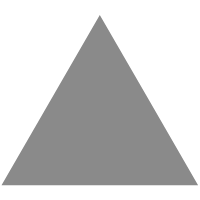
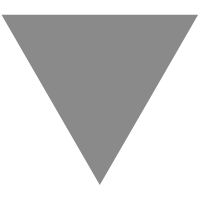
Optional chaining helps to avoid "undefined is not a function" excepti...
source link: https://www.stefanjudis.com/today-i-learned/optional-chaining-helps-to-avoid-undefined-is-not-a-function-exceptions/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
I was reading
the MDN docs for optional chaining
and came across a fact I didn't know about it.
Before we dive in, let's have a look at the new optional chaining
feature very quickly.
This new JavaScript language addition allows developers to access optional values that are nested deeply inside of an object in a safer way.
The process of accessing a deeply nested object property can be very tedious. It could be that the object does not have the structure you expect or that it doesn't define the values you're looking for. To avoid thrown exceptions developers had to check every single property for its existence before accessing the deeply nested property.
The new ?.
syntax helps developers to access properties in a safe manner even if the object structure is different than expected. Let's have a look at an example:
// object coming from an API const someObject = { foo: { bar: { baz: 'someValue' } } }; // old way to access foo.bar.baz // -> check all properties to avoid throwing an exception if (someObject.foo && someObject.foo.bar && someObject.foo.bar.baz) { console.log(someObject.foo.bar.baz); } else { console.log('noValue'); } // new way to access foo.bar.baz console.log(someObject?.foo?.bar?.baz || 'noValue'); // :point_up_2: logs 'someValue' because someObject.foo.bar.baz is defined console.log(someObject?.foo?.bar?.doesNotExist || 'noValue'); // :point_up_2: logs 'noValue' because someObject.foo.bar.baz is not defined console.log(someObject?.doesNotExist?.foo?.bar || 'noValue'); // :point_up_2: logs 'noValue' because someObject.doesNotExist is not defined // it does not throw an exception for accessing `foo` of `doesNotExist`
The optional chaining
feature proposal
is currently on stage 3 of the ECMAscript proposal process. Chrome already implements it behind the feature flag "Experimental JavaScript".
What I didn't know about was that this proposal also includes a mechanism to execute object methods that may or may not be defined.
const someObject = { foo() { return 'someValue'; } }; // execute functions with `?.()` to not throw an exception // in case it is not defined console.log(someObject.foo?.() || 'notDefined'); // :point_up_2: logs 'someValue' because `someObject.foo?.()` returns 'someValue' console.log(someObject.bar?.() || 'notDefined'); // 'notDefined' // :point_up_2: logs 'notDefined' because `someObject.foo?.()` returns undefined // instead of throwing an exception
In my opinion, optional chaining and its optional function execution is a very welcome language addition, which will help to avoid a few (clearly not all of them) undefined is not a function
exceptions.
I can't wait for it to be supported across browsers. If you want to use it today, babel has you covered .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK