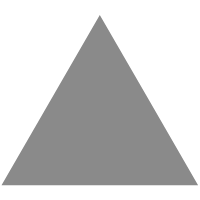
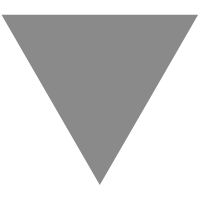
ASP.NET Core 中的框架级依赖注入 | Oopsguy
source link: http://oopsguy.com/2017/10/23/dependency-injection-with-asp-net-core/?
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
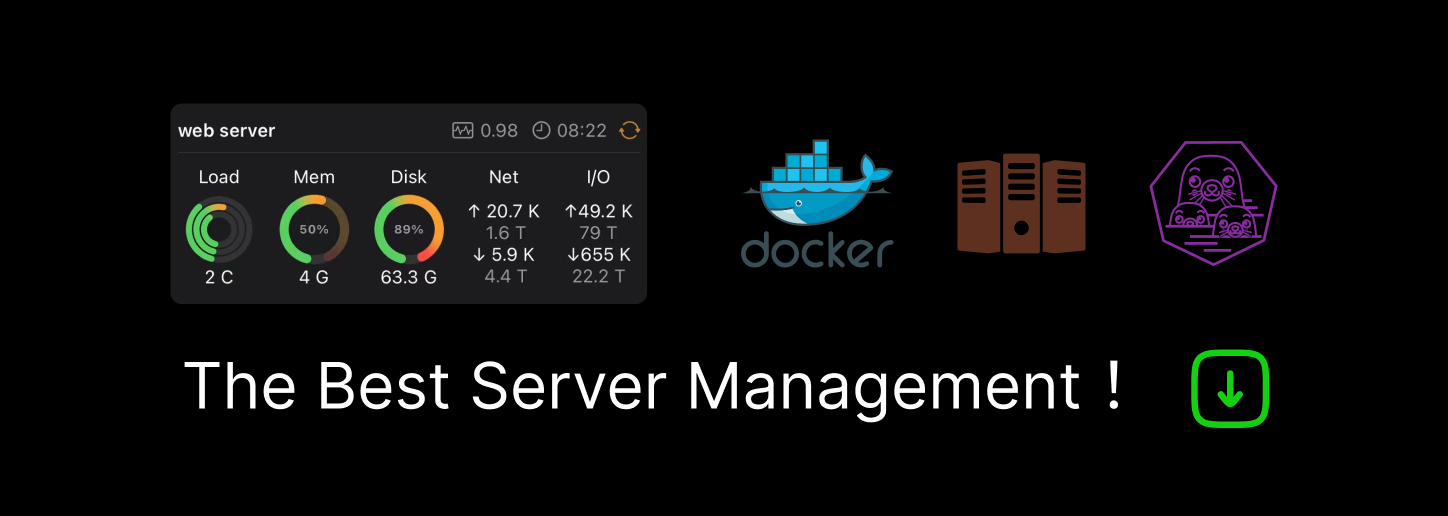
https://tech.io/playgrounds/5040/framework-level-dependency-injection-with-asp-net-core
作者: Gunnar Peipman
译者:http://oopsguy.com
1、ASP.NET Core 中的依赖注入
本文将介绍 ASP.NET Core 框架级依赖注入是如何工作的。其简单但功能强大,足以完成大部分依赖注入工作。框架级依赖注入支持以下 scope:
- Singleton — 总是返回相同的实例,单例
- Transient — 每次都返回新的实例
- Scoped — 在当前(request)范围内返回相同的实例
假设我们有两个要通过依赖注入来进行工作的工件:
- PageContext — 自定义请求上下文
- Settings — 全局应用程序设置
这两个都是非常简单的类。PageContext 类为布局页面提供当前页面标题的标题标签。
public class Settings
{
public string SiteName;
public string ConnectionString;
}
public class PageContext
{
private readonly Settings _settings;
public PageContext(Settings settings)
{
_settings = settings;
}
public string PageTitle;
public string FullTitle
{
get
{
var title = (PageTitle ?? "").Trim();
if(!string.IsNullOrWhiteSpace(title) &&
!string.IsNullOrWhiteSpace(_settings.SiteName))
{
title += " | ";
}
title += _settings.SiteName.Trim();
return title;
}
}
}
2、注册依赖
在 UI 构建块中使用这些类之前,你需要在应用程序启动时注册这些类。这些工作可以在 Startup 类的 ConfigureServices() 方法中完成。
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
var settings = new Settings();
settings.SiteName = Configuration["SiteName"];
services.AddSingleton(settings);
services.AddScoped<PageContext>();
}
现在可以将这些类注入到支持依赖注入的控制器和其他 UI 组件中。
3、向控制器注入实例
我们通过 Home 控制器中的 PageContext 类分配页面标题。
public class HomeController : Controller
{
private readonly PageContext _pageContext;
public HomeController(PageContext pageContext)
{
_pageContext = pageContext;
}
public IActionResult Index()
{
_pageContext.PageTitle = "";
return View();
}
public IActionResult About()
{
_pageContext.PageTitle = "About";
return View();
}
public IActionResult Error()
{
_pageContext.PageTitle = "Error";
return View();
}
}
这种分配页面标题的方式不错,因为我们不必使用 ViewData,这样更容易被支持多语言应用程序所支持。
4、向视图注入实例
现在控制器的 action 中分配了页面标题,是时候在布局页面中使用标题了。我在页面的内容区域添加了标题,所以在 tech.io 环境中也很容易看到。为了能在布局页面中使用到 PageContext,我使用了视图注入(以下代码片段中的第一行)。
@inject PageContext pageContext
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>@pageContext.FullTitle</title>
<environment names="Development">
<link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.css" />
<link rel="stylesheet" href="~/css/site.css" />
</environment>
<environment names="Staging,Production">
<link rel="stylesheet" href="https://ajax.aspnetcdn.com/ajax/bootstrap/3.3.7/css/bootstrap.min.css"
asp-fallback-href="~/lib/bootstrap/dist/css/bootstrap.min.css"
asp-fallback-test-class="sr-only" asp-fallback-test-property="position" asp-fallback-test-value="absolute" />
<link rel="stylesheet" href="~/css/site.min.css" asp-append-version="true" />
</environment>
</head>
...
</html>
5、参考材料
Recommend
-
22
写在前面 上一篇大家已经粗略接触了解到.NET Core中间件的使用:ASP .Net Core 中间件的使用(一):搭建静态文件服务器/访问指定文件,
-
8
IoC主要体现了这样一种设计思想:通过将一组通用流程的控制权从应用转移到框架之中以实现对流程的复用,并按照“好莱坞法则”实现应用程序的代码与框架之间的交互。我们可以采用若干设计模式以不同的方式实现IoC,比如我们在前面介绍的模板方法、工厂方法和抽象工...
-
9
ASP.NET Core在启动以及后续针对每个请求的处理过程中的各个环节都需要相应的组件提供相应的服务,为了方便对这些组件进行定制,ASP.NET通过定义接口的方式对它们进行了“标准化”,我们将这些标准化的组件称为服务,ASP.NET在内部专门维护了一个DI容器来提供所需...
-
5
正如我们在《依赖注入:控制反转》提到过的,很多人将IoC理解为一种“面向对象的设计模式”,实际上IoC不仅与面向对象没有必然的联系,它自身甚至算不上是一种设计模式。一般来讲...
-
8
在采用了依赖注入的应用中,我们总是直接利用DI容器直接获取所需的服务实例,换句话说,DI容器起到了一个服务提供者的角色,它能够根据我们提供的服务描述信息提供一个可用的服务对象。ASP.NET Core中的DI容器体现为一个实现了IServiceProvider接口的对象。
-
11
IoC主要体现了这样一种设计思想:通过将一组通用流程的控制从应用转移到框架之中以实现对流程的复用,同时采用“好莱坞原则”是应用程序以被动的方式实现对流程的定制。我们可以采用若干设计模式以不同的方式实现IoC,比如我们在
-
8
【ASP.NET Core】选项类的依赖注入 咱们继续上一个话题...
-
8
1. Ioc 与 DI Ioc 和DI 这两个词大家都应该比较熟悉,这两者已经在各种开发语言各种框架中普遍使用,成为框架中的一种基本设施了。 Ioc 是控制反转, Inversion of Control 的缩写,DI 是依赖注入,Inject Dependency 的缩写。...
-
11
.NET Core 依赖注入的基本用法 话接上篇,这一章介绍 .NET Core 框架自带的轻量级 Ioc 容器下服务使用的一些知识点,大家可以先看看上一篇文章 [ASP.NET Core - 依赖注入(一)] 2.3 服务...
-
6
4. ASP.NET Core默认服务 之前讲了中间件,实际上一个中间件要正常进行工作,通常需要许多的服务配合进行,而中间件中的服务自然也是通过 Ioc 容器进行注册和注入的。前面也讲到,按照约定中间件的封装一般会提供一个 User{Midd...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK