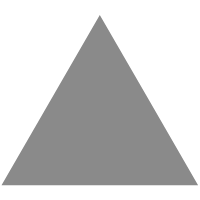
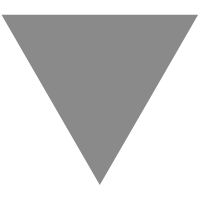
3 Ways To Theme React Components
source link: https://blog.bitsrc.io/3-ways-to-theme-react-components-9cfa631351e9
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
My favorite ways to theme React components.
In this post, I give a quick introduction to three of my top favorite ways to theming React components. When theming React components, we should take into consideration performance, ease-of-use (or developer experience), flexibility and “mobility” or how easy it is to reuse it in other projects.
For example, it’s quite common to see front-end teams publish their UI components to component hubs like Bit.dev . Unfortunately, publishing and documenting your components in component hubs is not enough for them to be easily reusable. A lot of consideration has to be taken into account — theming incorrectly can be detrimental to how much these components are actually being reused (and that, in turn, affects shipping time and UI consistency).

1. CSS Variables
CSS Variables or CSS custom properties are a way to set values in variables and refer to these variables, all with plain vanilla CSS.
To define a CSS variable globally , we commonly use the :root
pseudo-selector (keep in mind, variables can be defined under any other CSS selector, but that means they will only be available to that selected group of elements).
Read more about “scoping” CSS variables here:
Theming React Components with CSS Variables
Two ways to theme React components using CSS custom properties.
blog.bitsrc.io
:root { --css-variable: value; }
The css-variable
is the name of the CSS variable and value
is its — you guessed it — value.
To define a CSS variable we append the double --
followed by the variable name.
:root { --main-color: orangered; }
Here, we set the main color of our app to be “orangered”. The main-color
variable can be used as a value, anywhere in our style codes.
To use a CSS variable, we use a var(--css-variable-name)
syntax. We pass the CSS variable name plus the --
to the var
function, this will retrieve the value of the CSS variable --css-variable-name
and set it to the property name.
:root { --main-color: #1da1f2; --background-color: #d7d7db; }button { background-color: var(---main-color); ... }header { background-color: var(--main-color); ... }body { background-color: var(--background-color); ... }
We have the variables --main-color
and --background-color
set to the color #1da1f2
(a sky-blueish color) and #d7d7db
(ashen color), respectively. The background-color
of the button and header will resolve to the value of the --main-color, #1da1f2
and the background color of the body will resolve to the value of --background-color, #d7d7db
.
On execution, the style code would resolve to this:
button { background-color: #1da1f2; ... }header { background-color: #1da1f2; ... }body { background-color: #d7d7db; ... }
We can modify/update the CSS variables from JavaScript. We can get the value of CSS variables in a webpage by using the methods:
window .getComputedStyle(document.documentElement) .getPropertyValue(--css-variable-name)
and
document.documentElement.style.getPropertyValue(--css-variable-name)
Once we pass in the CSS variable name, the value is returned.
We can set the value of the CSS variable by using the method:
document .documentElement .style .setProperty(--css-variable-name, value)
The setProperty
needs the CSS variable name and the value to set it to as params. It then replaces the CSS variable name passed with the value
.
document.documentElement.style.getPropertyValue("--main-color")-> reddocument.documentElement.style.setProperty("--main-color", "blue")document.documentElement.style.getPropertyValue("--main-color")-> blue
We have seen what CSS Variable is and how we can use it to build a re-usable style code.
Now, let’s see how we can use it to theme React components.
Let’s say we have a simple React app with an App component:
:root { --main-color: #08e608; }#root { width: 100%; display: flex; align-items: center; justify-content: center; }body { color: var(--main-color); }.main { width: 400px; border: 2px solid var(--main-color); padding: 3px; }button { padding: 6px 12px; background: var(--main-color); color: white; border: 1px solid transparent; border-radius: 4px; font-size: 16px; margin: 2px; }
The CSS variable --main-color
holds #08e608 (“limegreen”) color. This will set the body color to #08e608, the button to #08e608 and the .main
div borders to #08e608 color.
class App extends React.Component { setTheme(color) { if (color === "default") color = "#08e608"; document .documentElement .style .setProperty("--main-color", color); } render() { return ( <div className="main"> <button onClick={() => this.setTheme("red")}>Set Theme(Red)</button> <button onClick={() => this.setTheme("orangered")}>Set Theme(Orange-Red)</button> <button onClick={() => this.setTheme("default")}>Reset Theme Default</button> </div> ) } }
We have the App component here with three buttons. Each button when clicked calls the setTheme with a color. The setTheme method uses the setProperty to set the color of the --main-color
.
The Set Theme(Red)
button calls the setTheme with text "red", this sets the theme of the App component to "red".
The Set Theme(Orange-Red)
button calls the setTheme with text "orangered", this sets the theme of the App component to "orangered".
The Reset Theme Default
sets the component theme to the default color #08e608.
Serve the app npm run start
and see the outcome:

Set the theme to “orangered” color

Set the theme back to the default theme:

Let’s see another example, a Counter application with theme styling. You can play with the example in Bit’s playground here:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK