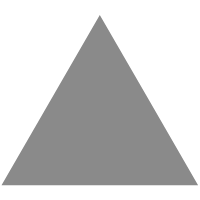
42
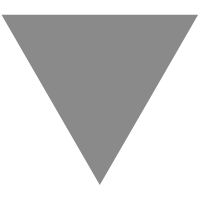
Golang设计模式(工厂方法模式)
source link: https://www.tuicool.com/articles/Zj6Nbqr
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
工厂方法模式类图,

image.png
abstract_factory_test.go
// abstract_factory_test package abstract_factory import ( "testing" ) func TestMotorbikeFactory(t *testing.T) { motobikeF, err := GetVehicleFactory(MotobikeFactoryType) if err != nil { t.Fatal("Create motorbike vehicle factory failed") } vehicle, err := motobikeF.GetVehicle(SportMotorbikeType) if err != nil { t.Fatal("Create sports motor bike failed") } sportVehicle, ok := vehicle.(Motorbike) if !ok { t.Fatal("vehicle is not a motorbike") } t.Logf("Motorbike type is %d", sportVehicle.GetType()) _, err = motobikeF.GetVehicle(3) if err == nil { t.Fatalf("There is no motor bike type is %d", 3) } }
car_factory.go
// car_factory package abstract_factory import ( "errors" "fmt" ) const ( LuxuryCarType = 1 FamiliarCarType = 2 ) type CarFactory struct{} func (c *CarFactory) GetVehicle(v int) (Vehicle, error) { switch v { case LuxuryCarType: return new(LuxuryCar), nil case FamiliarCarType: return new(FamiliarCar), nil default: return nil, errors.New(fmt.Sprintf("Car of type %v not recognized", v)) } }
motorbike_factory.go
// motorbike_factory package abstract_factory import ( "errors" "fmt" ) const ( SportMotorbikeType = 1 CruiseMotorbikeType = 2 ) type MotobikeFactory struct{} func (m *MotobikeFactory) GetVehicle(v int) (Vehicle, error) { switch v { case SportMotorbikeType: return new(SportMotorbike), nil case CruiseMotorbikeType: return new(CruiseMotorbike), nil default: return nil, errors.New(fmt.Sprintf("type of motorbike %v not recognized", v)) } }
vehicle.go
// vehicle package abstract_factory type Vehicle interface { GetWheels() int GetSeats() int }
car.go
// car package abstract_factory type Car interface { GetDoors() int }
motorbike.go
// motorbike package abstract_factory type Motorbike interface { GetType() int }
luxury_car.go
// luxury_car package abstract_factory type LuxuryCar struct{} func (l *LuxuryCar) GetDoors() int { return 4 } func (l *LuxuryCar) GetWheels() int { return 4 } func (l *LuxuryCar) GetSeats() int { return 5 }
familiar_car.go
// familiar_car package abstract_factory type FamiliarCar struct{} func (f *FamiliarCar) GetDoors() int { return 5 } func (f *FamiliarCar) GetWheels() int { return 4 } func (f *FamiliarCar) GetSeats() int { return 5 }
sport_motorbike.go
// sport_motorbike package abstract_factory type SportMotorbike struct{} func (s *SportMotorbike) GetType() int { return SportMotorbikeType } func (s *SportMotorbike) GetWheels() int { return 2 } func (s *SportMotorbike) GetSeats() int { return 2 }
cruise_motorbike.go
// cruise_motorbike package abstract_factory type CruiseMotorbike struct{} func (c *CruiseMotorbike) GetType() int { return CruiseMotorbikeType } func (c *CruiseMotorbike) GetWheels() int { return 2 } func (c *CruiseMotorbike) GetSeats() int { return 2 }
程序输出如下,单元测试不太充分,不想写了。覆盖率才到25%。

image.png
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK