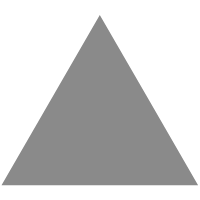
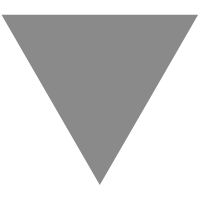
Chapter 5.3 : Working with Functions in Kotlin (Android)
source link: https://www.tuicool.com/articles/hit/reaY3aY
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
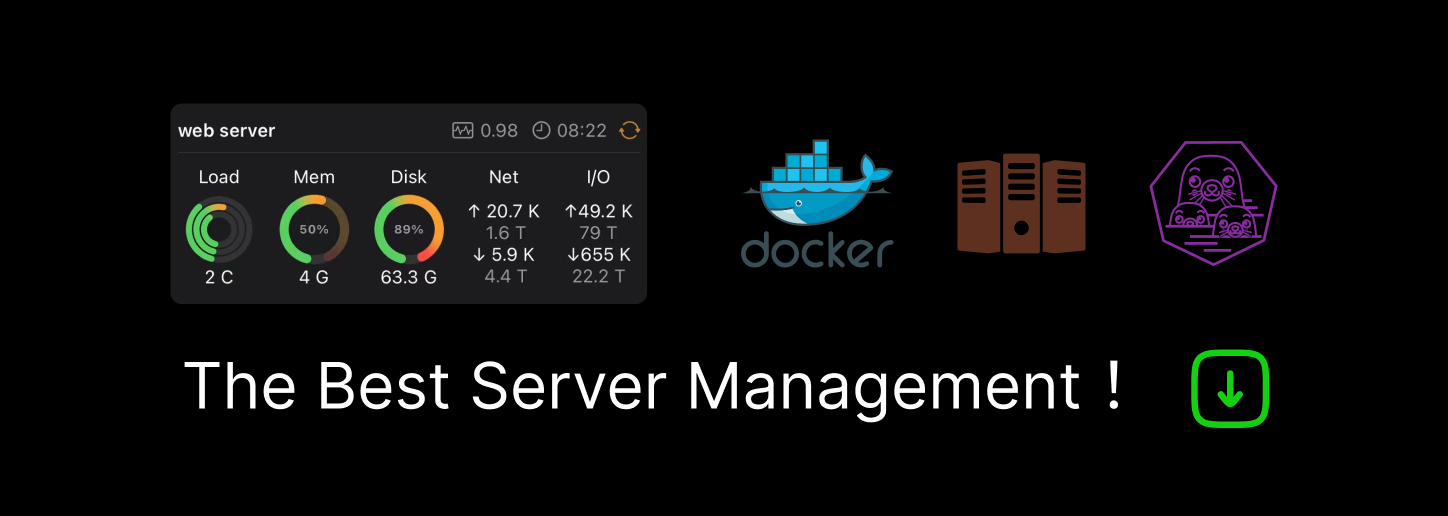
In this article, you will learn about functions scope like local and as member functions.
Before moving to current topic, I explained Kotlin from beginning and you can find full list of previous tutorial at Kotlin page.
In previous articles, we have learned about functions. In first article, we learned for return value and default argument in parameters. In second article, we learned about variable argument and infix notation in function.
Local function
Kotlin supports local functions. Local function means a function inside a function. It is also known as nested function.
fun calculateTotal(value : Int) : Int { if(value < 0){ throw IllegalArgumentException("Value must be greater than zero") } fun divideMe(value : Int) : Int { return value / 2 } var result = divideMe(value) return result } >>> println("Result : " + calculateTotal(-1)) >>> println("Result : " + calculateTotal(4)) //java.lang.IllegalArgumentException: Value must be greater than zero //Result : 2
This kind of functionality is very much helpful when you want to divide your function’s functionality into deeper level.
Member function
In any Object Oriented language, you can create class and its properties. Those properties also include function to perform. Such function known as member function.
data class Square(var value : Int) { fun calculateLength() : Int { return this.value * this.value } } >>> var square = Square(4) >>> println("Result : " + square.calculateLength()) //Result : 16
Summary
In this article, you learned how to work with local/nested and member functions.
Thanks for reading. Please ask any doubts in the comment. Don’t forget to share this article.

Views: 0
Recommend
-
127
More about functions in KotlinPublished by Gurleen Sethi on October 23, 2017
-
122
Firebase Functions in KotlinSome time ago I got interested in blending together two trendy technologies — Firebase Functions and Kotlin. After some research and tinkering I posted a
-
55
In this article, you will going to learn about functions and its usage in Kotlin. You’ll also learn function without return value, with return value and default argument. But before moving to current topic, I ex...
-
14
Chapter 6.1 : Working with Collections in Kotlin (Android) Chintan Rathod–Kotlin–July 16, 2018July 15, 2018...
-
9
SEO YouTube adds video chapter previews, auto-translates captions in new search functions The auto-translation...
-
20
Chapter 9. PostGIS Special Functions IndexChapter 9. PostGIS Special Functions IndexChapter 9. PostGIS Special Functions Index9.1. PostGIS Aggregate Functions...
-
18
The React Mega-Tutorial, Chapter 3: Working with Components
-
6
Reflection on Working effectively with legacy code --- chapter 20 to 23 Chapter 20: This Class Is Too Big and I Don’t Want It to Get Any Bigger Chapter 21: I’m Changing the Same Code All Over the Place Chapter 22: I...
-
8
Reflection on Working effectively with legacy code --- chapter 11 to 19At ch6-10 we know why adding t...
-
9
Reflection on Working effectively with legacy code --- chapter 6 to 10At part I we basically underst...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK