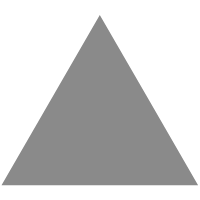
21
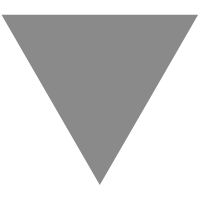
golang cli命令行框架使用
source link: https://studygolang.com/articles/24508
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
简介
cli提供了简单快速的命令行功能的开发。在应用中通过命令设定参数和配置是基本需求。Cli可以帮助快速构建命令行功能。
安装
go get github.com/urfave/cli
简单示例
package main import ( "fmt" "os" "github.com/urfave/cli" ) func main() { app := cli.NewApp() app.Name = "sysconfig" app.Usage = "Setting basic configuration" app.Version = "0.0.1" app.Action = func(c *cli.Context) error { fmt.Println("Prepare applying basic configuration") return nil } app.Run(os.Args) }
编译后执行测试
./cli -h NAME: sysconfig - Setting basic configuration USAGE: cli [global options] command [command options] [arguments...] VERSION: 0.0.1 COMMANDS: help, h Shows a list of commands or help for one command GLOBAL OPTIONS: --help, -h show help --version, -v print the version
可以看到Action的部分没有执行
命令行不带-h 或 -v
./cli Prepare applying basic configuration
可以看到Action的部分被执行
指定要设定的参数使用cli.flags进行参数设定
在应用中要指定MySQL地址或者debug用的port可以使用
cli --mysqlurl 192.168.111.11 --debugport 6666
或者
cli -url 192.168.111.11 -dp 6666
示例
package main import ( "fmt" "os" "github.com/urfave/cli" ) func main() { var mysqlUrl string var debugPort uint app := cli.NewApp() app.Name = "sysconfig" app.Usage = "Setting basic configuration" app.Version = "0.0.1" app.Flags = []cli.Flag{ cli.StringFlag{ Name: "mysqlurl, url", Usage: "specify mysql address", Value: "182.122.11.11", Destination: &mysqlUrl, }, cli.UintFlag{ Name: "debugport, dp", Usage: "specify debug port", Value: 9666, Destination: &debugPort, }, } app.Action = func(c *cli.Context) error { fmt.Println("Prepare applying basic configuration") fmt.Println("MySQL Url:", mysqlUrl) fmt.Println("Debug port:", debugPort) return nil } app.Run(os.Args) }
编译后执行
./cli --mysqlurl 192.168.128.122 --debugport 76554 Prepare applying basic configuration MySQL Url: 192.168.128.122 Debug port: 76554
可以看到mysqlUrl和debugPort已经被赋值为在命令行输入的参数
使用command
例如要设定debug的等级,mysql中查询userid对应的用户信息可以用如下格式的输入
./cli debug grade 2 ./cli mysql query 12345
示例代码如下
package main import ( "fmt" "os" "github.com/urfave/cli" ) func main() { app := cli.NewApp() app.Name = "sysconfig" app.Usage = "Setting basic configuration" app.Version = "0.0.1" app.Commands = []cli.Command{ { Name: "debug", Aliases: []string{"d"}, Usage: "debug settings", Subcommands: []cli.Command{ { Name: "grade", Usage: "set debug grade", Action: func(c *cli.Context) error { fmt.Println("Set debug grade to ", c.Args().First()) return nil }, }, }, }, { Name: "mysql", Aliases: []string{"q"}, Usage: "mysql operations", Subcommands: []cli.Command{ { Name: "query", Usage: "query userid", Action: func(c *cli.Context) error { fmt.Println("query userid=", c.Args().First()) return nil }, }, }, }, } app.Run(os.Args) }
编译后执行
./cli d grade 2 Set debug grade to 2 ./cli q query 3415 query userid= 3415
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK