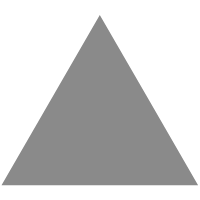
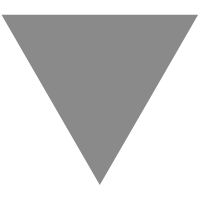
GitHub - ai/size-limit: Prevent JS libraries bloat. If you accidentally add a ma...
source link: https://github.com/ai/size-limit
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Size Limit 
Size Limit is a tool to prevent JavaScript libraries bloat. With it, you know exactly for how many kilobytes your JS library increases the user bundle.
You can add Size Limit to your continuous integration service (such as Travis CI) and set the limit. If you accidentally add a massive dependency, Size Limit will throw an error.
Size Limit could tell you not only library size. With --why
argument it can
tell you why your library has this size and show real cost of all your
internal dependencies.
Who Uses Size Limit
- MobX
- Material-UI
- Autoprefixer
- PostCSS reduced 25% of the size.
- Browserslist reduced 25% of the size.
- EmojiMart reduced 20% of the size
- nanoid reduced 33% of the size.
- Logux reduced 90% of the size.
How It Works
You can find more examples in Size Limit: Make the Web lighter article.
To be really specific, Size Limit creates an empty webpack project in memory. Then, it adds your library as a dependency to the project and calculates the real cost of your libraries, including all dependencies, webpack’s polyfills for process, etc.
Usage
First, install size-limit
:
$ npm install --save-dev size-limit
Add size-limit
section to package.json
and size
script:
+ "size-limit": [ + { + "path": "index.js" + } + ], "scripts": { + "size": "size-limit", "test": "jest && eslint ." }
The path
option:
- For an open source library, specify compiled sources, which will be published
to npm (usually the same value as the
main
field in thepackage.json
); - For an application, specify a bundle file and use
webpack: false
(see the Applications section).
Here’s how you can get the size for your current project:
$ npm run size Package size: 8.46 KB With all dependencies, minified and gzipped
If your project size starts to look bloated, run Webpack Bundle Analyzer for analysis:
$ npm run size -- --why
Now, let’s set the limit. Determine the current size of your library,
add just a little bit (a kilobyte, maybe) and use that as a limit in
your package.json
:
"size-limit": [
{
+ "limit": "9 KB",
"path": "index.js"
}
],
Add the size
script to your test suite:
"scripts": { "size": "size-limit", - "test": "jest && eslint ." + "test": "jest && eslint . && npm run size" }
If you don’t have a continuous integration service running, don’t forget to add one — start with Travis CI.
Config
Size Limits supports 3 ways to define config.
-
size-limit
section topackage.json
:"size-limit": [ { "path": "index.js", "limit": "9 KB" } ]
-
or separated
.size-limit
config file:[ { path: "index.js", limit: "9 KB" } ]
-
or more flexible
.size-limit.js
config file:module.exports = [ { path: "index.js", limit: "9 KB" } ]
Each section in config could have options:
- path: relative paths to files. The only mandatory option.
It could be a path
"index.js"
, a pattern"dist/app-*.js"
or an array["index.js", "dist/app-*.js"]
. - limit: size limit for files from
path
option. It should be a string with a number and unit (100 B
,10 KB
, etc). - name: the name of this section. It will be useful only if you have multiple sections.
- webpack: with
false
will disable webpack. - gzip: with
false
will disable gzip compression. - config: a path to custom webpack config.
Applications
Webpack inside Size Limit is very useful for small open source library. But if you want to use Size Limit for application, not open source library, you could already have webpack to make bundle.
In this case you can disable internal webpack:
"size-limit": [
{
"limit": "300 KB",
+ "webpack": false,
"path": "public/app-*.js"
}
],
JavaScript API
const getSize = require('size-limit') const index = path.join(__dirname, 'index.js') const extra = path.join(__dirname, 'extra.js') getSize([index, extra]).then(size => { if (size.gzip > 1 * 1024 * 1024) { console.error('Project is now larger than 1MB!') } })
Comparison with bundlesize
Main difference between Size Limit and bundlesize
, that Size Limit uses
webpack to build bundle. It has more accurate result and can show you
what and why causes the bloat.
- Size Limit has the
--why
mode to run Webpack Bundle Analyzer — this way, you can see what went wrong in a nice graphical representation. - Instead of bundlesize, Size Limit prevents the most popular source of libraries bloat — unexpected huge dependency.
- Also Size Limit prevents increasing library size because of wrong
process
orpath
usage, when webpack will add big unnecessary polyfill. - Size Limit runs only on first CI job, so it is more respectful to CI resources.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK