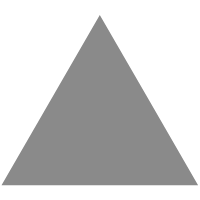
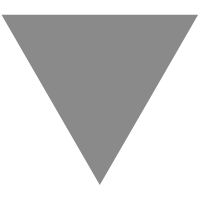
Build Popup Contact Form using jQuery and PHP
source link: https://www.codexworld.com/jquery-popup-contact-form-with-email-using-ajax-php/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Build Popup Contact Form using jQuery and PHP
The Contact Form is a must-have feature for the web application. It helps the site admin to get feedback/suggestions/complaints from the users. Generally, the contact form element is placed inside a web page. But, this placement can be changed as per the UI of the website. The modal window is a very useful element to add a contact form. You can easily integrate the contact form functionality to any web page using a modal window.
The modal window opens a popup element over the current web page without interacting page elements. The popup contact form can be attached to any link or button on the web page. In this tutorial, we will show you how to create a contact form in a popup dialog using jQuery and send an email with form data using Ajax and PHP.
In the example script, we will build a contact form in a popup window and submit the form data without page refresh using jQuery Ajax. On form submission, the form data will be sent to the site admin via email using PHP.
Popup Contact Form
Trigger Button:
The following button (#mbtn
) triggers the modal window.
<button id="mbtn" class="btn btn-primary turned-button">Contact Us</button>
Modal Dialog:
The following HTML creates a modal dialog with the contact form.
<div id="modalDialog" class="modal"> <div class="modal-content animate-top"> <div class="modal-header"> <h5 class="modal-title">Contact Us</h5> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> </div> <form method="post" id="contactFrm"> <div class="modal-body"> <!-- Form submission status --> <div class="response"></div> <!-- Contact form --> <div class="form-group"> <label>Name:</label> <input type="text" name="name" id="name" class="form-control" placeholder="Enter your name" required=""> </div> <div class="form-group"> <label>Email:</label> <input type="email" name="email" id="email" class="form-control" placeholder="Enter your email" required=""> </div> <div class="form-group"> <label>Message:</label> <textarea name="message" id="message" class="form-control" placeholder="Your message here" rows="6"></textarea> </div> </div> <div class="modal-footer"> <!-- Submit button --> <button type="submit" class="btn btn-primary">Submit</button> </div> </form> </div> </div>
Open/Hide Modal Popup:
Include the jQuery library.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
The following code helps to open or close the popup window using jQuery.
- The trigger button (
#mbtn
) open the modal. - The close button (
.close
) hide the modal.
<script> // Get the modal var modal = $('#modalDialog'); // Get the button that opens the modal var btn = $("#mbtn"); // Get the <span> element that closes the modal var span = $(".close"); $(document).ready(function(){ // When the user clicks the button, open the modal btn.on('click', function() { modal.show(); }); // When the user clicks on <span> (x), close the modal span.on('click', function() { modal.hide(); }); }); // When the user clicks anywhere outside of the modal, close it $('body').bind('click', function(e){ if($(e.target).hasClass("modal")){ modal.hide(); } }); </script>
Contact Form Submission
When the submit button is clicked on the dialog window, the form data is submitted to the server-side script via jQuery and Ajax.
$.ajax()
method is used to initialize the Ajax request.- The entire contact form data is sent to the server-side script (
ajax_submit.php
). - Based on the response, the form submission status is displayed on the popup.
<script> $(document).ready(function(){ $('#contactFrm').submit(function(e){ e.preventDefault(); $('.modal-body').css('opacity', '0.5'); $('.btn').prop('disabled', true); $form = $(this); $.ajax({ type: "POST", url: 'ajax_submit.php', data: 'contact_submit=1&'+$form.serialize(), dataType: 'json', success: function(response){ if(response.status == 1){ $('#contactFrm')[0].reset(); $('.response').html('<div class="alert alert-success">'+response.message+'</div>'); }else{ $('.response').html('<div class="alert alert-danger">'+response.message+'</div>'); } $('.modal-body').css('opacity', ''); $('.btn').prop('disabled', false); } }); }); }); </script>
Send Email using PHP
This ajax_submit.php file is called by the Ajax request to send an email with contact form data using PHP.
- Get form data using PHP $_POST method.
- Validate the user’s input to check whether the submitted value is not empty and valid.
- Send HTML email with form inputs using PHP mail() function.
- Return the response in JSON encoded format using json_encode() function.
<?php
// Recipient email
$toEmail = '[email protected]';
$status = 0;
$statusMsg = 'Something went wrong! Please try again after some time.';
if(isset($_POST['contact_submit'])){
// Get the submitted form data
$email = $_POST['email'];
$name = $_POST['name'];
$message = $_POST['message'];
// Check whether submitted data is not empty
if(!empty($email) && !empty($name) && !empty($message)){
if(filter_var($email, FILTER_VALIDATE_EMAIL) === false){
$statusMsg = 'Please enter a valid email.';
}else{
$emailSubject = 'Contact Request Submitted by '.$name;
$htmlContent = '<h2>Contact Request Submitted</h2>
<h4>Name</h4><p>'.$name.'</p>
<h4>Email</h4><p>'.$email.'</p>
<h4>Message</h4><p>'.$message.'</p>';
// Set content-type header for sending HTML email
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
// Additional headers
$headers .= 'From: '.$name.'<'.$email.'>'. "\r\n";
// Send email
$sendEmail = mail($toEmail, $emailSubject, $htmlContent, $headers);
if($sendEmail){
$status = 1;
$statusMsg = 'Thanks! Your contact request has been submitted successfully.';
}else{
$statusMsg = 'Failed! Your contact request submission failed, please try again.';
}
}
}else{
$statusMsg = 'Please fill in all the mandatory fields.';
}
}
$response = array(
'status' => $status,
'message' => $statusMsg
);
echo json_encode($response);
?>
Creating a Simple Contact Form with PHP
Conclusion
This example code helps you to build a contact form popup using jQuery. Not only the contact us form, but you can also use this script for any types of popup form (Login, Registration, Feedback, etc). When the user submits the form, the input data will be sent to the site admin via email. You can easily enhance the functionality of this jQuery popup contact form as per your needs.
Are you want to get implementation help, or modify or enhance the functionality of this script? Submit Paid Service Request
If you have any questions about this script, submit it to our QA community - Ask Question
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK