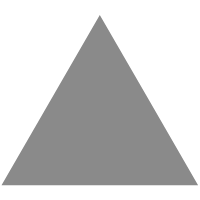
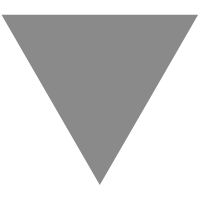
Mule 3.9: Separate Mule Info and Error Logs via Log4J2 Configuration
source link: https://www.tuicool.com/articles/hit/uuQ3emn
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this article, I will explain how to separate the Mule application info and error logs into a separate log file. For instance, we have a Mule application that runs every 10 seconds to read an input file located on the local path /appdata/temp/input/persons.csv.
LOG4J2.XML Configuration
To separate the Mule INFO and ERROR logs, we need to define 2 separate RollingRandomAccessFile tags under Appenders in our log4j2.xml file located in src/main/resources. One for INFO logs and another for ERROR logs . We can set the filename of the log file as well as the log category (INFO, ERROR, WARN, DEBUG) that the file should contain which can be configured using ThresholdFilter Level. Lastly, we need to add the two RollingRandonAccessFile configuration to the loggers tag under AsyncRoot.
<?xml version="1.0" encoding="utf-8"?> <Configuration> <Properties> <Property name="baseDir">${sys:mule.home}${sys:file.separator}logs${sys:file.separator}${sys:hostName}</Property> <Property name="infoFileName">mule-sample-interface-info</Property> <Property name="errorFileName">mule-sample-interface-error</Property> </Properties> <Appenders> <RollingRandomAccessFile name="info_file" fileName="${baseDir}-${infoFileName}.log" filePattern="${baseDir}-${infoFileName}-%d{yyyy-MM-dd}-%i.log"> <PatternLayout pattern="%d [%t] ${sys:hostName} %-5p %c - %m%n" /> <Policies> <SizeBasedTriggeringPolicy size="30 MB" /> <TimeBasedTriggeringPolicy interval="1" modulate="true" /> </Policies> <Filters> <ThresholdFilter level="INFO" /> </Filters> </RollingRandomAccessFile> <RollingRandomAccessFile name="error_file" fileName="${baseDir}-${errorFileName}.log" filePattern="${baseDir}-${errorFileName}-%d{yyyy-MM-dd}-%i.log"> <PatternLayout pattern="%d [%t] ${sys:hostName} %-5p %c - %m%n" /> <Policies> <SizeBasedTriggeringPolicy size="30 MB" /> <TimeBasedTriggeringPolicy interval="1" modulate="true" /> </Policies> <Filters> <ThresholdFilter level="ERROR" /> </Filters> </RollingRandomAccessFile> </Appenders> <Loggers> <!-- Http Logger shows wire traffic on DEBUG --> <AsyncLogger name="org.mule.module.http.internal.HttpMessageLogger" level="WARN"/> <!-- JDBC Logger shows queries and parameters values on DEBUG --> <AsyncLogger name="com.mulesoft.mule.transport.jdbc" level="WARN"/> <!-- CXF is used heavily by Mule for web services --> <AsyncLogger name="org.apache.cxf" level="WARN"/> <!-- Apache Commons tend to make a lot of noise which can clutter the log--> <AsyncLogger name="org.apache" level="WARN"/> <!-- Reduce startup noise --> <AsyncLogger name="org.springframework.beans.factory" level="WARN"/> <!-- Mule classes --> <AsyncLogger name="org.mule" level="INFO"/> <AsyncLogger name="com.mulesoft" level="INFO"/> <!-- Reduce DM verbosity --> <AsyncLogger name="org.jetel" level="WARN"/> <AsyncLogger name="Tracking" level="WARN"/> <AsyncRoot level="INFO"> <AppenderRef ref="info_file" /> <AppenderRef ref="error_file" /> </AsyncRoot> </Loggers> </Configuration>
Mule Application Code
<?xml version="1.0" encoding="UTF-8"?> <mule xmlns:validation="http://www.mulesoft.org/schema/mule/validation" xmlns:scripting="http://www.mulesoft.org/schema/mule/scripting" xmlns:file="http://www.mulesoft.org/schema/mule/file" xmlns="http://www.mulesoft.org/schema/mule/core" xmlns:doc="http://www.mulesoft.org/schema/mule/documentation" xmlns:spring="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.mulesoft.org/schema/mule/scripting http://www.mulesoft.org/schema/mule/scripting/current/mule-scripting.xsd http://www.mulesoft.org/schema/mule/file http://www.mulesoft.org/schema/mule/file/current/mule-file.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-current.xsd http://www.mulesoft.org/schema/mule/core http://www.mulesoft.org/schema/mule/core/current/mule.xsd http://www.mulesoft.org/schema/mule/validation http://www.mulesoft.org/schema/mule/validation/current/mule-validation.xsd"> <file:connector name="FILE_INBOUND_CONNECTOR_CONFIG" autoDelete="false" streaming="true" validateConnections="true" doc:name="File" fileAge="30000"/> <validation:config name="Validation_Configuration" doc:name="Validation Configuration"/> <flow name="mule-sample-interfaceFlow"> <poll doc:name="Run every 10 seconds"> <fixed-frequency-scheduler frequency="10000"/> <logger message="#['\n'+message.rootId] - Start Of The Process" level="INFO" doc:name="Logger Start"/> </poll> <message-properties-transformer scope="invocation" doc:name="Message Properties Set Path and Filename Variable"> <add-message-property key="targetPath" value="/appdata/temp/input/"/> <add-message-property key="targetFilename" value="persons"/> </message-properties-transformer> <scripting:transformer doc:name="Groovy Read File"> <scripting:script engine="Groovy"><![CDATA[def filenameWildcard = flowVars.targetFilename + "*" def endpointBuilder = muleContext.endpointFactory.getEndpointBuilder('file://'+flowVars.targetPath+'?connector=FILE_INBOUND_CONNECTOR_CONFIG') endpointBuilder.addMessageProcessor(new org.mule.routing.MessageFilter(new org.mule.transport.file.filters.FilenameWildcardFilter(filenameWildcard))) def inboundEndpoint = endpointBuilder.buildInboundEndpoint() inboundEndpoint.request(60000)]]></scripting:script> </scripting:transformer> <validation:is-not-null config-ref="Validation_Configuration" message="No Input File on the source path." value="#[payload]" doc:name="Validation"/> <byte-array-to-string-transformer doc:name="Byte Array to String"/> <logger message="#['\nContent:\n'+payload]" level="INFO" doc:name="Logger File Content"/> <logger message="#['\n'+message.rootId] - End Of The Process" level="INFO" doc:name="Logger End"/> </flow> </mule>
Test Result
The Mule Application was deployed and ran as expected.
2 log files were generated after the deployment of the Mule application.
The mule-sample-interface-info only contains info logs of the Mule application.
The mule-sample-interface-error has no error logs since there's no error occurring during the Mule application execution.
Removing the input file should cause an error and that will be logged in the mule-sample-interface-error file.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK