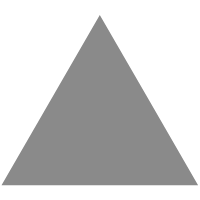
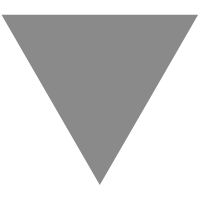
Build a Contact Form with React and PHP
source link: https://www.tuicool.com/articles/hit/ayyAzq7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
There are many ways to create contact forms, from using HTML and jQuery or HTML and Javascript to choosing more modern frameworks like React.
Today we’ll try to do something a little different, in a modern way.
By the end of this post, you’ll have a fully working contact from you can integrate into Wordpress or your php website. I also published the final project in github, so you can download and play around with it.
Prerequisites? not really
For this tutorial you don’t have to be an expert in Javascript, React or PHP. Basic knowledge is enough to get the hang of this tutorial. I also won’t go into basic details like installing React and writing CSS code.
We will only be creating the frontEnd part, but I will show you how to build the backend part as well. For the backend part you need a web server like Apache or Nginx. Only the basic email sending will be done with php.
For Mac users the fastest way to setup a server is to download and install Mamp. I have already installed Mamp with Nginx. You don’t need any database for this because we are just sending emails to your mailbox without saving it in a database.
OK then, let’s get this party started!
Tip: Build faster with React components by sharing them with Bit . Your team can easily share components, sync changes and use them anywhere. Try it out.
Creating the React app
You should have create-react-app installed in your computer. If you don’t have create-react-app, you can install it via npm. Run this in your console.
npm install create-react-app
Let’s navigate to your project folder in console. Mine is:
cd ~/Sites/react-contact-form
To create the React app in the folder run this command:
create-react-app .
This will take a couple of seconds or minutes. Once it is done open the project with your favorite IDE or code editor. Mine is VSCode. Then open up App.js file in the ./src/App.js folder. You will see something like this :

Creating the contact form
Let’s clean up a bit. Delete everything inside the div with class App. Now your render method should looks like this.
Just an empty div.
Let’s create a basic form with two text inputs for the first and second name, one email input for the user email, one textarea for the message and of course the submit button.
Now your render method will look like this:
Adding basic styling to your form
Create React app has already imported a CSS file by default. You can see App.css file in the ./src folder. Open it up and delete everything inside the file. Add this CSS code to the file. I’m not gonna explain what all the CSS does. But it’s pretty basic, just adding some widths and aligning.
Okay. I don’t wanna confuse you too much. We did a bit of coding but have not seen any output yet. So let’s save everything and go back to the terminal. In the project root folder run:
npm start
This will open up the browser with localhost:3000 port. Now you will be able to see our cute little form like this.

Okay, great. Now it’s time for some real React stuff.
Handling the form with React!
Now we have a basic static React form. But it doesn’t do anything yet. We will need an initial state and submit handler function.
Form state
Now we will add a state for our form. We should create a constructor for this:
Then we need our form submit handler method like this:
For now we can log the state to see if we have all the values saved in the state.
Finally, let’s bind the form handler.
Now we have to set our initial states as form input values, and whenever changing our input we will set our state to the current input value.
At this point we change our form like this:
If you try to write something in the form and try to submit it now, you will see your data in the console. Open your console and check if it works. The console output should look like this.

Send an email with PHP
Well. We are almost done with the frontend part. Now we need a server side language to send emails to our mailbox. I choose php this time. Before we start writing the backend part let’s add two more properties to our state. We need to add { error and mailSent }.
The state is ready. Let’s start with php. If you created your react app already in Apache or Nginx www folder, you can go to your project root and create a folder called api/contact inside the contact folder.
Create an index.php file. Our email function will live here. I wanna mention that we are not going to use OOP approach. But if you want a cleaner php code, you can clean up and improve it a little bit.
From the React app we are sending a post request to php api. In our contact form the first name and email should be required. Because in php we are gonna check for those two values. If these two values are empty we are going to throw an error. So keep it in mind.
Let’s add this code to your index.php file.
Basically what we are doing is: We are checking for the first name and email. Then using the php mail() function, we are sending an email to our email account with the contact form data.
Now if you navigate to your api folder http://localhost/react-contact-form/api/contact
…you should see nothing. Because our api is dying, if we don’t send the first name and email as well — it’s looking for a post request.
Sending the data to an API
Our API is ready and now it’s time for finishing up our form. So we need to send our data that has been saved in the state. We can use Javascript fetch for this. But recently I was using another npm package that works very well with React for http requests. Maybe you already know about it ‘Axios’. So we need to install this package first. Go to your React app folder in the console.
And execute:
npm install axios
Great. Now go back to App.js and on the top of the file you have to import Axios.
import axios from 'axios';
After importing Axios we have to create a constant for our api path. Create the constant before the App class.
Then let’s use Axios inside the handleFormSubmit method to send our data to the backend.
Now we should be able to send emails via our contact form. Finally, let’s show a message to the user after they sent the email.
Before the form closing tag </form>, add this.
Now our user is able to see a message after sending the email. All done! You have a cute little contact form with React and php.
Download the code from github :
Final look

Conclusion
Thanks for reading! I hope this article provides some insight into how easy it is to use React and php to create a simple contact form. I encourage you to change or try and improve the React part. Maybe even add a loader and play around with the styling.
If you have any questions or remarks, leave a comment below.
Thanks again for reading! Please star the github repo , drop a :clap: (or two), that would make me very happy and please feel free to comment below!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK