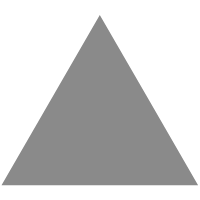
19
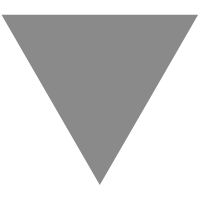
golang并发编程
source link: https://studygolang.com/articles/25980
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
go
main函数的执行本身就是一个协程,当使用 go
关键字的时候,就会创建一个新的协程
channel
channel 管道,用于在多个协程之间传递信号
无缓存管道
当对无缓冲通道写的时候,会一直阻塞等到某个协程对这个缓冲通道读
阻塞场景:
- 通道中无数据,但执行读通道。
- 通道中无数据,向通道写数据,但无协程读取。
综上,无缓存通道的读写必须同时存在,且读写分别在两个不同的协程
func main(){ ch := make(chan int) go func(ch chan int){ ch <-222 }(ch) println(<-ch) }
有缓冲管道
有缓存时可以向通道中写入数据后直接返回,缓存中有数据时可以从通道中读到数据直接返回,这时有缓存通道是不会阻塞的
阻塞场景:
- 通道的缓存无数据,但执行读通道。
- 通道的缓存已经占满,向通道写数据,但无协程读。
综上,有缓冲通道的读写必须在两个不同协程
func main() { ch := make(chan int, 1) //长度为1的缓冲管道也是有缓冲管道 ch <- 333 go func(ch chan int) { println(<-ch) }(ch) ch <- 333 }
sync.Mutex 和 sync.RwMutex
sync.Mutex 并发锁,一次只可以加载一个并发锁
sync.RwMutex 读写锁,一次可以加载多个读锁和一个写锁。当写锁存在时候,不能再加载读锁和写锁
sync.WaitGroup
阻塞等待所有任务完成之后再继续执行
WaitGroup在不方法中传递,需要传指针
func main() { var wg sync.WaitGroup ch := make(chan int, 1000) for i := 0; i < 1000; i++ { wg.Add(1) go doSomething(i, &wg, ch) } wg.Wait() fmt.Println("all done") for i := 0; i < 1000; i++ { dd := <-ch fmt.Println("from ch:"+strconv.Itoa(dd)) } } func doSomething(index int, wg *sync.WaitGroup, ch chan int) { defer wg.Done() fmt.Println("start done:" + strconv.Itoa(index)) //time.Sleep(20 * time.Millisecond) ch <- index }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK