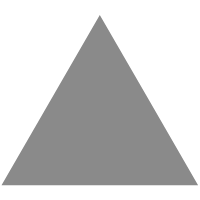
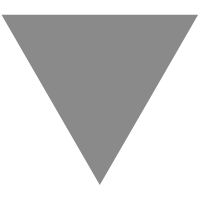
「docker实战篇」python的docker爬虫技术-appium+python实战(18)
source link: https://idig8.com/2019/04/06/dockershizhanpianpythondedockerpachongjishu-appiumpythonshizhan18/?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
上次通过appium进行了,录制脚本的功能,而且还可以进行转换成python,java,js等等语言的,这次实战下,从登陆,到进入某个页面操作获取信息。
源码:https://github.com/limingios/dockerpython.git
流程代码
1.点击跳过导航页面,进入登录页面
2.输入用户名和密码,点击登录
3.进入页面点击同意,点击研迅
4.模拟手势,上移
- 准备工作
- 安装【考研帮】
2.设置-应用-点击【考研帮】-清除数据
- 启动【考研帮】
- 启动直接adb中的uiautomatorviewer.bat 记得看看下载源码包里面的增强版
5.看到uiautomatorviewer 和 夜神模拟器
6.appium启动
- 编写代码
\#!/usr/bin/env python # -*- coding: utf-8 -*- # @Time : 2019/1/22 20:15 # @Author : Aries # @Site : # @File : yankao.py # @Software: PyCharm #pip3 install Appium-Python-Client import time from appium import webdriver from selenium.webdriver.support.ui import WebDriverWait cap = { "platformName": "Android", "platformVersion": "5.1.1", "deviceName": "127.0.0.1:62001", "appPackage": "com.tal.kaoyan", "appActivity": "com.tal.kaoyan.ui.activity.SplashActivity", "noReset": True } driver = webdriver.Remote("http://localhost:4723/wd/hub",cap) def get_size(): x = driver.get_window_size()['width'] y = driver.get_window_size()['height'] return(x,y) #导航点击跳过 try: #是否跳过 if WebDriverWait(driver,3).until(lambda x:x.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.TextView[1]")): driver.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.TextView[1]").click() except: pass try: if WebDriverWait(driver,3).until(lambda x:x.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.ScrollView[1]/android.widget.LinearLayout[1]/android.widget.EditText[1]")): driver.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.ScrollView[1]/android.widget.LinearLayout[1]/android.widget.EditText[1]").send_keys("idig8") driver.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.ScrollView[1]/android.widget.LinearLayout[1]/android.widget.EditText[2]").send_keys("3989441") driver.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.ScrollView[1]/android.widget.LinearLayout[1]/android.widget.Button[1]").click() except: pass #隐私协议 try: #隐私协议 if WebDriverWait(driver,3).until(lambda x:x.find_element_by_xpath("//android.widget.TextView[@resource-id='com.tal.kaoyan:id/tv_agree']")): driver.find_element_by_xpath("//android.widget.TextView[@resource-id='com.tal.kaoyan:id/tv_agree']").click() driver.find_element_by_xpath("//android.support.v7.widget.RecyclerView[@resource-id='com.tal.kaoyan:id/date_fix']/android.widget.RelativeLayout[3]").click() except: pass #点击研讯 if WebDriverWait(driver,3).until(lambda x:x.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.support.v4.view.ViewPager[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[2]/android.widget.LinearLayout[1]/android.widget.LinearLayout[1]/android.widget.LinearLayout[1]/android.support.v4.view.ViewPager[1]/android.widget.FrameLayout[1]/android.widget.RelativeLayout[1]/android.support.v7.widget.RecyclerView[1]/android.widget.LinearLayout[1]/android.support.v7.widget.RecyclerView[1]/android.widget.RelativeLayout[3]/android.widget.LinearLayout[1]/android.widget.ImageView[1]")): driver.find_element_by_xpath("//android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[1]/android.widget.FrameLayout[1]/android.support.v4.view.ViewPager[1]/android.widget.FrameLayout[1]/android.widget.LinearLayout[2]/android.widget.LinearLayout[1]/android.widget.LinearLayout[1]/android.widget.LinearLayout[1]/android.support.v4.view.ViewPager[1]/android.widget.FrameLayout[1]/android.widget.RelativeLayout[1]/android.support.v7.widget.RecyclerView[1]/android.widget.LinearLayout[1]/android.support.v7.widget.RecyclerView[1]/android.widget.RelativeLayout[3]/android.widget.LinearLayout[1]/android.widget.ImageView[1]").click() l = get_size() x1 = int(l[0]*0.5) y1 = int(l[1]*0.75) y2 = int(l[1]*0.25) #滑动操作 while True: driver.swipe(x1,y1,x1,y2) time.sleep(0.5)
注意点:
1.xpath 通过uiautomatorviewer 点击查看
2.python在运行过程中如果进行uiautomatorviewer加载会报错
3.python在使用的过程中需要先引入
from appium import webdriver
from selenium.webdriver.support.ui import WebDriverWait
4.前提是启动的appium:提示:The server is running
5.里面有几个需要注意的套路:
appium服务的远程调用
webdriver.Remote(“http://localhost:4723/wd/hub”,cap)
判断xpath是否存在
if WebDriverWait(driver,3).until(lambda x:x.find_element_by_xpath(“‘路径”)):
输入框复制
driver.find_element_by_xpath(“路径”).send_keys(“填入数据”)
按钮点击
driver.find_element_by_xpath(“路径”).click()
模拟手势上啦,先获取屏幕的宽高,然后通过driver.swipe(x1,y1,x1,y2) 从指定的(x1,y1)坐标到(x2,y2)
def get_size():
x = driver.get_window_size()[‘width’]
y = driver.get_window_size()[‘height’]
return(x,y)
l = get_size()
0.5)
0.75)y2 = int(l[1]*0.25)
driver.swipe(x1,y1,x1,y2)
PS:最后实现了如何从登陆到点击研迅,模拟手指,拉下加载的功能。
>>原创文章,欢迎转载。转载请注明:转载自IT人故事会,谢谢!
>>原文链接地址:上一篇:已是最新文章
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK