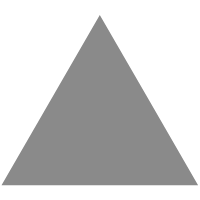
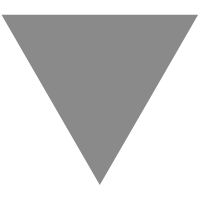
What's new in ES2023? 👀
source link: https://dev.to/jasmin/what-is-new-in-es2023-4bcm
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Last year I wrote an article about the new features of ES2022 this year let's check out the new features coming in as part of ES2023.
Features of ES2023
1. Find array from the last
This function will allow us to find element from the last to first of the array based on a condition.
const array = [{a: 1, b: 1}, {a: 2, b: 2}, {a: 3, b: 3}, {a: 4, b: 4}]
console.log(array.findLast(n => n)); //result -> {a: 4,b: 4 }
console.log(array.findLast(n => n.a * 5 === 20)); // result -> {a:4,b:4} as the condition is true so it returns the last element.
console.log(array.findLast(n => n.a * 5 === 21)); //result -> undefined as the condition is false so return undefined instead of {a:4,b:4}.
console.log(array.findLastIndex(n => n.a * 5 === 21)); // result -> -1 as the condition is not justified for returning the last element.
console.log(array.findLastIndex(n => n.a * 5 === 20)); // result -> 3 which is the index of the last element as the condition is true.
2. Hashbang Grammer
This feature would enable us to use Hashbang / Shebang in some CLI.
Shebang is represented by #! and is a special line at the beginning of the script that tells the operating system which interpreter to use when executing the script.
#!/usr/bin/env node
// in the Script Goal
'use strict';
console.log(2*3);
#!/usr/bin/env node
// in the Module Goal
export {};
console.log(2*2);
#!/usr/bin/env node
this line would invoke a Node.js source file directly, as an executable in its own.
We would not need this line (#!/usr/bin/env node)
to invoke a file explicitly via the node interpreter, e.g., node ./file
3. Symbols as WeakMap keys
This allows using unique Symbols as keys. Currently WeakMaps are limited to only allow objects as keys. Objects are used as keys for WeakMaps because they share the same identity aspect.
Symbol is the only primitive type in ECMAScript that allows unique values therefor using Symbol as key instead of creating a new object with WeakMap.
const weak = new WeakMap();
const key = Symbol('my ref');
const someObject = { a:1 };
weak.set(key, someObject);
console.log(weak.get(key));
More use cases related to ShadowRealms and Record & Tuples using Symbols as WeakMaps
4. Change Array by Copy
This provides additional methods on Array.prototype
to make changes to array by returning new copy of it with the change instead of updating the original array.
New Array.prototype
introduced functions are:
Array.prototype.toReversed()
Array.prototype.toSorted(compareFn)
Array.prototype.toSpliced(start, deleteCount, ...items)
-
Array.prototype.with(index, value)
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
/* toReversed */
const reversed = numbers.toReversed();
console.log("reversed", reversed); // "reversed", [9, 8, 7, 6, 5, 4, 3, 2, 1]
console.log("original", numbers); // "original", [1, 2, 3, 4, 5, 6, 7, 8, 9]
/* toSorted */
const sortedArr = numbers.toSorted();
console.log("sorted", sortedArr); // "sorted", [1, 2, 3, 4, 5, 6, 7, 8, 9]
console.log("original", numbers); // "original", [1, 2, 3, 4, 5, 6, 7, 8, 9]
/* with */
const replaceWith = numbers.with(1, 100);
console.log("with", replaceWith); // "with", [1, 100, 3, 4, 5, 6, 7, 8, 9]
console.log("original", numbers); // "original", [1, 2, 3, 4, 5, 6, 7, 8, 9]
/* toSpliced */
const splicedArr = numbers.toSpliced(0, 4);
console.log("toSpliced", splicedArr); // "toSpliced", [5, 6, 7, 8, 9]
console.log("original", numbers); // "original", [1, 2, 3, 4, 5, 6, 7, 8, 9]
These are some awesome set of features coming up with ES2023 which I am really looking forward to try them out personally in my day to day coding.
Happy Coding! 👩💻
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK