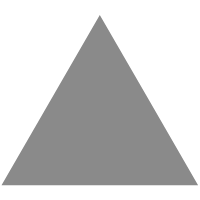
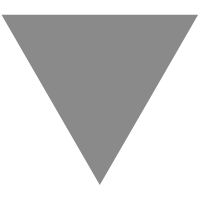
Build an Awesome Developer Portfolio Website Using React
source link: https://levelup.gitconnected.com/build-an-awesome-developer-portfolio-website-using-react-667abd7bab4d?source=friends_link&%3Bsk=128b34f902f9363ef9f6f18125e58b06&%3Bgi=61e18b3f80fd
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Use Create React App and the gitconnected.com portfolio API to build your personal website

Dec 10 ·7min read
In this article, I’ll walk you through building your portfolio website using React with Create React App and deploying the finished project to GitHub Pages.
Using the gitconnected.com portfolio API , we can remove one of the biggest pains of a portfolio website — maintaining the data so it’s always up to date. We’ll fetch the data inside our React app using hooks so your portfolio is always in sync, without ever needing to make code updates or re-deploying the project.
A portfolio is essential for every developer. It gives you the opportunity to display your projects and show everyone the programming languages and libraries you’re passionate about using.
If you want to see the full working code or get stuck at any point, you can find the project on GitHub .
Getting Started
Navigate to your project directory and initialize the application:
npx create-react-app portfolio
We’ll be using React Router to create different views, Styled Components for CSS-in-JS, and Carbon components as our UI framework. Carbon uses Sass for its styles, so we’ll need to handle that in our build process.
yarn add react-router-dom styled-components carbon-components carbon-components-react @carbon/icons-react @carbon/themes node-sass
Fetching Your Data
We’ll start by fetching the data for our portfolio since we only need one request and can use the response for the entire app. Using your GitHub/gitconnected username, we’ll make a request to the portfolio API using React hooks and fetch
. The API can be accessed by making a GET request to the following URL:
https://gitconnected.com/v1/portfolio/<username>
To see an example user, visit — https://gitconnected.com/v1/portfolio/richard-hendricks-demo
Inside src/App.js
, we use the useEffect
hook to make the request only when the component mounts by using an empty array []
for the “watch” parameter. We store the result with the useState
hook and will pass it to our portfolio components which we’ll build in the upcoming sections.
Layout Components
Each page in our app will have the same general structure. There will be a sidebar that has our navigation for the different areas of our portfolio. There will be a top section that will show your general information, and then we will have a dynamic section below this that will change when the page changes.
Shared Styles
Our pages will have some common styles that we’ll want to share, so let’s create a src/styles.js
file and add our reusable styled components first.
Header Component
Our header component displays your general details at the top of every page. It includes your basic information — for me, I used my avatar as well as my name, username, job title, location, years of experience, my headline, and my blog.
Create a src/components/UserHeader
folder and inside it create an index.js
file, a styles.js
file, and a UserHeader.js
file. Inside index.js
we simply import and export the component:
We add our CSS to style and position the content in the header component. In styles.js
:
The user
prop which we fetched as the data at the beginning of the article will be used to populate the content of this component. The user.basics
section will contain all the details about you. Your UserHeader.js
component will contain the following content:
We use the useLocation
hook from React Router to determine if we are on the home page. For a better mobile experience, we will only show the header component on the home page instead of on every page.
Sidebar Component
The sidebar is how visitors will navigate to different parts of your portfolio. First create a src/components/Sidebar
folder and inside it an index.js
file. Inside this, we will import and export the Sidebar
component which we will build next.
To make our page mobile responsive, we’ll need to hide the Sidebar
for mobile device screen widths. Create a styles.js
file and add the following code:
Now we can build out the Sidebar
component in the Sidebar.js
file. We will use the <Link>
component for SPA navigation links, and the useLocation
hook which will let us determine which path is active. We create an array with the routes’ names and paths, and we map
through those to build out the links. Your Sidebar
will be the following:
MobileNav Component
For mobile devices, we’ll instead have buttons at the top of the page to navigate to the different sections of your portfolio.
Create a src/components/MobileNav
folder and inside it an index.js
file. Inside this file, we will import and export the MobileNav
component which we will build next.
Add your style.js
file inside the MobileNav
component folder.
Finally, create your MobileNav.js
component file which is simply 4 navigation buttons at the top of the page.
Layout Component
The Layout
component will be the shell for all of the pages. It will contain the UserHeader
, Sidebar
, and the MobileNav
component and then wrap the children
specific to each section of the portfolio.
Create a src/components/Layout
folder and inside it index.js
, styles.js
, and Layout.js
files. In the index.js
file, we will import and export the Layout.js
component as we have been doing.
In the styles.js
file, make our structure mobile responsive.
Our Layout.js
component will set our Sidebar
and MobileNav
components so that we have navigation on every page. It also adds the UserHeader
to the top of every page, and we pass our user
prop to it. We use the children
for the dynamic page content which will be the components passed from the parent page components.
Pages
We will use React Router to access different views for the different parts of our portfolio (about me, projects, work, and education). Each page will be supplied the user
prop from the data we received from the gitconnected.com portfolio API. This will provide all the content we need to build each page of our portfolio
Create a src/pages
folder and add an index.js
file. This file will declare the routes for each portfolio section.
Then in the pages
folder, we make top-level folders for each route — Me
, Projects
, Work
, and Education
. Inside each folder, create an index.js
, styles.js
, and <PageComponentName>.js
. As we’ve done previously, import and export the component file in each of the individual index.js
files (I will no longer show this step in the code below for brevity).
About Me Page
The Me
page will contain additional general details about you — your bio, skills, and social media links. The additional styles needed for this page are simple. In styles.js
add:
The Me.js
will use the user.basics.summary
and user.basics.profiles
, as well as the user.skills
from the user
prop.
Projects Page
Displaying your projects is one of the most important things you do as a developer. It shows the types of problems you’re passionate about solving and the languages/frameworks you have experience with. First add your styles.js
:
Then in our Projects.js
file, we map
through user.projects
and display the project name, summary, and the languages/libraries used to build it:
Work Page
This section will allow you to display your jobs and relevant work experience. In the styles.js
file, add the following code:
Then in Work.js
, we map through all the user.work
items and display the details of the job:
Education Page
This section will display your education. In the styles.js file, add the following code:
Then in Education.js
, we map through all the user.education
items:
Global Styles for Dark Mode
We will use a dark mode theme from Carbon which is supplied through their Sass files.
Rename the src/index.css
file to src/index.scss
. To make sure our project uses Carbon CSS globally, we will import their stylesheet in this file. We also add some additional styles to support dark mode and mobile responsiveness. Replace the content of index.scss
with the following:
Then in your src/index.js
, change your style import to use the new filename import './index.scss'
. This is all we need to use Carbon — the rest of our styling will be done through Styled Components we previously built.
Deploy to GitHubPages
Following these steps, you should have a fully-built portfolio website! The only thing left to do is deploy it. I’ll use GitHub Pages to deploy our static portfolio site for free, but another good/easy option is using Netlify.
Commit all your code and push it to GitHub. I named my repo portfolio-create-react-app
. Follow this guide if you need help with creating the repo and uploading the code.
1) Add "homepage": "http://<username>.github.io/<repo-name>"
to your package.json
. Replace <username>
and <repo-name>
with the values that you used — my values would be treyhuffine
and portfolio-create-react-app
.
2) Install gh-pages
as a dev dependency:
yarn add -D gh-pages
3) Add 2 scripts to your package.json
:
"predeploy": "npm run build", "deploy": "gh-pages -d build"

4) Finally, run yarn deploy
and visit the URL that you specified in the homepage.
Conclusion
We built a high-quality and dynamic developer portfolio using React, Styled Components, and Carbon design. We used a dark mode theme and created different pages using React Router to navigate between our different portfolio sections.
With the gitconnected.com portfolio API, we were able to dynamically load our portfolio details. This enables us to make updates without needing to change any code or re-deploy the website. We simply make changes to our Gitconnected profiles and see them propagate to our portfolio and resume.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK