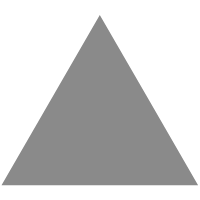
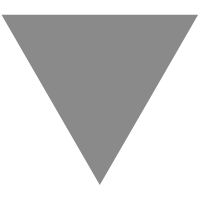
Recreate the Tweet Creation Modal with Tailwind CSS
source link: https://scotch.io/tutorials/recreate-the-tweet-creation-modal-with-tailwind-css
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Tailwind CSS is an awesome tool that changes the way we write our HTML and CSS. Tailwind is a "utility-first CSS framework" that I initially wasn't sure about. Our HTML gets pretty busy when using Tailwind, but I've found it's not that bad of a tradeoff.
Table of Contents
- Create the Modal Header
- Create the Modal Body
- Adding a Profile Image
- Create the Modal Footer
The more I have used Tailwind, the more I have liked it. It lets me design my UI quicker than ever before. People say that CSS frameworks will make you not know the underlying CSS. With Tailwind, I believe you need to know your CSS even better to be able to use the utility classes.
Let's recreate something with Tailwind so that we can practice.
I've found some things around the web to rebuild with Tailwind. For this article, we'll build Twitter's tweet creation modal. Let's get started!
We'll do all of our work inside of CodePen . It's a great place to experiment with HTML/CSS/JavaScript projects.
Fork this CodePen to start: https://codepen.io/chrisoncode/pen/mdyoGar
Essential Reading : Learn React from Scratch! (2020 Edition)The Final CodePen
If you want to see what our final project will look like, here you go:
We want our modal to sit in the middle of our screen. For this, we'll need a container that is set to the full height of our browser screen. Then we'll use CSS flexbox to vertically and horizontally center.
Write the following in your HTML section of CodePen.
<div class="h-screen flex items-center justify-center"> </div>
Let's set a background to match Twitter's dark mode background. With Tailwind, we try to write as little custom CSS as possible. This is one of the times that we will write custom CSS because Tailwind doesn't have this color that we want in its pre-built classes. Here's the rundown:
h-screen flex items-center justify-center
In the CSS section of our CodePen, add the following:
body { background: #15202b; }
Now that we have the foundation, everything we put inside of that main div will be centered in our browser.
<!-- tweet creation modal --> <div class="modal rounded-lg shadow-lg bg-blue-900"> <!-- header --> <!-- body --> <!-- footer --> </div>
We have given it rounded borders, a box shadow, and a background of blue. Tailwind provides colors from 100 to 900 with 900 being the darkest.
I've also given a custom class ( modal
) so that we can add one custom style here. Again, we usually want to avoid using custom styles. In this case, we want to set a min-width , but Tailwind only allows for widths of 0 or 100%.
Add this to our CSS:
.modal { min-width: 60%; }
That will be all the custom CSS that we need! All the rest of the work will be Tailwind classes.
Create the Modal Header
Let's move forward to make the modal header. It's just a section that has an "X" to close the modal. We need a border and text colors for our header.
<!-- header --> <div class="border-b border-blue-800 text-blue-400"> <div class="p-4">X</div> </div>
Create the Modal Body
Next up is the modal body. This will require an <image>
and a <textarea>
. We want the image on the left; there a few ways to accomplish this:
- Make the image and textarea positioned next to each other with flexbox
- Make the image positioned absolutely
We'll go for the positioned absolutely option. This may be easier to align with the footer since it looks like the spacing is the same from the body to the footer in Twitter's modal.
Let's start our body section:
<!-- body --> <div class="p-4 pl-20 relative"> </div>
We've added a padding to the left of the body. This is where we'll position our image. We've also added padding to the top for some spacing. Lastly, we have added the relative
class so that we can position a child image absolutely.
Adding a Profile Image
Let's add the image now:
<!-- body --> <div class="p-4 pl-20 relative"> <img class="absolute top-0 left-0 mt-4 ml-4 rounded-full" src="http://placekitten.com/g/50/50"> </div>
Here are the classes we've used:
absolute top-0 left-0 mt-4 ml-4
Feel free to add in your own image also. If your image is larger, you may need to constrain its size with one of the width classes .
Adding a Textarea
We'll add the textarea and some styles:
<!-- body --> <div class="p-4 pl-20 relative"> <img class="absolute top-0 left-0 mt-4 ml-4 rounded-full" src="http://placekitten.com/g/50/50"> <textarea class="bg-transparent w-full pt-4 outline-none text-lg text-white" placeholder="What's happening?" rows="5"></textarea> </div>
Here's what we've done so far:
bg-transparent w-full pt-4 outline-none text-lg text-white
Last step is to create the footer! Notice that there is a left and ride side to our footer. We need to use flexbox to space them appropriately.
<!-- footer --> <div class="p-4 pl-20 flex justify-between"> <div class="flex items-center text-3xl"> left side </div> <div class="flex items-center"> right side </div> </div>
We now have a left and right side! The important thing to note here is justify-between
. It's flexbox's way of pushing things to the sides of a container.
Let's fill in the icons on the left side. We don't have an icon font installed, so we'll just use emojis! Update the left side to be:
<!-- footer --> <div class="p-4 pl-20 flex justify-between"> <div class="flex items-center text-3xl"> <a class="inline-block mr-2"></a> <a class="inline-block mr-2">拾</a> <a class="inline-block mr-2"></a> <a class="inline-block">珞</a> </div> <div class="flex items-center"> right side </div> </div>
Now update the right side to be:
<!-- footer --> <div class="p-4 pl-20 flex justify-between"> <div class="flex items-center text-3xl"> <a class="inline-block mr-2"></a> <a class="inline-block mr-2">拾</a> <a class="inline-block mr-2"></a> <a class="inline-block">珞</a> </div> <div class="flex items-center"> <button class="rounded-full bg-blue-500 py-2 px-4 text-blue-100">Tweet</button> </div> </div>
With that, we have our modal!
All in all, this was a fun exercise to put Tailwind into action. Check out the CodePen to see the final code:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK