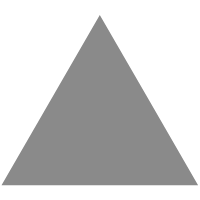
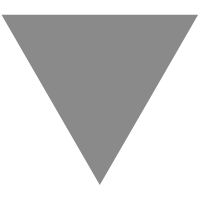
给我十分钟!我就能带你入门Python!超级详细的基础教程!
source link: http://www.10tiao.com/html/385/201806/2651697818/1.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python
>>> help(5)
Help on int object:
(etc etc)
>>> dir(5)
['__abs__', '__add__', ...]
>>> abs.__doc__
'abs(number) -> number
Return the absolute value of the argument.'
语法
Python中没有强制的语句终止字符,且代码块是通过缩进来指示的。缩进表示一个代码块的开始,逆缩进则表示一个代码块的结束。声明以冒号(:)字符结束,并且开启一个缩进级别。单行注释以井号字符(#)开头,多行注释则以多行字符串的形式出现。赋值(事实上是将对象绑定到名字)通过等号(“=”)实现,双等号(“==”)用于相等判断,”+=”和”-=”用于增加/减少运算(由符号右边的值确定增加/减少的值)。这适用于许多数据类型,包括字符串。你也可以在一行上使用多个变量。例如:
数据类型
Python具有列表(list)、元组(tuple)和字典(dictionaries)三种基本的数据结构,而集合(sets)则包含在集合库中(但从Python2.5版本开始正式成为Python内建类型)。列表的特点跟一维数组类似(当然你也可以创建类似多维数组的“列表的列表”),字典则是具有关联关系的数组(通常也叫做哈希表),而元组则是不可变的一维数组(Python中“数组”可以包含任何类型的元素,这样你就可以使用混合元素,例如整数、字符串或是嵌套包含列表、字典或元组)。数组中第一个元素索引值(下标)为0,使用负数索引值能够从后向前访问数组元素,-1表示最后一个元素。数组元素还能指向函数。来看下面的用法:
字符串
Python中的字符串使用单引号(‘)或是双引号(“)来进行标示,并且你还能够在通过某一种标示的字符串中使用另外一种标示符(例如 “He said ‘hello’.”)。而多行字符串可以通过三个连续的单引号(”’)或是双引号(“””)来进行标示。Python可以通过u”This is a unicode string”这样的语法使用Unicode字符串。如果想通过变量来填充字符串,那么可以使用取模运算符(%)和一个元组。使用方式是在目标字符串中从左至右使用%s来指代变量的位置,或者使用字典来代替,示例如下:
流程控制
Python中可以使用if、for和while来实现流程控制。Python中并没有select,取而代之使用if来实现。使用for来枚举列表中的元素。如果希望生成一个由数字组成的列表,则可以使用range(<number>)函数。以下是这些声明的语法示例:
Python
函数
函数通过“def”关键字进行声明。可选参数以集合的方式出现在函数声明中并紧跟着必选参数,可选参数可以在函数声明中被赋予一个默认值。已命名的参数需要赋值。函数可以返回一个元组(使用元组拆包可以有效返回多个值)。Lambda函数是由一个单独的语句组成的特殊函数,参数通过引用进行传递,但对于不可变类型(例如元组,整数,字符串等)则不能够被改变。这是因为只传递了该变量的内存地址,并且只有丢弃了旧的对象后,变量才能绑定一个对象,所以不可变类型是被替换而不是改变(译者注:虽然Python传递的参数形式本质上是引用传递,但是会产生值传递的效果)。例如:
类
Python支持有限的多继承形式。私有变量和方法可以通过添加至少两个前导下划线和最多尾随一个下划线的形式进行声明(如“__spam”,这只是惯例,而不是Python的强制要求)。当然,我们也可以给类的实例取任意名称。例如:
Python
class MyClass(object):
common = 10
def __init__(self):
self.myvariable = 3
def myfunction(self, arg1, arg2):
return self.myvariable
# This is the class instantiation
>>> classinstance = MyClass()
>>> classinstance.myfunction(1, 2)
3
# This variable is shared by all classes.
>>> classinstance2 = MyClass()
>>> classinstance.common
10
>>> classinstance2.common
10
# Note how we use the class name
# instead of the instance.
>>> MyClass.common = 30
>>> classinstance.common
30
>>> classinstance2.common
30
# This will not update the variable on the class,
# instead it will bind a new object to the old
# variable name.
>>> classinstance.common = 10
>>> classinstance.common
10
>>> classinstance2.common
30
>>> MyClass.common = 50
# This has not changed, because "common" is
# now an instance variable.
>>> classinstance.common
10
>>> classinstance2.common
50
# This class inherits from MyClass. The example
# class above inherits from "object", which makes
# it what's called a "new-style class".
# Multiple inheritance is declared as:
# class OtherClass(MyClass1, MyClass2, MyClassN)
class OtherClass(MyClass):
# The "self" argument is passed automatically
# and refers to the class instance, so you can set
# instance variables as above, but from inside the class.
def __init__(self, arg1):
self.myvariable = 3
print arg1
>>> classinstance = OtherClass("hello")
hello
>>> classinstance.myfunction(1, 2)
3
# This class doesn't have a .test member, but
# we can add one to the instance anyway. Note
# that this will only be a member of classinstance.
>>> classinstance.test = 10
>>> classinstance.test
10
导入
外部库可以使用 import [libname] 关键字来导入。同时,你还可以用 from [libname] import [funcname] 来导入所需要的函数。例如:
Python
import random
from time import clock
randomint = random.randint(1, 100)
>>> print randomint
64
文件I / O
Python针对文件的处理有很多内建的函数库可以调用。例如,这里演示了如何序列化文件(使用pickle库将数据结构转换为字符串):
其它杂项
数值判断可以链接使用,例如 1<a<3 能够判断变量 a 是否在1和3之间。
可以使用 del 删除变量或删除数组中的元素。
列表推导式(List Comprehension)提供了一个创建和操作列表的有力工具。列表推导式由一个表达式以及紧跟着这个表达式的for语句构成,for语句还可以跟0个或多个if或for语句,来看下面的例子:
全局变量在函数之外声明,并且可以不需要任何特殊的声明即能读取,但如果你想要修改全局变量的值,就必须在函数开始之处用global关键字进行声明,否则Python会将此变量按照新的局部变量处理(请注意,如果不注意很容易被坑)。例如:
小结
本教程并未涵盖Python语言的全部内容(甚至连一小部分都称不上)。Python有非常多的库以及很多的功能特点需要学习,所以要想学好Python你必须在此教程之外通过其它方式,例如阅读Dive into Python。我希望这个教程能给你一个很好的入门指导。如果你觉得本文还有什么地方值得改进或添加,或是你希望能够了解Python的哪方面内容,请留言。
欢迎关注我的博客或者公众号:https://home.cnblogs.com/u/Python1234/ Python学习交流
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK