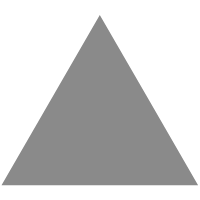
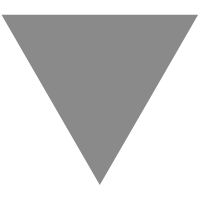
iOS定时器使用
source link: http://mp.weixin.qq.com/s?__biz=MzUyMDAxMjQ3Ng%3D%3D&%3Bmid=2247491713&%3Bidx=1&%3Bsn=fe18cdd5abab3bebf88273e9a35eb3d8
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
在开发中我们经常用到定时器,主要用于统计累加数据或者倒计时等。iOS中主要有三种定时器,包括NSTimer、CADisplayLink、GCD,其本质都是通过RunLoop来实现,但GCD通过其调度机制大大提高了性能。下面就分别对这三种计时器进行说明。
NSTimer
iOS中最基本的定时器,其通过RunLoop来实现,一般情况下较为准确,但当当前循环耗时操作较多时,会出现延迟问题。同时,也受所加入的RunLoop的RunLoopMode影响。
1
创建方法
NSTimer的初始化方式
NSTimer的初始化方式有几下几种:
invocation和selector两种调用方式,其实这两种区别不大,一般我们用selector方式较为方便。
+ (NSTimer *)timerWithTimeInterval:(NSTimeInterval)ti invocation:(NSInvocation *)invocation repeats:(BOOL)yesOrNo;
+ (NSTimer *)scheduledTimerWithTimeInterval:(NSTimeInterval)ti invocation:(NSInvocation *)invocation repeats:(BOOL)yesOrNo;
+ (NSTimer *)timerWithTimeInterval:(NSTimeInterval)ti target:(id)aTarget selector:(SEL)aSelector userInfo:(nullable id)userInfo repeats:(BOOL)yesOrNo;
+ (NSTimer *)scheduledTimerWithTimeInterval:(NSTimeInterval)ti target:(id)aTarget selector:(SEL)aSelector userInfo:(nullable id)userInfo repeats:(BOOL)yesOrNo;
invocation方式:
- (void)logicMethod{
NSMethodSignature *signature = [[self class] instanceMethodSignatureForSelector:@selector(Timefired:)];
NSInvocation* invocation = [NSInvocation invocationWithMethodSignature:signature];
invocation.target = self;
invocation.selector = @selector(Timefired:);
NSTimer* timer = [NSTimer scheduledTimerWithTimeInterval:1 invocation:invocation repeats:YES];
}
- (void): Timefired (NSTimer*)timer {
NSLog(@"timer called");
}
selector方式:
- (void)logicMethod {
NSTimer* timer = [NSTimer scheduledTimerWithTimeInterval:60.0 target:self selector:@selector(Timefired:) userInfo:nil repeats:YES];
}
- (void) Timefired:(NSTimer*)timer {
NSLog(@"timer called");
}
2
方法说明
TimerInterval
: 执行之前等待的时间。比如设置成60.0,就代表60秒后执行方法
target
: 需要执行方法的对象。
selector
: 需要执行的方法
repeats
: 是否需要循环
sheduledTimerWith 和 timerWith 和区别。
scheduledTimerWith:
看scheduledTimerWith的函数说明,创建并添加到runloop的default mode。Creates a timer and schedules it on the current run loop in the default mode.
timerWith :
如果调用timerWith函数,就需要自己加入runloop。
You must add the new timer to a run loop, using addTimer:forMode:.
NSTimer *timer = [NSTimer timerWithTimeInterval:1.0 target:self selector:@selector(Timered) userInfo:nil repeats:YES];
[[NSRunLoop mainRunLoop] addTimer:timer forMode:NSDefaultRunLoopMode];
3
释放方法
[timer invalidate];
timer = nil;
注意 :
调用创建方法后,
target
对象的计数器会加1,直到执行完毕,自动减1。如果是循环执行的话,就必须手动关闭,否则会不执行释放方法。
4
特性
1.存在延迟
不管是一次性的还是周期性的timer的实际触发事件的时间,都会与所加入的RunLoop和RunLoop Mode有关,如果此RunLoop正在执行一个连续性的运算,timer就会被延时出发。重复性的timer遇到这种情况,如果延迟超过了一个周期,则会在延时结束后立刻执行,并按照之前指定的周期继续执行。
2.必须加入Runloop
使用scheduledTimerWith的创建方式,会自动把timer加入MainRunloop的NSDefaultRunLoopMode中。如果使用timerWith建定时器,就必须手动加入Runloop:
5
重要属性
1.timeInterval:
时间间隔(秒)
2.valid:
获取定时器是否有效
3.userInfo:
传递信息(可以以字典的形式,将信息传递给要执行的方法)
介绍完了大名鼎鼎的NSTimer的基本使用,大家可能感觉不过如此so eazy,殊不知里面暗坑很多,一不留神就让你程序内存泄漏,下面就让我们来介绍一下。
6
NSTimer问题汇总
问题1:子线程启动定时器问题:
我们都知道iOS是通过runloop作为消息循环机制,主线程默认启动了runloop,可是子线程没有默认的runloop,因此,我们在子线程启动定时器是不生效的。
解决的方式也简单,在子线程启动一下runloop就可以了。
dispatch_async(dispatch_get_global_queue(0, 0), ^{
NSTimer* timer = [NSTimer timerWithTimeInterval:1 target:self selector:@selector(Timered:) userInfo:nil repeats:YES];
[[NSRunLoop currentRunLoop] addTimer:timer forMode:NSRunLoopCommonModes];
[[NSRunLoop currentRunLoop] run];
});
问题2:runloop的mode问题:
我们注意到schedule方式启动的timer是add到runloop的NSDefaultRunLoopMode中,这就会出现其他mode时timer得不到调度的问题。最常见的问题就是在UITrackingRunLoopMode,即UIScrollView滑动过程中定时器失效。
解决方式就是把timer add到runloop的NSRunLoopCommonModes。UITrackingRunLoopMode和kCFRunLoopDefaultMode都被标记为了common模式,所以只需要将timer的模式设置为NSRunLoopCommonModes,就可以在默认模式和追踪模式都能够运行。
问题3:循环引用问题及解决方案:
常见的场景:
有两个控制器ViewControllerA和ViewControllerB,ViewControllerA 跳转到ViewControllerB中,ViewControllerB开启定时器,但是当返回ViewControllerA界面时,定时器依然还在走,控制器也并没有执行dealloc方法销毁掉
为何会出现循环引用的情况呢?究其原因,就是NSTimer的target被强引用了,而通常target就是所在的控制器,他又强引用的timer,造成了循环引用。下面是target参数的说明:
target:The object to which to send the message specified by aSelector when the timer fires. The timer maintains a strong reference to this object until it (the timer) is invalidated.
下面说一下如何解决循环引用的问题。解决的主要方式就是打破timer对target的强引用。
建立一个proxy类,让timer强引用这个实例,这个类中对timer的使用者target采用弱引用的方式,再把需要执行的方法都转发给timer的使用者。
@interface OrderProxyObject : NSProxy
@property (weak, nonatomic) id target;
+ (instancetype)proxyWithTarget:(id)target;
@end
@implementation OrderProxyObject
+ (instancetype)proxyWithTarget:(id)target {
ProxyObject* proxy = [[self class] alloc];
proxy.target = target;
return proxy;
}
- (NSMethodSignature *)methodSignatureForSelector:(SEL)sel{
return [self.target methodSignatureForSelector:sel];
}
- (void)forwardInvocation:(NSInvocation *)invocation{
SEL sel = [invocation selector];
if ([self.target respondsToSelector:sel]) {
[invocation invokeWithTarget:self.target];
}
}
@end
@implementation ProxyTimer
+ (NSTimer *)scheduledTimerWithTimeInterval:(NSTimeInterval)ti target:(id)aTarget selector:(SEL)aSelector userInfo:(nullable id)userInfo repeats:(BOOL)yesOrNo{
NSTimer* timer = [NSTimer scheduledTimerWithTimeInterval:ti target:[ProxyObject proxyWithTarget: aTarget] selector:aSelector userInfo:userInfo repeats:yesOrNo];
return timer;
}
@end
CADisplayLink
CADisplayLink是基于屏幕刷新的周期,所以其一般很准时,每秒刷新60次。其本质也是通过RunLoop,所以不难看出,当RunLoop选择其他模式或被耗时操作过多时,仍旧会造成延迟。
由于其是基于屏幕刷新的,所以也度量单位是每帧,其提供了根据屏幕刷新来设置间隔的frameInterval属性,其决定于屏幕刷新多少帧时调用一次该方法,默认为1,即1/60秒调用一次。
如果我们想要计算出每次调用的时间间隔,可以通过frameInterval * duration求出,后者为屏幕每帧间隔的只读属性。
在日常开发中,适当使用CADisplayLink甚至有优化作用。比如对于需要动态计算进度的进度条,由于起进度反馈主要是为了UI更新,那么当计算进度的频率超过帧数时,就造成了很多无谓的计算。如果将计算进度的方法绑定到CADisplayLink上来调用,则只在每次屏幕刷新时计算进度,优化了性能。MBProcessHUB则是利用了这一特性。
1
创建方法
self.displayLink =[CADisplayLink displayLinkWithTarget:self selector:@selector(handleDisplayLink:)];
[self.displayLink addToRunLoop:[NSRunLoop currentRunLoop] forMode:NSDefau
2
停止方法
[self.displayLink invalidate];
self.displayLink = nil;
当把
CADisplayLink
对象
add
到
runloop
中后,
selector
就能被周期性调用,类似于重复的
NSTimer
被启动了;执行
invalidate
操作时,
CADisplayLink
对象就会从
runloop
中移除,
selector
调用也随即停止,类似于
NSTimer
的
invalidate
方法。
3
特性
1.屏幕刷新时调用
CADisplayLink
是一个能让我们以和屏幕刷新率同步的频率将特定的内容画到屏幕上的定时器类。
CADisplayLink
以特定模式注册到
runloop
后,每当屏幕显示内容刷新结束的时候,
runloop
就会向
CADisplayLink
指定的
target
发送一次指定的
selector
消息,
CADisplayLink
类对应的
selector
就会被调用一次。所以通常情况下,按照iOS设备屏幕的刷新率
60次/秒。
2.延迟
iOS
设备的屏幕刷新频率是固定的,
CADisplayLink
在正常情况下会在每次刷新结束都被调用,精确度相当高。但如果调用的方法比较耗时,超过了屏幕刷新周期,就会导致跳过若干次回调调用机会。
如果CPU过于繁忙,无法保证屏幕
60次/秒
的刷新率,就会导致跳过若干次调用回调方法的机会,跳过次数取决
CPU
的忙碌程度。
4
重要属性
1.frameInterval
NSInteger
类型的值,用来设置间隔多少帧调用一次
selector
方法,默认值是1,即每帧都调用一次。
2.duration
readOnly
的
CFTimeInterval
值,表示两次屏幕刷新之间的时间间隔。需要注意的是,该属性在
target
的
selector
被首次调用以后才会被赋值。
selector
的调用间隔时间计算方式是:
调用间隔时间 = duration × frameInterval
。
dispatch_source
GCD定时器是dispatch_source_t类型的变量,其可以实现更加精准的定时效果。
1
创建方法
//需要将dispatch_source_t timer设置为成员变量,不然会立即释放
@property(nonatomic, strong) dispatch_source_t timer;
//定时器开始执行的延时时间
NSTimeInterval delayTime =3.0f;
//定时器间隔时间
NSTimeInterval timeInterval =3.0f;
//创建子线程队列
dispatch_queue_t queue =dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT,0);
//使用之前创建的队列来创建计时器
_timer =dispatch_source_create(DISPATCH_SOURCE_TYPE_TIMER,0,0, queue);
//设置延时执行时间,delayTime为要延时的秒数
dispatch_time_t startDelayTime =dispatch_time(DISPATCH_TIME_NOW,(int64_t)(delayTime * NSEC_PER_SEC));
//设置计时器
dispatch_source_set_timer(_timer, startDelayTime, timeInterval * NSEC_PER_SEC,0.1* NSEC_PER_SEC);
dispatch_source_set_event_handler(_timer,^{
//执行事件
});
// 启动计时器
dispatch_resume(_timer);
2
停止方法
dispatch_source_cancel(_timer);
3
特性
默认是重复执行的,可以在事件响应回调中通过
dispatch_source_cancel
方法来设置为只执行一次,如下代码:
dispatch_source_set_event_handler(_timer,^{
//执行事件
dispatch_source_cancel(_timer);
});
4
重要属性
1、start
计时器起始时间,可以通过
`dispatch_time`
创建,如果使用
`DISPATCH_TIME_NOW`
,则创建后立即执行
2、
interval
计时器间隔时间,可以通过
`timeInterval * NSEC_PER_SEC`
来设置,其中,
`timeInterval`
为对应的秒数
3、 leeway
“这个参数告诉系统我们需要计时器触发的精准程度。所有的计时器都不会保证
100%
精准,这个参数用来告诉系统你希望系统保证精准的努力程度。如果你希望一个计时器没五秒触发一次,并且越准越好,那么你传递
0
为参数。另外,如果是一个周期性任务,比如检查email,那么你会希望每十分钟检查一次,但是不用那么精准。所以你可以传入
60
,告诉系统
60
秒的误差是可接受的。这样有什么意义呢?简单来说,就是降低资源消耗。如果系统可以让cpu休息足够长的时间,并在每次醒来的时候执行一个任务集合,而不是不断的醒来睡去以执行任务,那么系统会更高效。如果传入一个比较大的leeway给你的计时器,意味着你允许系统拖延你的计时器来将计时器任务与其他任务联合起来一起执行。
通过观察代码,我们可以发现GCD定时器实际上是使用了dispatch源(dispatch source),dispatch源监听系统内核对象并处理。dispatch类似生产者消费者模式,通过监听系统内核对象,在生产者生产数据后自动通知相应的dispatch队列执行,后者充当消费者。通过系统级调用,更加精准。同时可以使用子线程,解决定时间跑在主线程上卡UI问题
需要将dispatch_source_t timer设置为成员变量,不然会立即释放。
定时器不准时的问题及解决
通过上文的叙述,我们大致了解了定时器不准时的原因,总结一下主要是:
-
当前RunLoop过于繁忙
-
RunLoop模式与定时器所在模式不同
上面解释了GCD更加准时的原因,所以解决方案也不难得出:
-
避免过多耗时操作并发
-
采用GCD定时器
-
创建新线程并开启RunLoop,将定时器加入其中(适度使用)
-
将定时器添加到NSRunLoopCommonModes(使用不当会阻塞UI响应)
其中后两者在使用前应确保合理使用,否则会产生负面影响。
参考资料:
iOS开发的三种定时器
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK