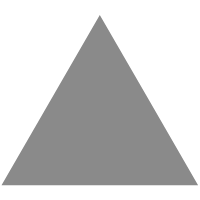
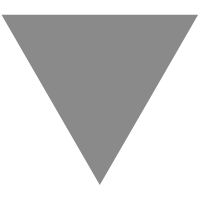
The 3 Secret Weapons That Changed My Python Editor Forever
source link: https://towardsdatascience.com/the-3-secret-weapons-that-changed-my-python-editor-forever-c99f7b2e0084?gi=65a4cf3e458a
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
3 Insane Secret Weapons for Python
I don’t know how I lived without them
Apr 20 ·6min read
I’ve been writing Python for over 5 years and my toolset generally has been getting smaller, not bigger. A lot of tools just aren’t necessary or useful, and some of them you’ll simply outgrow.
These are three I’ve been stuck on for a long time, and unlike the rest, I just keep using them more and more.
Secret Weapon #1: Write Faster and Google Less with Kite
Most code editors have an autocomplete feature that looks something like this:
…which uses language (and sometimes library) documentation to suggest things like function names and parameters.
Sure that’s okay, but what if your editor could surf years of GitHub data and autocomplete not just function names, but entire lines of code.
This is just the first of three reasons you should use Kite.
Reason 1: Line-of-Code Completions
Kite looks at your codebase and variables, frequently used parameter names online, documentation, and then makes super contextual recommendations like this:
The above example shows how Kite can predict which variables you’ll use where even they’re named generically (like b
) or using common names (like x
or y
).
…we’ve spent around 50 engineer-years semantically indexing all the code on Github, building statistical type inference, and rich statistical models that use this semantic information in a very deep way. — Adam Smith , Founder/CEO at Kite
Here’s a real-time demo video , or if you’d like to, go play in the sandbox .
Reason 2: Copilot for Documentation
If you’ve never heard the term “ RTFM ” then you probably haven’t worked around baby-boomer developers before.
I’m not sure if that’s good or bad, but regardless, you should always read the documentation before bugging a senior developer or even looking at Stack Overflow answers.
Kite Copilot makes documentation stupid-easy. It runs alongside your editor and in real-time shows the docs for any object/function/etc that you highlight with your cursor.
Dear senior developer at my first job: I’m sorry. Now I truly have no excuse not to look for answers in the docs first. :wink:
Reason 3: Runs Locally, Privately
On top of all that it’s built to run locally so you get incredibly fast recommendations, it works offline, and your code is never sent to the cloud.
This is incredibly important for people with poor internet connections and folks who work in closed-source codebases.
Result
I’ve been using Kite for years, and it just keeps getting better. With over $17M in investment, this company isn’t going anywhere and the tool, for some stupid reason, is completely free .
All you have to do is download the Kite plugin for your editor, or download the copilot and it can install plugins for you. Go get it !
Secret Weapon #2: Stabilize Your Code with Mypy
Python is dynamically typed , an oversimplified explanation would be that you can make any variable be any data type (string, integer, etc) at any time.
// These two variable types are declared the exact same way // Python figures out the data type on it's own, dynamically// string var_name = "string here"// integer var_name = 1234
The opposite is languages that are statically typed , where variables must have one specific data type, and always adhere to it.
// Many languages require the data type to be declared too// string str var_name = "string here"// integer int var_name = 1234
Pros/Cons of Dynamic Typing
The advantage of dynamic typing is that you can be lazy when you’re writing and it can reduce code clutter.
The disadvantages are numerous and big though:
- You generally run into errors later in the development cycle
- Code performs worse since Python is constantly figuring out types
- Functions are less stable since their inputs and outputs can change datatypes without warning
- Handing off code is much more volatile since others might not know what datatypes your variables are or could become
Static Typing in Python
Enter Mypy. A free Python module that lets you use static typing inside of Python.
After you pip install mypy
, here’s just one example of how it’s used:
// Declaring a function using normal dynamic typing, without mypy def iter_primes(): # code here// Declaring the same function with mypy static typing from typing import Iteratordef iter_primes() -> Iterator[int]: # code here
With the mypy example, we’re specifying that the function returns an Iterator of Integers. This simple change makes the function more future-proof by enforcing a consistent output.
Other developers only have to look at the declaration to see what data type the output will be, and unlike just using documentation, your code will error if that declaration is disobeyed.
This is a super simple example taken from the examples here , go check them out if it’s still not making sense.
Result
It’s hard to list all the ways that static typing can save you future pain, but the mypy docs have a great FAQ with more pros and cons.
If you’re working in a production codebase where stability is king, definitely give mypy a try .
Secret Weapon #3: Find Errors Faster and Write Simpler Functions with Sonarlint
Nowadays every editor has some type of error checking or “linter” built-in. It looks at the code, typically without running it, and tries to guess what might go wrong. This is called Static Code Analysis.
Dynamic Code Analysis actually attempts to run/compile parts of your code to see if it’s working properly, but it does this in the background automatically. Instead of guessing, it actually knows if it will work and what the exact errors would be.
SonarLint is Dynamic Code Analysis at its best, plus more. These features are why I love it:
Commented or Uncalled Code
I’m guilty of leaving print statements, commented out code, and unused functions lying all over my codebase. This will warn me about it, making it hard to forget, and tell me where it is, making it easy to find.
Security Risks
A huge database of constantly updated security risks is thrown at your codebase in real-time, warning you of any known vulnerabilities that you’re exposed to.
Security risks are very niche and impossible to memorize, so everyone should use something to track these. SonarLint is a great place to start.
Code That’s Never Executed
Slightly different from uncalled code, this will warn me if I’ve created any evaluations that have a result that is impossible to reach. These are tricky to spot and can lead to hours of debugging, so it’s one of my favorite warnings.
Here’s an example:
a = Noneif a == None or not a or a: this_will_always_get_called() else: // sonarlint will warn you about this line never being executed this_will_never_get_called()
Cognitive Complexity
This is a fascinating topic that I could write an entire post about, in fact, there’s a whole whitepaper on it.
The simple explanation is that they’ve created a math formula that can score how difficult code is to read/understand.
It’s not only incredibly useful, but it’s also easy to follow. Every time SonarLint has asked me to “reduce cognitive complexity” it comes with a simple explanation of the rule I broke, like “too many nested if statements”.
Result
I find this more useful than basic blocking and linting practices, and I’m convinced it’s making me write human-friendlier code. Which is Pythonic, by the way !
SonarLint is free, so there’s no reason not to grab it now and get it attached to your editor.
Conclusion
If you skipped here, just a quick warning that you might not be able to use these tools properly unless you have a basic understanding of the features.
Many of them are invisible or inside a menu, so be sure to glance over the ones I pointed out above or the overviews on each website first.
I hope these tools serve you well, I’ve become quite attached to them myself. I’m sure I’ve missed out on some other incredible resources though, so be sure to share what you can’t live without in the comments.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK