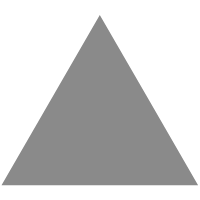
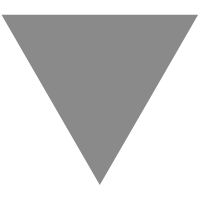
GitHub - fragkp/laravel-route-breadcrumb: Add breadcrumbs to your routes
source link: https://github.com/fragkp/laravel-route-breadcrumb
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Add breadcrumbs to your routes
This package tries to give a simple solution for breadcrumbs. Add breadcrumbs direct to your routes and display them in your views.
Installation
You can install the package via composer:
composer require fragkp/laravel-route-breadcrumb
Laravel 5.5 uses Package Auto-Discovery, so doesn't require you to manually add the ServiceProvider.
If you don't use Auto-Discovery, add the ServiceProvider to the providers array in config/app.php
Fragkp\LaravelRouteBreadcrumb\BreadcrumbServiceProvider::class,
If you want also use the facade to access the main breadcrumb class, add this line to your facades array in config/app.php:
'Breadcrumb' => Fragkp\LaravelRouteBreadcrumb\Facades\Breadcrumb::class,
Usage
Defining breadcrumbs
Basic
To add a breadcrumb title to your route, call the breadcrumb
method and pass your title.
Route::get('/')->breadcrumb('Your custom title');
Index
On some websites, you wish to have always an index inside your breadcrumbs. Use the breadcrumbIndex
method.
This method should only be used once.
Note:
breadcrumbIndex
sets also the breadcrumb title for this route.
Route::get('/')->breadcrumbIndex('Start'); Route::get('/foo')->breadcrumb('Your custom title');
Inside groups
The breadcrumb
method will also work inside route groups.
Route::get('/')->breadcrumbIndex('Start'); Route::prefix('/foo')->group(function () { Route::get('/bar')->breadcrumb('Your custom title'); });
Group index
Also, it is possible to specify a group index title by calling breadcrumbGroup
.
This method should only be used once inside a group.
Note:
breadcrumbGroup
sets also the breadcrumb title for this route.
Route::get('/')->breadcrumbIndex('Start'); Route::prefix('/foo')->group(function () { Route::get('/')->breadcrumbGroup('Foo group index'); Route::get('/bar')->breadcrumb('Your custom title'); });
Custom title resolver
If you want to customize your breadcrumb title, you could pass a closure or callback to all breadcrumb methods.
Route::get('/')->breadcrumb(function () { return 'Your custom title'; });
You could also pass a fully qualified class name. This will invoke your class.
Route::get('/')->breadcrumb(YourCustomTitleResolver::class); class YourCustomTitleResolver { public function __invoke() { return 'Your custom title'; } }
Route parameters
All route parameters will be passed to your resolver. Route model binding is also supported.
Route::get('/{foo}')->breadcrumb(YourCustomTitleResolver::class); class YourCustomTitleResolver { public function __invoke(Foo $foo) { return "Title: {$foo->title}"; } }
Accessing breadcrumb
Links
The links
method will return a Collection
of BreadcrumbLink
.
Note: The array is indexed by the uri.
app(Breadcrumb::class)->links(); // or use here the facade
Example result:
Illuminate\Support\Collection {#266 #items: array:2 [ "/" => Fragkp\LaravelRouteBreadcrumb\BreadcrumbLink {#41 +uri: "/" +title: "Start" } "foo" => Fragkp\LaravelRouteBreadcrumb\BreadcrumbLink {#262 +uri: "foo" +title: "Your custom title" } ] }
Index
The index
method will return a single instance of BreadcrumbLink
. If you haven't defined any index, null is returned.
app(Breadcrumb::class)->index(); // or use here the facade
Example result:
Fragkp\LaravelRouteBreadcrumb\BreadcrumbLink {#36 +uri: "/" +title: "Start" }
Current
The current
method will return a single instance of BreadcrumbLink
. If no route is provided (e.g. on errors), null is returned.
app(Breadcrumb::class)->current(); // or use here the facade
Example result:
Fragkp\LaravelRouteBreadcrumb\BreadcrumbLink {#36 +uri: "/" +title: "Your custom title" }
View example
A good way to access the breadcrumb inside your views is to bound it via a View Composer.
For more information about View Composers, have a look at the Laravel docs.
// app/Providers/AppServiceProvider.php class AppServiceProvider extends ServiceProvider { public function boot() { View::composer('your-view', function ($view) { $view->with('breadcrumb', app(Breadcrumb::class)->links()); }); } }
// resources/views/breadcrumb.blade.php <ul> @foreach ($breadcrumb as $link) <li> <a href="{{ url($link->uri) }}">{{ $link->title }}</a> </li> @endforeach </ul>
Testing
./vendor/bin/phpunit
License
MIT License (MIT). Please see License File for more information.
Recommend
-
22
README.md Laravel Breadcrumbs
-
0
Some time ago, we introduced breadcrumbs for Ruby and JavaScript. We're...
-
7
Ziggy – Use your Laravel routes in JavaScript Ziggy provides a JavaScript route() helper function that works like Laravel's, making it easy to use your Laravel named routes in JavaScript. Ziggy supports all versio...
-
6
Pretty Routes for Laravel Display your Laravel routes in the console, but make it pretty. Installation You can instal...
-
6
Laravel Auto Routes _ _____ _ /\ | | | __ \ | | / \ _ _| |_ ___ ______| |__) |___ _ _| |_ ___ ___ / /\ \|...
-
6
Use PHP 8 attributes to register routes in a Laravel app This package provides annotations to automatically register routes. Here's a quick example: use Spatie\RouteAttributes\Attributes\Get; class MyController { #...
-
3
Harry Nguyen Posted on Nov 27...
-
2
Test Your Laravel Routing Skills This repository is a test for you: fill in routes/web.php and routes/api.php which are left intentionally empty. In both of those files, you will find comments, descri...
-
235
Image credit: freepik.comA Qui...
-
6
M-x breadcrumb-mode
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK