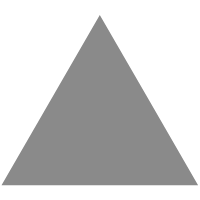
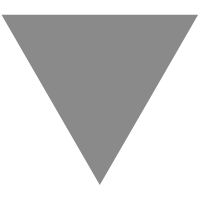
GitHub - Rocketseat/unform: ReactJS form library to create uncontrolled form str...
source link: https://github.com/Rocketseat/unform
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
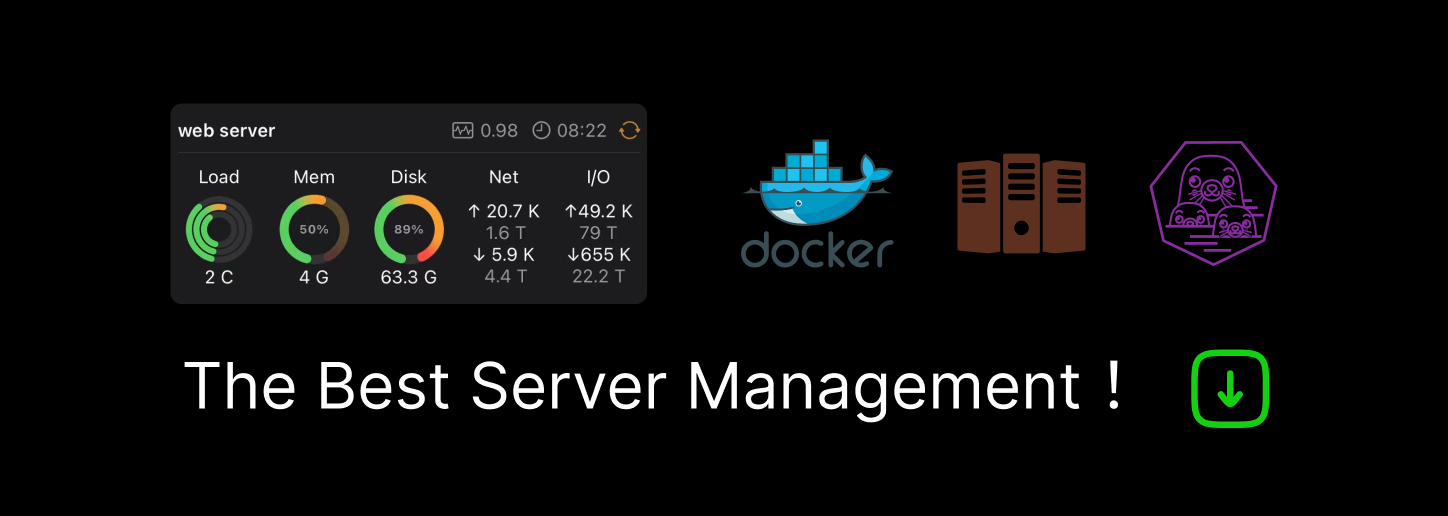
README.md
Overview
Unform is a performance focused library that helps you creating beautiful forms in React with the power of uncontrolled components performance and React Hooks.
Main advantages
- Beautiful syntax;
- React Hooks ?;
- Performance focused;
- Use of uncontrolled components;
- Integration with pickers, dropdowns and other libraries;
Why not Formik, Redux Form or another library?
Formik/Redux Form has a really great syntax while it has a really poor support to uncontrolled components and deep nested data structures. With unform it's easy to create forms with complex relationships without losing performance.
Roadmap
- Native checkbox/radio support;
- Styled components support;
- React Native support (should we?);
- Better docs;
Installation
Just add unform to your project:
yarn add @rocketseat/unform
Table of contents
Guides
Basics
import React from "react"; import { Form, Input } from "@rocketseat/unform"; function App() { function handleSubmit(data) { console.log(data); /** * { * email: 'email@example.com', * password: "123456" * } */ }; return ( <Form onSubmit={handleSubmit}> <Input name="email" /> <Input name="password" type="password" /> <button type="submit">Sign in</button> </Form> ); }
Nested fields
import React from "react"; import { Form, Input, Scope } from "@rocketseat/unform"; function App() { function handleSubmit(data) { console.log(data); /** * { * name: 'Diego', * address: { street: "Name of street", number: 123 } * } */ }; return ( <Form onSubmit={handleSubmit}> <Input name="name" /> <Scope path="address"> <Input name="street" /> <Input name="number" /> </Scope> <button type="submit">Save</button> </Form> ); }
Initial data
import React from "react"; import { Form, Input, Scope } from "@rocketseat/unform"; function App() { const initialData = { name: 'John Doe', address: { street: 'Sample Avenue', }, } function handleSubmit(data) {}; return ( <Form onSubmit={handleSubmit} initialData={initialData}> <Input name="name" /> <Scope path="address"> <Input name="street" /> <Input name="number" /> </Scope> <button type="submit">Save</button> </Form> ); }
Validation
import React from "react"; import { Form, Input } from "@rocketseat/unform"; import * as Yup from 'yup'; const schema = Yup.object().shape({ email: Yup.string() .email('Custom invalid email message') .required('Custom required message'), password: Yup.string().min(4).required(), }) function App() { function handleSubmit(data) {}; return ( <Form schema={schema} onSubmit={handleSubmit}> <Input name="email" /> <Input name="password" type="password" /> <button type="submit">Save</button> </Form> ); }
Contribute
- Fork it
- Create your feature branch (git checkout -b my-new-feature)
- Commit your changes (git commit -am 'Add some feature')
- Push to the branch (git push origin my-new-feature)
- Create new Pull Request
License
MIT © Rocketseat
Recommend
-
36
The Uncontrolled An Uncontrolled Component is one that stores and maintains its own state internally. A
-
48
When we working with form validation, most of us would be familiar with libraries such as Formik and Redux-form. Both are popular amon...
-
53
Join GitHub today GitHub is home to over 50 million developers working together to host and review code, manage projects, and build software together.
-
45
README.md Expo common issues Esse repositório contém uma série de erros (e suas soluções) que você pode ter com o Expo. Expo...
-
21
readme.md Semana OmniStack 10.0 da Rocketseat 🚀 🚀 Tecnologias usadas Este maravilhoso projeto foi desenvolvido com as seguintes tecn...
-
10
Custom defn macro with clojure.spec - part 1: conform/unform Oct 10, 2016 • Yehonathan Sharvit Parsing With clojure.spec, we can parse functions and macro...
-
17
Files Permalink Latest commit message Commit time
-
10
Tecnologias | Projeto |
-
4
Files Permalink Latest commit message Commit time
-
21
README.md ...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK