']
df.drop(labels=['popularity'], axis=1,inplace = True)
df.insert(0, 'popularity', temp)
15
数据提取
题目 :提取 popularity 列最大值所在行
难度 : :star::star:
Python解法
df[df['popularity'] == df['popularity'].max()]
16
数据查看
题目 :查看最后5行数据
难度 : :star:
Python解法
df.tail()
17
数据修改
题目 :删除最后一行数据
难度 : :star:
Python解法
df = df.drop(labels=df.shape[0]-1)
18
数据修改
题目 :添加一行数据[ 'Perl' , 6.6]
难度 : :star::star:
Python解法
row = {'grammer':'Perl','popularity':6.6}
df = df.append(row,ignore_index=True)
19
数据整理
题目 :对数据按照 "popularity" 列值的大小进行排序
难度 : :star::star:
Python解法
df.sort_values("popularity",inplace=True)
20
字符统计
题目 :统计 grammer 列每个字符串的长度
难度 : :star::star::star:
Python解法
df['grammer'] = df['grammer'].fillna('R')
df['len_str'] = df['grammer'].map(lambda x: len(x))

第二期:数据处理基础
21
数据读取
题目 :读取本地EXCEL数据
难度 : :star:
Python解法
import pandas as pd
import numpy as np
df = pd.read_excel(r'C:\Users\chenx\Documents\Data Analysis\pandas120.xlsx')
21—50部分习题与该数据相关
22
数据查看
题目 :查看df数据前5行
难度 : :star:
期望输出
Python解法
df.head()
23
数据计算
题目 :将 salary 列数据转换为最大值与最小值的平均值
难度 : :star::star::star::star:
期望输出
Python解法
# 方法一:apply + 自定义函数
def func(df):
lst = df['salary'].split('-')
smin = int(lst[0].strip('k'))
smax = int(lst[1].strip('k'))
df['salary'] = int((smin + smax) / 2 * 1000)
return df
df = df.apply(func,axis=1)
# 方法二:iterrows + 正则
import re
for index,row in df.iterrows():
nums = re.findall('\d+',row[2])
df.iloc[index,2] = int(eval(f'({nums[0]} + {nums[1]}) / 2 * 1000'))
24
数据分组
题目 :将数据根据学历进行分组并计算平均薪资
难度 : :star::star::star:
期望输出
educationsalary
不限 19600.000000
大专 10000.000000
本科 19361.344538
硕士 20642.857143
Python解法
df.groupby('education').mean()
25
时间转换
题目 :将 createTime 列时间转换为 月-日
难度 : :star::star::star:
期望输出
Python解法
for index,row in df.iterrows():
df.iloc[index,0] = df.iloc[index,0].to_pydatetime().strftime("%m-%d")
26
数据查看
题目 :查看索引、数据类型和内存信息
难度 : :star:
期望输出
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 135 entries, 0 to 134
Data columns (total 4 columns):
createTime 135 non-null object
education 135 non-null object
salary 135 non-null int64
categories 135 non-null category
dtypes: category(1), int64(1), object(2)
memory usage: 3.5+ KB
Python解法
df.info()
27
数据查看
题目 :查看数值型列的汇总统计
难度 : :star:
Python解法
df.describe()
R解法
summary(df)
28
数据整理
题目 :新增一列根据salary将数据分为三组
难度 : :star::star::star::star:
输入
期望输出
Python解法
bins = [0,5000, 20000, 50000]
group_names = ['低', '中', '高']
df['categories'] = pd.cut(df['salary'], bins, labels=group_names)
29
数据整理
题目 :按照 salary 列对数据降序排列
难度 : :star::star:
Python解法
df.sort_values('salary', ascending=False)
3 0
数据提取
题目 :取出第33行数据
难度 : :star::star:
Python解法
df.iloc[32]
31
数据计算
题目 :计算 salary 列的中位数
难度 : :star::star:
Python解法
np.median(df['salary'])
# 17500.0
32
数据可视化
题目 :绘制薪资水平频率分布直方图
难度 : :star::star::star:
期望输出
Python解法
# Jupyter运行matplotlib成像需要运行魔术命令
%matplotlib inline
plt.rcParams['font.sans-serif'] = ['SimHei'] # 解决中文乱码
plt.rcParams['axes.unicode_minus'] = False # 解决符号问题
import matplotlib.pyplot as plt
plt.hist(df.salary)
# 也可以用原生pandas方法绘图
df.salary.plot(kind='hist')
33
数据可视化
题目 :绘制薪资水平密度曲线
难度 : :star::star::star:
期望输出
Python解法
df.salary.plot(kind='kde',xlim = (0,70000))
34
数据删除
题目 :删除最后一列 categories
难度 : :star:
Python解法
del df['categories']
# 等价于
df.drop(columns=['categories'], inplace=True)
35
数据处理
题目 :将df的第一列与第二列合并为新的一列
难度 : :star::star:
Python解法
df['test'] = df['education'] + df['createTime']
36
数据处理
题目 : 将education列 与salary列合并为新的一列
难度 : :star::star::star:
备注: sala ry为int类型,操作与35题有所不同
Python解法
df["test1"] = df["salary"].map(str) + df['education']
37
数据计算
题目 :计算salary最大值与最小值之差
难度 : :star::star::star:
Python解法
df[['salary']].apply(lambda x: x.max() - x.min())
# salary 41500
# dtype: int64
38
数据处理
题目 :将第一行与最后一行拼接
难度 : :star::star:
Python解法
pd.concat([df[1:2], df[-1:]])
39
数据处理
题目 :将第8行数据添加至末尾
难度 : :star::star:
Python解法
df.append(df.iloc[7])
40
数据查看
题目 :查看每列的数据类型
难度 : :star:
期望结果
createTime object
education object
salary int64
test object
test1 object
dtype: object
Python解法
df.dtypes
# createTime object
# education object
# salary int64
# test object
# test1 object
# dtype: object
41
数据处理
题目 :将 c r e a t e T i m e 列设置为索引
难度 : :star::star:
Python解法
df.set_index("createTime")
42
数据创建
题目 :生成一个和df长度相同的随机数dataframe
难度 : :star::star:
Python解法
df1 = pd.DataFrame(pd.Series(np.random.randint(1, 10, 135)))
43
数据处理
题目 :将上一题生成的dataframe与df合并
难度 : :star::star:
Python解法
df= pd.concat([df,df1],axis=1)
44
数据计算
题目 :生成新的一列 new 为 salary 列减去之前生成随机数列
难度 : :star::star:
Python解法
df["new"] = df["salary"] - df[0]
45
缺失值处理
题目 :检查数据中是否含有任何缺失值
难度 : :star::star::star:
Python解法
df.isnull().values.any()
# False
46
数据转换
题目 :将 salary 列类型转换为浮点数
难度 : :star::star::star:
Python解法
df['salary'].astype(np.float64)
47
数据计算
题目 :计算 salary 大于10000的次数
难度 : :star::star:
Python解法
len(df[df['salary'] > 10000])
# 119
48
数据统计
题目 :查看每种学历出现的次数
难度 : :star::star::star:
期望输出
本科 119
硕士 7
不限 5
大专 4
Name: education, dtype: int64
Python解法
df.education.value_counts()
49
数据查看
题目 :查看 education 列共有几种学历
难度 : :star::star:
Python解法
df['education'].nunique()
# 4
50
数据提取
题目 :提取 salary 与 new 列的和大于60000的最后3行
难度 : :star::star::star::star:
期望输出
Python解法
rowsums = df[['salary','new']].apply(np.sum, axis=1)
res = df.iloc[np.where(rowsums > 60000)[0][-3:], :]
51
数据读取
题目 :使用绝对路径读取本地Excel数据
难度 : :star:
Python解法
import pandas as pd
import numpy as np
df = pd.read_excel(r'C:\Users\chenx\Documents\Data Analysis\Pandas51-80.xls')
备注
请将答案中路径替换为自己机器存储数据的绝对路径,51—80相关习题与该数据有关
52
数据查看
题目 :查看数据前三行
难度 : :star:
期望结果
Python解法
df.head(3)
53
缺失值处理
题目 :查看每列数据缺失值情况
难度 : :star: :star:
期望结果
代码 1
简称 2
日期 2
前收盘价(元) 2
开盘价(元) 2
最高价(元) 2
最低价(元) 2
收盘价(元) 2
成交量(股) 2
成交金额(元) 2
.................
Python解法
df.isnull().sum()
54
缺失值处理
题目 :提取日期列含有空值的行
难度 : :star: :star:
期望结果
Python解法
df[df['日期'].isnull()]
55
缺失值处理
题目 :输出每列缺失值具体行数
难度 : :star: :star: :star:
期望结果
列名:"代码", 第[327]行位置有缺失值
列名:"简称", 第[327, 328]行位置有缺失值
列名:"日期", 第[327, 328]行位置有缺失值
列名:"前收盘价(元)", 第[327, 328]行位置有缺失值
列名:"开盘价(元)", 第[327, 328]行位置有缺失值
列名:"最高价(元)", 第[327, 328]行位置有缺失值
列名:"最低价(元)", 第[327, 328]行位置有缺失值
列名:"收盘价(元)", 第[327, 328]行位置有缺失值
................
Python解法
for i in df.columns:
if df[i].count() != len(df):
row = df[i][df[i].isnull().values].index.tolist()
print('列名:"{}", 第{}行位置有缺失值'.format(i,row))
56
缺失值处理
题目 :删除所有存在缺失值的行
难度 : :star: :star:
Python解法
df.dropna(axis=0, how='any', inplace=True)
备注
axis:0-行操作(默认),1-列操作
how:any-只要有空值就删除(默认),all-全部为空值才删除
inplace:False-返回新的数据集(默认),True-在原数据集上操作
57
数据可视化
题目 :绘制收盘价的折线图
难度 : :star: :star:
期望结果
Python解法
# Jupyter运行matplotlib
%matplotlib inline
df['收盘价(元)'].plot()
# 等价于
import matplotlib.pyplot as plt
plt.plot(df['收盘价(元)'])
58
数据可视化
题目 :同时绘制开盘价与收盘价
难度 : :star: :star: :star:
期望结果
Python解法
plt.rcParams['font.sans-serif'] = ['SimHei'] # 解决中文乱码
plt.rcParams['axes.unicode_minus'] = False # 解决符号问题
df[['收盘价(元)','开盘价(元)']].plot()
59
数据可视化
题目 :绘制涨跌幅的直方图
难度 : :star: :star:
期望结果
Python解法
plt.hist(df['涨跌幅(%)'])
# 等价于
df['涨跌幅(%)'].hist()
60
数据可视化
题目 :让直方图更细致
难度 : :star: :star:
期望结果
Python解法
df['涨跌幅(%)'].hist(bins = 30)
61
数据创建
题目 : 以data的列名创建一个dataframe
难度 : :star: :star:
Python解法
temp = pd.DataFrame(columns = df.columns.to_list())
62
异常值处理
题目 : 打印所有换手率不是数字的行
难度 : :star: :star: :star:
期望结果
Python解法
for index,row in df.iterrows():
if type(row[13]) != float:
temp = temp.append(df.loc[index])
63
异常值处理
题目 : 打印所有换手率为--的行
难度 : :star: :star: :star:
Python解法
df[df['换手率(%)'] == '--']
备注
通过上一题我们发现换手率的异常值只有--
64
数据处理
题目 : 重置data的行号
难度 : :star:
Python解法
df = df.reset_index(drop=True)
备注
有时我们修改数据会导致索引混乱
65
异常值处理
题目 : 删除所有换手率为非数字的行
难度 : :star: :star: :star:
Python解法
lst = []
for index,row in df.iterrows():
if type(row[13]) != float:
lst.append(index)
df.drop(labels=lst,inplace=True)
66
数据可视化
题目 : 绘制 换手率的密度曲线
难度 : :star: :star: :star:
期望结果
Python解法
df['换手率(%)'].plot(kind='kde',xlim=(0,0.6))
67
数据计算
题目 : 计算前一天与后一天收盘价的差值
难度 : :star: :star:
Python解法
df['收盘价(元)'].diff()
68
数据计算
题目 : 计算前一天与后一天收盘价变化率
难度 : :star: :star:
Python解法
data['收盘价(元)'].pct_change()
69
数据处理
题目 : 设置日期为索引
难度 : :star:
Python解法
df.set_index('日期')
70
指标计算
题目 : 以5个数据作为一个数据滑动窗口,在这个5个数据上取均值(收盘价)
难度 : :star: :star: :star:
Python解法
df['收盘价(元)'].rolling(5).mean()
71
指标计算
题目: 以5个数据作为一个数据滑动窗口,计算这五个数据总和(收盘价)
难度 : :star: :star: :star:
Python解法
df['收盘价(元)'].rolling(5).sum()
72
数据可视化
题目: 将收盘价5日均线、20日均线与原始数据绘制在同一个图上
难度 : :star: :star: :star:
期望结果
Python解法
df['收盘价(元)'].plot()
df['收盘价(元)'].rolling(5).mean().plot()
df['收盘价(元)'].rolling(20).mean().plot()
73
数据重采样
题目: 按周为采样规则,取一周收盘价最大值
难度 : :star: :star: :star:
Python解法
df = df.set_index('日期')
df['收盘价(元)'].resample('W').max()
74
数据可视化
题目: 绘制重采样数据与原始数据
难度 : :star: :star: :star:
期望结果
Python解法
df['收盘价(元)'].plot()
df['收盘价(元)'].resample('7D').max().plot()
75
数据处理
题目 :将数据往后移动5天
难度 : :star: :star:
Python解法
df.shift(5)
76
数据处理
题目 :将数据向前移动5天
难度 : :star: :star:
Python解法
df.shift(-5)
77
数据计算
题目 :使用expending函数计算开盘价的移动窗口均值
难度 : :star: :star:
Python解法
df['开盘价(元)'].expanding(min_periods=1).mean()
78
数据可视化
题目 :绘制上一题的移动均值与原始数据折线图
难度 : :star: :star: :star:
期望结果
Python解法
df['expanding Open mean']=df['开盘价(元)'].expanding(min_periods=1).mean()
df[['开盘价(元)', 'expanding Open mean']].plot(figsize=(16, 6))
79
数据计算
题目 :计算布林指标
难度 : :star: :star: :star: :star:
Python解法
df['former 30 days rolling Close mean']=df['收盘价(元)'].rolling(20).mean()
df['upper bound']=df['former 30 days rolling Close mean']+2*df['收盘价(元)'].rolling(20).std()
df['lower bound']=df['former 30 days rolling Close mean']-2*df['收盘价(元)'].rolling(20).std()
80
数据可视化
题目 :计算 布林线 并绘制
难度 : :star: :star: :star:
期望结果
Python解法
df[['收盘价(元)', 'former 30 days rolling Close mean','upper bound','lower bound' ]].plot(figsize=(16, 6))
81
数据查看
题目 :导入并查看pandas与numpy版本
难度 : :star:
Python解法
import pandas as pd
import numpy as np
print(np.__version__)
# 1.16.5
print(pd.__version__)
# 0.25.1
82
数据创建
题目 :从NumPy数组创建DataFrame
难度 : :star:
备注
使用numpy生成20个0-100 随机数
Python解法
tem = np.random.randint(1,100,20)
df1 = pd.DataFrame(tem)
83
数据创建
题目 :从NumPy数组创建DataFrame
难度 : :star:
备注
使用numpy生成20个0-100 固定步长 的数
Python解法
tem = np.arange(0,100,5)
df2 = pd.DataFrame(tem)
84
数据创建
题目 :从NumPy数组创建DataFrame
难度 : :star:
备注
使用numpy生成20个 指定分布(如标准正态分布) 的数
Python解法
tem = np.random.normal(0, 1, 20)
df3 = pd.DataFrame(tem)
85
数据创建
题目 :将df1,df2,df3按照行合并为新DataFrame
难度 : :star::star:
Python解法
df = pd.concat([df1,df2,df3],axis=0,ignore_index=True)
86
数据创建
题目 :将df1,df2,df3按照列合并为新DataFrame
难度 : :star::star:
期望结果
0 1 2
0 95 0 0.022492
1 22 5 -1.209494
2 3 10 0.876127
3 21 15 -0.162149
4 51 20 -0.815424
5 30 25 -0.303792
...............
Python解法
df = pd.concat([df1,df2,df3],axis=1,ignore_index=True)
87
数据查看
题目 :查看df所有数据的最小值、25%分位数、中位数、75%分位数、最大值
难度 : :star::star:
Python解法
np.percentile(df, q=[0, 25, 50, 75, 100])
88
数据修改
题目 :修改列名为col1,col2,col3
难度 : :star:
Python解法
df.columns = ['col1','col2','col3']
89
数据提取
题目 :提取第一列中不在第二列出现的数字
难度 : :star::star::star:
Python解法
df['col1'][~df['col1'].isin(df['col2'])]
90
数据提取
题目 :提取第一列和第二列出现频率最高的三个数字
难度 : :star::star::star:
Python解法
temp = df['col1'].append(df['col2'])
temp.value_counts()[:3]
91
数据提取
题目 :提取第一列中可以整除5的数字位置
难度 : :star::star::star:
Python解法
np.argwhere(df['col1'] % 5==0)
92
数据计算
题目 :计算第一列数字前一个与后一个的差值
难度 : :star::star:
Python解法
df['col1'].diff().tolist()
93
数据处理
题目 :将col1,col2,clo3三列顺序颠倒
难度 : :star::star:
Python解法
df.iloc[:, ::-1]
94
数据提取
题目 :提取第一列位置在1,10,15的数字
难度 : :star::star:
Python解法
df['col1'].take([1,10,15])
# 等价于
df.iloc[[1,10,15],0]
95
数据查找
题目 :查找第一列的局部最大值位置
难度 : :star::star::star::star:
备注
即比它前一个与后一个数字的都大的数字
Python解法
res = np.diff(np.sign(np.diff(df['col1'])))
np.where(res== -2)[0] + 1
# array([ 2, 4, 7, 9, 12, 15], dtype=int64)
96
数据计算
题目 :按行计算df的每一行均值
难度 : :star::star:
Python解法
df[['col1','col2','col3']].mean(axis=1)
97
数据计算
题目 :对第二列计算移动平均值
难度 : :star::star::star:
备注
每次移动三个位置,不可以使用自定义函数
Python解法
np.convolve(df['col2'], np.ones(3)/3, mode='valid')
98
数据修改
题目 :将数据按照第三列值的大小升序排列
难度 : :star::star:
Python解法
df.sort_values("col3",inplace=True)
99
数据修改
题目 :将第一列大于50的数字修改为'高'
难度 : :star::star:
Python解法
df.col1[df['col1'] > 50] = '高'
100
数据计算
题目 :计算第一列与第二列之间的欧式距离
难度 : :star::star::star:
备注
不可以使用自定义函数
Python解法
np.linalg.norm(df['col1']-df['col2'])
# 194.29873905921264
101
数据读取
题目 :从CSV文件中读取指定数据
难度 : :star::star:
备注
从数据1中的前10行中读取positionName, salary两列
Python解法
df1 = pd.read_csv(r'C:\Users\chenx\Documents\Data Analysis\数据1.csv',encoding='gbk', usecols=['positionName', 'salary'],nrows = 10)
102
数据读取
题目 :从CSV文件中读取指定数据
难度 : :star::star:
备注
从数据2中读取数据并在读取数据时将薪资大于10000的为改为高
Python解法
df2 = pd.read_csv(r'C:\Users\chenx\Documents\Data Analysis\数据2.csv',
converters={'薪资水平': lambda x: '高' if float(x) > 10000 else '低'} )
103
数据计算
题目 :从dataframe提取数据
难度 : :star::star::star:
备注
从上一题数据中,对薪资水平列每隔20行进行一次抽样
期望结果
Python解法
df2.iloc[::20, :][['薪资水平']]
104
数据处理
题目 :将数据取消使用科学计数法
难度 : :star::star:
输入
df = pd.DataFrame(np.random.random(10)**10, columns=['data'])
期望结果
Python解法
df = pd.DataFrame(np.random.random(10)**10, columns=['data'])
df.round(3)
105
数据处理
题目 :将上一题的数据转换为百分数
难度 : :star::star::star:
期望结果
Python解法
df.style.format({'data': '{0:.2%}'.format})
106
数据查找
题目 :查找上一题数据中第3大值的行号
难度 : :star::star::star:
Python解法
df['data'].argsort()[len(df)-3]
107
数据处理
题目 :反转df的行
难度 : :star::star:
Python解法
df.iloc[::-1, :]
108
数据重塑
题目 :按照多列对数据进行合并
难度 : :star::star:
输 入
df1= pd.DataFrame({'key1': ['K0', 'K0', 'K1', 'K2'],
'key2': ['K0', 'K1', 'K0', 'K1'],
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3']})
df2= pd.DataFrame({'key1': ['K0', 'K1', 'K1', 'K2'],
'key2': ['K0', 'K0', 'K0', 'K0'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
Python解法
pd.merge(df1, df2, on=['key1', 'key2'])
109
数据重塑
题目 :按照多列对数据进行合并
难度 : :star::star:
备注
只保存df1的数据
Python解法
pd.merge(df1, df2, how='left', on=['key1', 'key2'])
R语言解法
left_join(df1,df2,by = c('key1','key2'))
110
数据处理
题目 :再次读取数据1并显示所有的列
难度 : :star::star:
备注
数据中由于列数较多中间列不显示
Python解法
df = pd.read_csv(r'C:\Users\chenx\Documents\Data Analysis\数据1.csv',encoding='gbk')
pd.set_option("display.max.columns", None)
111
数据查找
题目 :查找secondType与thirdType值相等的行号
难度 : :star::star:
Python解法
np.where(df.secondType == df.thirdType)
112
数据查找
题目 :查找薪资大于平均薪资的第三个数据
难度 : :star::star::star:
Python解法
np.argwhere(df['salary'] > df['salary'].mean())[2]
# array([5], dtype=int64)
113
数据计算
题目 :将上一题数据的salary列开根号
难度 : :star::star:
Python解法
df[['salary']].apply(np.sqrt)
114
数据处理
题目 :将上一题数据的linestaion列按_拆分
难度 : :star::star:
Python解法
df['split'] = df['linestaion'].str.split('_')
115
数据查看
题目 :查看上一题数据中一共有多少列
难度 : :star:
Python解法
df.shape[1]
# 54
116
数据提取
题目 :提取industryField列以'数据'开头的行
难度 : :star::star:
Python解法
df[df['industryField'].str.startswith('数据')]
117
数据计算
题目 : 以salary score 和 positionID制作数据透视
难度 : :star::star::star:
Python解法
pd.pivot_table(df,values=["salary","score"],index="positionId")
118
数据计算
题目 :同时对salary、score两列进行计算
难度 : :star::star::star:
Python解法
df[["salary","score"]].agg([np.sum,np.mean,np.min])
119
数据计算
题目 :对不同列执行不同的计算
难度 : :star::star::star:
备注
对salary求平均,对score列求和
Python解法
df.agg({"salary":np.sum,"score":np.mean})
120
数据计算
题目 :计算并提取平均薪资最高的区
难度 : :star::star::star::star:
Python解法
df[['district','salary']].groupby(by='district').mean().sort_values(
'salary',ascending=False).head(1)
往期文章
先有收获,再点在看!
后台回复 pandas120 即可下载本教程课件代码
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK